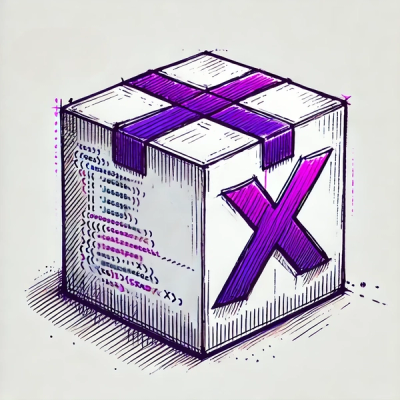
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
filerobot-uploader
Advanced tools
[DEPRECATED] The Filerobot Uploader is a multi-function Uploader that will make uploads super easy in your web sites and apps. It is fast to integrate allows end users to upload media, files and any assets via Filerobot's reverse CDN. Files are stored int
Repository includes React version and JS wrapper for standalone usage
Docs • Demo • CodeSandbox
The Filerobot Uploader is a multi-function Uploader that will make uploads super easy on your web and mobile applications. With few lines of code, you will get a state of the art Uploader and enable your users to upload media, files and any assets via Filerobot's reverse CDN. Files are stored into scalable and flexible Cloud storage, optimized and delivered over CDN to your end users rocket fast. Features include inline image editing, auto-tagging, auto-cropping and many more.
Use latest CDNized plugin version
<script src="https://cdn.scaleflex.it/plugins/filerobot-uploader/2.15.20/filerobot-uploader.min.js"></script>
To use the Filerobot Uploader in your application, you need to first create a free Filerobot account here. Once registered, you will obtain your container name and filerobotUploadKey to configure the Uploader in your application:
<script>
let config = {
container: 'example',
filerobotUploadKey: '0cbe9ccc4f164bf8be26bd801d53b132'
};
let onUpload = (files) => {
console.log('files: ', files);
alert('Files uploaded successfully! check the console to see the uploaded files');
};
let uploader = FilerobotUploader.init(config, onUpload);
uploader.open();
</script>
window.FilerobotUploader.init(config: {}, uploadHandler: callback)
: functionInitialization of Filerobot Uploader plugin.
uploadHandler(files: file[], info: {})
: functionFunction to handle uploaded files.
example response:
[{
"uuid": "a955877f-c79d-5b7c-8f70-18e7a2950000",
"name": "Screen Shot 2019-02-28 at 10.03.43 PM.png",
"type": "image/png",
"size": 102069,
"sha1": "a91c1e7aea6aed05a22b42bc3f46326820e4275e",
"meta": { "img_type": "PNG", "img_h": 320, "img_w": 816 },
"url_permalink": "https://scaleflex-tests-v5a.api.airstore.io/v1/get/_/a955877f-c79d-5b7c-8f70-18e7a2950000/Screen Shot 2019-02-28 at 10.03.43 PM.png",
"url_public": "https://scaleflex-tests-v5a.airstore.io/demo_filerobot_en/Screen Shot 2019-02-28 at 10.03.43 PM.png",
"properties": {
"description": "",
"tags": ["Text", "Blue", "Font", "White", "Logo", "Azure", "Line", "Product", "Aqua", "Brand"],
"lang": "en",
"search": " Text Blue Font White Logo Azure Line Product Aqua Brand"
},
"overwrite": false
}]
info: {} - additional information on upload
info.stage: string (upload|edit|select) - stage on which uploadHandler was triggered
window.FilerobotUploader.open(tab : string, options: {})
: functionOpen uploader modal.
tab: string (optional, default: 'UPLOAD') - allow to choose the initial tab (should be one of enabled modules)
options: {} (optional) - options for tabs
options.file: {} (optional) - open Uploader to Tag or Edit specified file
options.closeOnEdit: bool (optional) - close Uploader on complete Tagging or Editing, default false
options.closeOnSave: bool (optional) - close Uploader on click "save" button in Tagging tab, default true
window.FilerobotUploader.close()
: functionClose uploader modal.
window.FilerobotUploader.unmount()
: functionDestroy uploader
$ npm install --save filerobot-uploader
We provide easy way to integrate image uploader in your applications
import React, { Component } from 'react';
import { render } from 'react-dom';
import FilerobotUploader from 'filerobot-uploader';
const config = {
modules: ['UPLOAD', 'MY_GALLERY', 'ICONS_GALLERY', 'IMAGES_GALLERY', 'TAGGING', 'IMAGE_EDITOR'],
uploadParams: { dir:"/demo_filerobot_en" },
filerobotUploadKey: '7cc1f659309c480cbc8a608dc6ba5f03',
container: 'scaleflex-tests-v5a'
}
class App extends Component {
constructor() {
super();
this.state = {
isShow: false
}
}
render() {
return (
<div>
<h1>React Example</h1>
<button onClick={() => { this.setState({ isShow: true }); }}>Click</button>
<FilerobotUploader
opened={this.state.isShow}
config={config}
onClose={() => { this.setState({ isShow: false }); }}
onUpload={(img) => { console.log(img); }}
/>
</div>
)
}
}
render(<App/>, document.getElementById('app'));
opened
: bool (required)default: false
Trigger to display the uploader widget.
initialTab
: string (optional)default: 'UPLOAD'
Allow to choose the initial tab. Should be one of enabled modules.
config
: object (required)Uploader config.
options
: object (optional)onClose()
: function (required)Close uploader widget.
onUpload(files: file[], info: {})
: function (required)Function to handle uploaded files.
example response:
[{
"uuid": "a955877f-c79d-5b7c-8f70-18e7a2950000",
"name": "Screen Shot 2019-02-28 at 10.03.43 PM.png",
"type": "image/png",
"size": 102069,
"sha1": "a91c1e7aea6aed05a22b42bc3f46326820e4275e",
"meta": { "img_type": "PNG", "img_h": 320, "img_w": 816 },
"url_permalink": "https://scaleflex-tests-v5a.api.airstore.io/v1/get/_/a955877f-c79d-5b7c-8f70-18e7a2950000/Screen Shot 2019-02-28 at 10.03.43 PM.png",
"url_public": "https://scaleflex-tests-v5a.airstore.io/demo_filerobot_en/Screen Shot 2019-02-28 at 10.03.43 PM.png",
"properties": {
"description": "",
"tags": ["Text", "Blue", "Font", "White", "Logo", "Azure", "Line", "Product", "Aqua", "Brand"],
"lang": "en",
"search": " Text Blue Font White Logo Azure Line Product Aqua Brand"
},
"overwrite": false
}]
info: {} - additional information on upload
info.stage: string (upload|edit|select) - stage on which uploadHandler was triggered
container
: string (required)Filerobot Container name.
config.container = 'example';
filerobotUploadKey
: string (required)Unique upload key for Filerobot.
config.filerobotUploadKey = 'xxxxxxxxxxxx';
openpixKey
: string (required)Key for Openpix. Required if you are using "ICONS_GALLERY", "IMAGES_GALLERY"
config.openpixKey = 'xxxxxxxxxxxx';
language
: stringdefault: 'en'
Language of uploader
available languages: en, fr, de, ru
config.language = 'en';
modules
: string[]default: ["UPLOAD", "MY_GALLERY", "ICONS_GALLERY", "IMAGES_GALLERY"]
Modules (tabs) in file uploader modal.
Available modules: UPLOAD, MY_GALLERY, ICONS_GALLERY, IMAGES_GALLERY, TAGGING, IMAGE_EDITOR
config.modules = ['UPLOAD', 'ICONS_GALLERY', 'TAGGING'];
uploadParams
: objectconfig.uploadParams = {
dir: '/folder_name',
...
};
initialTab
: stringdefault: 'UPLOAD'
Allow to choose the initial tab. Should be one of enabled modules.
config.initialTab = 'UPLOAD';
preUploadImageProcess
: booldefault: false
Activates Pre-upload process module which allows to transform images before uploading them to a server. Available operations: "smart crop", "face detection", "resize"
config.preUploadImageProcess = true;
processBeforeUpload
: objectdefault: null
Auto pre-upload process which allows to transform images before uploading them to a server. Available operations: "resize"
config.processBeforeUpload = {
operation: 'resize',
widthLimit: 2000,
heightLimit: 2000
};
folderBrowser
: objectdefault: { show: true, rootFolder: '/' }
Aside menu to browse folders in your container.
show
: bool - show folder manager
rootFolder
: string - limit access to some root folder on server
config.folderBrowser = {
show: true,
rootFolder: '/'
};
sortParams
: objectdefault: { show: true, field: 'name', order: 'asc' }
Default sorting for my gallery tab
show
: bool - show sort module
field
: string - sort field: ['name', 'type', 'uploaded_at', 'modified_at']
order
: string - the sort order can be either asc (ascending/up) or desc (descending/down).
config.sortParams = {
show: true,
field: 'name',
order: 'asc'
};
myGallery
: objectupload
: bool | default: true - possibility to upload in my gallery tabconfig.myGallery = {
upload: true
};
tagging
: objectkey
: string (require) - key to use image recognition technology
executeAfterUpload
: bool - initiates auto-tagging on upload images
autoTaggingButton
: bool - adds button which will automatically generate tags based on image recognition technology
provider
: string [google|imagga] - recognition provider
confidence
: number [0..100] - confidence of recognition
limit
: number - limit of tags generated by image recognition technology
customFields
: array [{ name, metaKey, type }] - custom properties for an image. Where name - name of the field,
metaKey - key/id of the field, type - type of the field: 'text', 'textarea'
suggestionList
: array ['string'] - activates suggestion drop-down list of tags on typing;
config.tagging = {
executeAfterUpload: true,
autoTaggingButton: true,
provider: 'google',
confidence: 60,
limit: 10,
key: 'xxxxx',
customFields: [
{
name: 'Test name 1',
metaKey: 'test_key',
type: 'text'
},
{
name: 'Test name 2',
metaKey: 'test_key_2',
type: 'textarea'
}
],
suggestionList: ['Color', 'Colored', 'Cobalt', 'Coral', 'Cobre']
};
extensions
: arraydefault: [ ]
Allows to limit files according to the extension on upload. Extension jpg = jpeg and vice versa.
config.extensions = ['jpg', 'png', 'pdf'];
modifyURLButton
: booldefault: true
Activate "Modify URL" button.
config.modifyURLButton = true;
deleteButton
: booldefault: true
Display/hide delete button on gallery tab.
config.deleteButton = true;
colorScheme
: objectactive
string (default: 'default'; available schemes: light, dark, solarized, purple, lilac)- active theme scheme
custom
: object - custom color scheme
custom.mainBackground
: color - main background
custom.navBackground
: color - nav background
custom.buttonBackground
: color - button background
custom.hoverButtonBackground
: color - button background on hover
custom.inputBackground
: color - search field background
custom.inputOutlineColor
: color - search field outline
custom.activeTabBackground
: color - current nav tab background
custom.text
: color - text
custom.title
: color - title
custom.inputTextColor
: color - search field text
custom.tabTextColor
: color - nav tab text
custom.activeTabTextColor
: color - current nav tab text
custom.buttonTextColor
: color - button text
custom.border
: color - draggable boundaries border
let config = {
...,
colorScheme: {
active: 'custom',
custom: {
mainBackground: '#f5f5f5',
navBackground: '#181830',
buttonBackground: '#00707C',
hoverButtonBackground: '#096868',
inputBackground: '#fff',
inputOutlineColor: '#4d90fe',
activeTabBackground: '#40545b',
text: '#5d636b',
title: '#1e262c',
inputTextColor: '#555555',
tabTextColor: '#c0c1c1',
activeTabTextColor: '#fff',
buttonTextColor: '#fff',
border: '#d8d8d8'
}
}
};
imageEditorConfig
: objectFilerobot Image Editor Configuration. https://github.com/scaleflex/filerobot-image-editor#table-of-contents
<script>
let config = {
modules: ['UPLOAD', 'MY_GALLERY', 'ICONS_GALLERY', 'IMAGES_GALLERY', 'TAGGING', 'IMAGE_EDITOR'],
uploadParams: {
dir: '/your_root_folder'
},
filerobotUploadKey: '0cbe9ccc4f164bf8be26bd801d53b132',
container: 'example',
openpixKey: 'xxxxxxxxxxxxxxx',
initialTab: 'UPLOAD',
folderBrowser: true,
tagging: {
executeAfterUpload: true,
autoTaggingButton: true,
provider: 'google',
confidence: 60,
limit: 10,
key: 'aaaa'
},
language: 'en',
colorScheme: {
active: 'custom',
custom: {
mainBackground: '#f5f5f5',
navBackground: '#181830',
buttonBackground: '#00707C',
hoverButtonBackground: '#096868',
inputBackground: '#fff',
inputOutlineColor: '#4d90fe',
activeTabBackground: '#40545b',
text: '#5d636b',
title: '#1e262c',
inputTextColor: '#555555',
tabTextColor: '#c0c1c1',
activeTabTextColor: '#fff',
buttonTextColor: '#fff',
border: '#d8d8d8'
}
}
};
let onUpload = (files) => {
console.log('files: ', files);
alert('Files uploaded successfully! check the console to see the uploaded files');
};
let uploader = FilerobotUploader.init(config, onUpload);
let button = document.createElement('button');
button.onclick = () => { uploader.open(); }
button.innerText = 'Open Uploader';
document.body.appendChild(button);
</script>
All contributions are super welcome!
Filerobot Uploader is provided under the MIT License
FAQs
[DEPRECATED] The Filerobot Uploader is a multi-function Uploader that will make uploads super easy in your web sites and apps. It is fast to integrate allows end users to upload media, files and any assets via Filerobot's reverse CDN. Files are stored int
We found that filerobot-uploader demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.