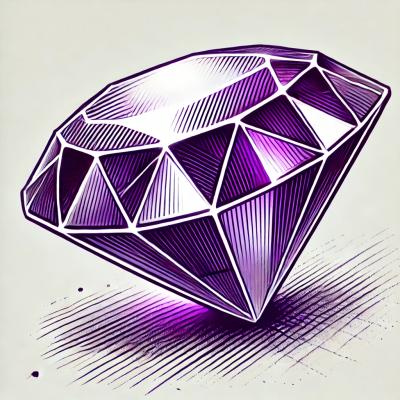
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
filestack-js
Advanced tools
Official browser client to the Filestack API's. Available via NPM and CDN script.
Filestack documentation can be found here: https://filestack.com/docs/
npm install --save filestack-js
Via ES6 modules:
import filestack from 'filestack-js';
const apikey = 'abc';
const client = filestack.init(apikey);
Via script tag:
<script src="http://static.filestackapi.com/v3/filestack.js"></script>
<script>
const apikey = 'abc';
const client = filestack.init(apikey);
</script>
string
object
object
object
Promise
Promise
Promise
Promise
Promise
string
Promise
object
function
function
string
Gets current version.
Kind: inner property of filestack
Example
import filestack from 'filestack-js';
console.log(filestack.version);
object
Initializes the client.
Kind: inner method of filestack
Returns: object
- Object containing the available methods documented below.
Params
string
- Filestack API key. Get a free key here.object
- Read about security policies.
string
- Filestack security policy encoded in base64.string
- HMAC-SHA256 sIgnature for the security policy.object
Get current security parameters
Kind: static method of init
Returns: object
- Object containing current security parameters
object
Set security parameters -- useful for changing policy on instantiated client
Kind: static method of init
Returns: object
- Object containing current session parameters
Params
object
- Read about security policies.
string
- Filestack security policy encoded in base64.string
- HMAC-SHA256 sIgnature for the security policy.Example
client.setSecurity({ policy: 'policy', signature: 'signature' });
Promise
⏏Opens the picker UI.
Kind: static method of init
Fulfil: object
- Object contains keys filesUploaded
and filesFailed
which are both arrays of file metadata.
Params
object
Array.<string>
- Valid sources are:
local_file_system
- Defaultimagesearch
- Defaultfacebook
- Defaultinstagram
- Defaultgoogledrive
- Defaultdropbox
- Defaultevernote
flickr
box
github
gmail
picasa
onedrive
clouddrive
string
| Array.<string>
- Restrict file types that are allowed to be picked. Formats accepted:
.pdf
<- any file extensionimage/jpeg
<- any mime type commonly known by browsersimage/*
<- special mime type accepting all types of imagesvideo/*
<- special mime type accepting all types of video filesaudio/*
<- special mime type accepting all types of audio filesboolean
= false
- For cloud sources whether to link or store files.number
= 1
- Minimum number of files required to start uploading.number
= 1
- Maximum number of files allowed to upload.boolean
= false
- Whether to start uploading automatically when maxFiles is hit.boolean
= false
- Hide the picker UI once uploading begins.boolean
= true
- Start uploading immediately on file selection.boolean
= false
- When true removes ability to edit images with transformer UI.object
- Options to be passed to the transformer UI.
array
- Minimum dimensions for picked image. Image will be upscaled if smaller. (e.g. [200, 300])array
- Maximum dimensions for picked image. Image will be downscaled if smaller. (e.g. [200, 300])object
- Enable and set options for various transformations.
boolean
| object
- Enable crop.
number
- Maintain aspect ratio for crop selection. (e.g. 16/9 or 4/3)boolean
- Enable rotate.boolean
- Enable circle.boolean
- Enable monochrome.boolean
- Enable sepia.object
- Options for file storage.
string
= "s3"
- One of s3
, gcs
, rackspace
, azure
, dropbox
.string
- Valid S3 region for the selected container (S3 only).string
string
string
- One of public
or private
.onFileSelected
- Called whenever user selects a file.onFileUploadStarted
- Called when a file begins uploading.onFileUploadProgress
- Called during multi-part upload progress events.onFileUploadFinished
- Called when a file is done uploading.onFileUploadFailed
- Called when uploading a file fails.Example
client.pick({
maxFiles: 20,
fromSources: ['local_file_system', 'facebook'],
}).then(res => console.log(res));
function
Kind: inner typedef of pick
Params
object
- File metadata.Example
// Using to veto file selection
// If you throw any error in this function it will reject the file selection.
// The error message will be displayed to the user as an alert.
onFileSelected(file) {
if (file.size > 1000 * 1000) {
throw new Error('File too big, select something smaller than 1MB');
}
}
// Using to change selected file name
onFileSelected(file) {
file.name = 'foo';
// It's important to return altered file by the end of this function.
return file;
}
function
Kind: inner typedef of pick
Params
object
- File metadata.function
Kind: inner typedef of pick
Params
object
- File metadata.function
Kind: inner typedef of pick
Params
object
- File metadata.error
- Error instance for this upload.function
Kind: inner typedef of pick
Params
object
- File metadata.object
- Progress event.
number
- Percent of total upload.number
- Total number of bytes uploaded thus far across all parts.number
- Part #.number
- Amount of data in this part that has been uploaded.number
- Total number of bytes in this part.Promise
Interface to the Filestack Store API. Used for storing from a URL.
Kind: static method of init
Fulfil: object
- Metadata of stored file.
Reject: error
- A Superagent error object.
Params
string
- Valid URL to a file.object
string
string
= "s3"
- One of s3
, gcs
, rackspace
, azure
, dropbox
.string
string
string
string
- Valid S3 region for the selected container (S3 only).string
- One of public
or private
.boolean
Example
client
.storeURL('https://d1wtqaffaaj63z.cloudfront.net/images/NY_199_E_of_Hammertown_2014.jpg')
.then(res => console.log(res));
Promise
Interface to the Filestack Retrieve API. Used for accessing files via Filestack handles.
Kind: static method of init
Fulfil: object
- Metadata of stored file or stored file, depending on metadata / head option.
Reject: error
- A Superagent error object.
Params
string
- Valid Filestack handle.object
boolean
- return json of file metadataboolean
- perform a 'head' request instead of a 'get'boolean
- X-File-Name will be returnedstring
- add extension to handleExample
client
.retrieve('DCL5K46FS3OIxb5iuKby')
.then((blob) => {
var urlCreator = window.URL || window.webkitURL;
var imageUrl = urlCreator.createObjectURL(blob);
document.querySelector('#myImage').src = imageUrl;
})
.catch((err) => {
console.log(err);
}));
Promise
Interface to the Filestack Remove API. Used for removing files, requires security to be enabled.
Kind: static method of init
Fulfil: object
- Result of remove.
Reject: error
- A Superagent error object.
Params
string
- Valid Filestack handle.Example
client
.remove('DCL5K46FS3OIxb5iuKby')
.then((res) => {
console.log(res);
})
.catch((err) => {
console.log(err);
}));
Promise
Interface to the Filestack Metadata API. Used for retrieving detailed data of stored files.
Kind: static method of init
Fulfil: object
- Result of metadata.
Reject: error
- A Superagent error object.
Params
string
- Valid Filestack handle.object
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
Example
client
.metadata('DCL5K46FS3OIxb5iuKby')
.then((res) => {
console.log(res);
})
.catch((err) => {
console.log(err);
}));
string
Interface to the Filestack transformation engine.
Kind: static method of init
Returns: string
- A new URL that points to the transformed resource.
Params
string
- Valid URL to an image.TransformOptions
- Transformations are applied in the order specified by this object.Example
const transformedUrl = client.transform(url, {
crop: {
dim: {
x: 0,
y: 50,
width: 300,
height: 300,
},
},
vignette: {
blurmode: 'gaussian',
amount: 50,
},
flip: true,
};
// optionally store the new URL
client.storeURL(transformedUrl).then(res => console.log(res));
Promise
Initiates a multi-part upload flow.
Kind: static method of init
Fulfil: object
- Metadata of uploaded file.
Reject: error
- An error object depending on where the flow halted.
Params
File
- must be a valid File.object
number
= 5 * 1024 * 1024
- Size of each uploaded part.number
= 5
- Max number of concurrent uploads.object
- params for retry settings
number
= 10
- max number of retriesnumber
= 2
- the exponential factor to usenumber
= 1 * 1000
- ms before starting first retrynumber
= 60 * 1000
- max ms between two retriesfunction
- Called when the flow begins (before Filestack handshake request is made).function
- Called when an upload begins (after S3 request is made).progressCallback
- Called on progress event.retryCallback
- Called if upload fails and retry is occurringfunction
- Called when an upload is completing (before final completion request).object
- Configure where the file is stored.
string
- Valid options are: s3
, gcs
, dropbox
, azure
, rackspace
.string
- Name of the storage container.string
- Valid S3 region for the selected container (S3 only).string
- Path where the file will be stored. A trailing slash will put the file in that folder path.string
- Valid options are private
or public
.Example
client.upload(file).then(res => console.log(res));
object
Kind: inner typedef of filestack
Params
object
- Crop options.
object
- Crop dimensions.
number
number
number
number
object
- Resize options. At least one option is required.
number
number
string
- One of clip
, crop
, scale
, max
.string
- One of center
, top
, bottom
, left
, right
, faces
, or align pair like ['top', 'left']
.object
- Rotate options. At least one option is required.
number
| string
- Can be number in range 0-359 or exif
.boolean
string
boolean
- Flip imageboolean
- Flop imageobject
- Rounded corners options.
number
| string
- Can be number in range 1-10000 or max
.number
string
object
- Vignette options.
number
string
- One of linear
or gaussian
.string
object
- Polaroid options.
string
number
string
object
- Torn edges options.
Array.<number>
- Range format [10, 50]
.string
object
- Shadow options.
number
number
Array.<number>
- Range format [25, 25]
.string
string
object
- Circle options.
string
object
- Border options.
number
number
number
boolean
- Monochrome.object
- Sepia.
number
function
Kind: inner typedef of filestack
Params
object
- Progress event.
number
- Percent of total upload.number
- Total number of bytes uploaded thus far across all parts.number
- Part #.number
- Amount of data in this part that has been uploaded.number
- Total number of bytes in this part.function
Kind: inner typedef of filestack
Params
number
- Which attempt the upload is currently on.number
- ms before the attempt will start© 2017 Filestack.
0.3.0 (2017-03-14)
FAQs
Official JavaScript library for Filestack
The npm package filestack-js receives a total of 0 weekly downloads. As such, filestack-js popularity was classified as not popular.
We found that filestack-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.