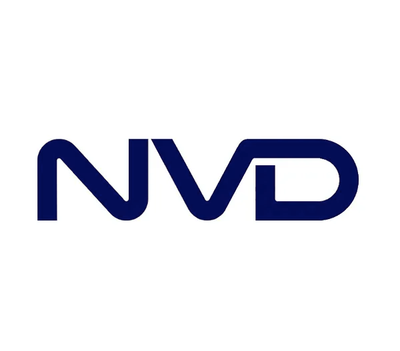
Security News
NVD Backlog Tops 20,000 CVEs Awaiting Analysis as NIST Prepares System Updates
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
find-my-way
Advanced tools
The find-my-way npm package is a fast HTTP router for Node.js. It is designed to help developers create routing systems for web applications and APIs. It supports dynamic and static routing, HTTP methods, middleware, hooks, and versioning.
Static Routing
Static routing allows you to define routes for specific paths. When a request matches the path, the associated handler is called.
const findMyWay = require('find-my-way')({ defaultRoute: (req, res) => { res.end('Not Found') } });
findMyWay.on('GET', '/example', (req, res, params) => { res.end('This is a static route example'); });
Dynamic Routing
Dynamic routing supports path parameters, enabling you to capture values from the URL path and pass them to your handler function.
findMyWay.on('GET', '/example/:param', (req, res, params) => { res.end(`Parameter value: ${params.param}`); });
HTTP Methods
The package supports various HTTP methods, allowing you to define different handlers for GET, POST, PUT, DELETE, etc.
findMyWay.on('POST', '/example', (req, res, params) => { res.end('This is a POST method example'); });
Middleware Support
Middleware functions can be used to perform actions before the final handler is executed.
findMyWay.use('/example', (req, res, params, next) => { console.log('Middleware executed'); next(); });
Versioned Routes
Versioning allows you to define different handlers for different versions of your API.
findMyWay.on('GET', '/example', { version: '1.0.0' }, (req, res, params) => { res.end('This is version 1.0.0'); });
Express is a widely-used web application framework for Node.js. It provides a robust set of features for web and mobile applications, including routing, middleware, and template engines. Compared to find-my-way, Express offers a more comprehensive solution with additional features beyond routing.
Koa-router is a router middleware for Koa, another Node.js web framework. It offers similar functionalities to find-my-way, such as HTTP method support and dynamic routing, but is specifically designed to work within the Koa ecosystem.
Hapi is a rich framework for building applications and services, which includes powerful plugin capabilities. It provides a more extensive set of features than find-my-way, including input validation, caching, authentication, and more.
A crazy fast HTTP router, internally uses an highly performant Radix Tree (aka compact Prefix Tree), supports route params, wildcards, and it's framework independent.
If you want to see a benchmark comparison with the most commonly used routers, see here.
Do you need a real-world example that uses this router? Check out Fastify.
npm i find-my-way --save
const http = require('http')
const router = require('find-my-way')()
router.on('GET', '/', (req, res, params) => {
res.end('{"hello":"world"}')
})
const server = http.createServer((req, res) => {
router.lookup(req, res)
})
server.listen(3000, err => {
if (err) throw err
console.log('Server listening on: http://localost:3000')
})
Instance a new router.
You can pass a default route with the option defaultRoute
.
const router = require('find-my-way')({
defaultRoute: (req, res) => {
res.statusCode = 404
res.end()
}
})
Register a new route, store
is an object that you can access later inside the handler function.
router.on('GET', '/', (req, res, params) => {
// your code
})
// with store
router.on('GET', '/store', (req, res, params, store) => {
// the store can be updated
assert.equal(store, { hello: 'world' })
}, { hello: 'world' })
If you want to register a parametric path, just use the colon before the parameter name, if you need a wildcard use the star.
Remember that static routes are always inserted before parametric and wildcard.
// parametric
router.on('GET', '/example/:name', () => {}))
// wildcard
router.on('GET', '/other-example/*', () => {}))
Regex routes are supported as well, but pay attention, regex are very expensive!
// parametric with regex
router.on('GET', '/test/:file(^\\d+).png', () => {}))
If you want an even nicer api, you can also use the shorthand methods to declare your routes.
router.get(path, handler [, store])
router.delete(path, handler [, store])
router.head(path, handler [, store])
router.patch(path, handler [, store])
router.post(path, handler [, store])
router.put(path, handler [, store])
router.options(path, handler [, store])
router.trace(path, handler [, store])
router.connect(path, handler [, store])
Start a new search, request
and response
are the server req/res objects.
If a route is found it will automatically called the handler, otherwise the default route will be called.
The url is sanitized internally, all the parameters and wildcards are decoded automatically.
router.lookup(req, res)
Return (if present) the route registered in method:path.
The path must be sanitized, all the parameters and wildcards are decoded automatically.
router.find('GET', '/example')
// => { handler: Function, params: Object, store: Object}
// => null
Prints the representation of the internal radix tree, useful for debugging.
findMyWay.on('GET', '/test', () => {})
findMyWay.on('GET', '/test/hello', () => {})
findMyWay.on('GET', '/hello/world', () => {})
console.log(findMyWay.prettyPrint())
// └── /
// ├── test (GET)
// │ └── /hello (GET)
// └── hello/world (GET)
This project is kindly sponsored by LetzDoIt.
It is inspired by the echo router, some parts have been extracted from trekjs router.
find-my-way - MIT
trekjs/router - MIT
Copyright © 2017 Tomas Della Vedova
FAQs
Crazy fast http radix based router
The npm package find-my-way receives a total of 2,218,301 weekly downloads. As such, find-my-way popularity was classified as popular.
We found that find-my-way demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.