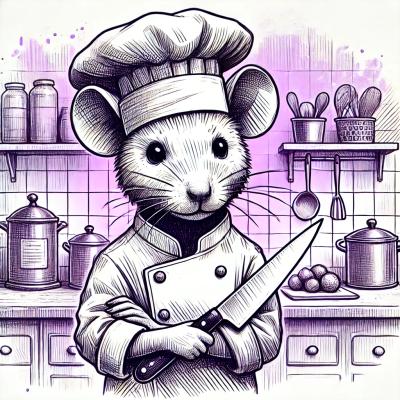
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
The findit2 npm package is a utility for recursively finding files and directories within a given directory. It is useful for tasks such as searching for specific files, filtering files by certain criteria, and performing actions on files or directories as they are found.
Finding all files in a directory
This feature allows you to find all files within a specified directory. The code sample demonstrates how to use the 'file' event to log each file found.
const findit = require('findit2');
const finder = findit('/path/to/dir');
finder.on('file', (file, stat) => {
console.log('File: ' + file);
});
Finding all directories in a directory
This feature allows you to find all directories within a specified directory. The code sample demonstrates how to use the 'directory' event to log each directory found.
const findit = require('findit2');
const finder = findit('/path/to/dir');
finder.on('directory', (dir, stat) => {
console.log('Directory: ' + dir);
});
Filtering files by extension
This feature allows you to filter files by their extension. The code sample demonstrates how to log only '.txt' files found in the specified directory.
const findit = require('findit2');
const finder = findit('/path/to/dir');
finder.on('file', (file, stat) => {
if (file.endsWith('.txt')) {
console.log('Text file: ' + file);
}
});
The glob package is a powerful tool for matching files using patterns. It supports advanced pattern matching features and is highly configurable. Compared to findit2, glob is more focused on pattern matching and less on event-driven file discovery.
The readdirp package is a recursive version of fs.readdir, allowing you to read directories and their subdirectories. It provides a stream-based API for handling large directories efficiently. Compared to findit2, readdirp is more focused on providing a stream-based approach to directory reading.
The walk package is a simple and efficient directory tree walker with support for filtering and event handling. It is similar to findit2 in its event-driven approach but offers additional features such as the ability to pause and resume the walk process.
Recursively walk directory trees. Think /usr/bin/find
.
There is a pull request to merge this project back into findit.
The pull request fixes every open issue in findit, and it completely rewrites the code from the ground up.
It also adds an additional feature regarding symlinks.
I would love for substack to merge the pull request, but realistically it might not happen, and this code is objectively cleaner, more robust, and fixes several critical issues.
I recommend depending on this module rather than the original findit. If the pull request is merged, however, I will add a deprecation notice to this module and happily hand the maintainer hat back to substack.
var finder = require('findit2')(process.argv[2] || '.');
var path = require('path');
finder.on('directory', function (dir, stat, stop, linkPath) {
var base = path.basename(dir);
if (base === '.git' || base === 'node_modules') stop()
else console.log(dir + '/')
});
finder.on('file', function (file, stat, linkPath) {
console.log(file);
});
finder.on('link', function (link, stat) {
console.log(link);
});
var findit = require('findit2')
Return an event emitter finder
that performs a recursive walk starting at
basedir
.
If you set opts.followSymlinks
, symlinks will be followed. Otherwise, a
'link'
event will fire but symlinked directories will not be walked.
If basedir
is actually a non-directory regular file, findit emits a single
"file" event for it then emits "end".
You can optionally specify a custom
fs
implementation with opts.fs
. opts.fs
should implement:
opts.fs.readdir(dir, cb)
opts.fs.lstat(dir, cb)
opts.fs.readlink(dir, cb)
- optional if your stat objects from
opts.fs.lstat
never return true for stat.isSymbolicLink()
Stop the traversal. A "stop"
event will fire and then no more events will
fire.
For each file, directory, and symlink file
, this event fires.
If followSymlinks
is true
, then linkPath
will be defined when file
was found via a symlink. In this situation, linkPath
is the path including
the symlink; file
is the resolved actual location on disk.
For each file, this event fires.
For each directory, this event fires with the path dir
.
Your callback may call stop()
on the first tick to tell findit to stop walking
the current directory.
For each symlink, this event fires.
Every time a symlink is read when opts.followSymlinks
is on, this event fires.
When the recursive walk is complete unless finder.stop()
was called, this
event fires.
When finder.stop()
is called, this event fires.
Whenever there is an error, this event fires. You can choose to ignore errors or
stop the traversal using finder.stop()
.
You can always get the source of the error by checking err.path
.
With npm do:
npm install findit2
FAQs
walk a directory tree recursively with events
The npm package findit2 receives a total of 456,498 weekly downloads. As such, findit2 popularity was classified as popular.
We found that findit2 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.