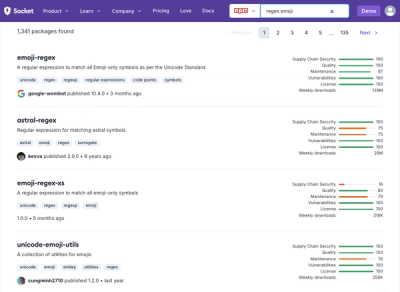
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
A finite state machine library for Node.js and the browser with a friendly configuration DSL
The finity npm package is a lightweight and flexible state machine library for JavaScript. It allows you to define and manage states and transitions in a clear and concise manner, making it easier to handle complex state logic in your applications.
Define a State Machine
This code sample demonstrates how to define a simple state machine with three states: 'idle', 'running', and 'paused'. It shows how to configure transitions between these states based on events such as 'start', 'pause', 'resume', and 'stop'.
const finity = require('finity');
const stateMachine = finity
.configure()
.initialState('idle')
.state('idle')
.on('start').transitionTo('running')
.state('running')
.on('pause').transitionTo('paused')
.on('stop').transitionTo('idle')
.state('paused')
.on('resume').transitionTo('running')
.on('stop').transitionTo('idle')
.start();
stateMachine.handle('start'); // Transition to 'running'
stateMachine.handle('pause'); // Transition to 'paused'
stateMachine.handle('resume'); // Transition to 'running'
stateMachine.handle('stop'); // Transition to 'idle'
Handle State Transitions
This code sample shows how to handle state transitions and retrieve the current state of the state machine. It demonstrates transitioning from 'idle' to 'running' and then to 'paused', and how to check the current state after each transition.
const finity = require('finity');
const stateMachine = finity
.configure()
.initialState('idle')
.state('idle')
.on('start').transitionTo('running')
.state('running')
.on('pause').transitionTo('paused')
.on('stop').transitionTo('idle')
.state('paused')
.on('resume').transitionTo('running')
.on('stop').transitionTo('idle')
.start();
stateMachine.handle('start'); // Transition to 'running'
console.log(stateMachine.getCurrentState()); // Output: 'running'
stateMachine.handle('pause'); // Transition to 'paused'
console.log(stateMachine.getCurrentState()); // Output: 'paused'
Add Entry and Exit Actions
This code sample demonstrates how to add entry and exit actions to states. It shows how to log messages when entering and exiting states, providing a way to execute custom logic during state transitions.
const finity = require('finity');
const stateMachine = finity
.configure()
.initialState('idle')
.state('idle')
.onEntry(() => console.log('Entering idle state'))
.on('start').transitionTo('running')
.state('running')
.onEntry(() => console.log('Entering running state'))
.onExit(() => console.log('Exiting running state'))
.on('pause').transitionTo('paused')
.on('stop').transitionTo('idle')
.state('paused')
.onEntry(() => console.log('Entering paused state'))
.on('resume').transitionTo('running')
.on('stop').transitionTo('idle')
.start();
stateMachine.handle('start'); // Logs 'Entering running state'
stateMachine.handle('pause'); // Logs 'Exiting running state' and 'Entering paused state'
stateMachine.handle('resume'); // Logs 'Exiting paused state' and 'Entering running state'
XState is a robust and feature-rich state machine library for JavaScript and TypeScript. It provides a comprehensive set of tools for defining, visualizing, and interpreting state machines and statecharts. Compared to finity, XState offers more advanced features such as hierarchical states, parallel states, and built-in support for statechart visualization.
The javascript-state-machine package is a simple and lightweight state machine library for JavaScript. It allows you to define states and transitions in a straightforward manner. While it is similar to finity in terms of simplicity, it lacks some of the advanced features and flexibility that finity offers.
Machina is a powerful state machine library for JavaScript that supports hierarchical states, event emitters, and more. It is designed for complex state management scenarios and provides a rich API for defining and managing states. Compared to finity, machina offers more advanced features and is suitable for more complex use cases.
FAQs
A finite state machine library for Node.js and the browser with a friendly configuration DSL
The npm package finity receives a total of 335,505 weekly downloads. As such, finity popularity was classified as popular.
We found that finity demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.