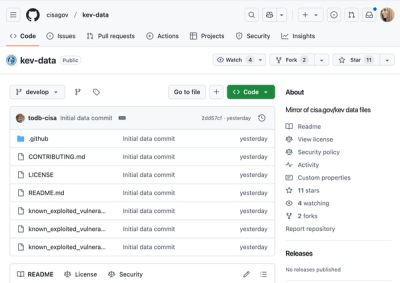
Security News
CISA Brings KEV Data to GitHub
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.
five-bells-condition
Advanced tools
Implementation of Interledger condition validation and fulfillment
Implementation of crypto-conditions
Editor's Draft: draft-thomas-crypto-conditions-01
This specification is only a draft at this stage and has not been submitted.
Number
Number
Buffer
Number
String
Buffer
Boolean
Condition
Condition
Number
Number
Condition
String
Buffer
String
Buffer
Boolean
Fulfillment
Fulfillment
Number
Boolean
const cc = require('five-bells-condition')
// Check a condition for validity
const condition = 'cc:0:3:47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU:0'
const validationResult = cc.validateCondition(condition)
// validationResult === true
This will ensure that the requested type, features and fulfillment length are all accepted by the current implementation.
const cc = require('five-bells-condition')
const condition = 'cc:0:3:47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU:0'
const fulfillment = 'cf:0:'
const validationResult = cc.validateFulfillment(fulfillment, condition)
// validationResult === true
This validates the fulfillment and ensures that it matches the given condition.
const cc = require('five-bells-condition')
const fulfillment = 'cf:0:'
const condition = cc.fulfillmentToCondition(fulfillment)
// You could now look up this condition in your database etc.
const validationResult = cc.validateFulfillment(fulfillment, condition)
// validationResult === true
const cc = require('five-bells-condition')
const myFulfillment = new cc.PreimageSha256()
myFulfillment.setPreimage(new Buffer(''))
console.log(myFulfillment.getConditionUri())
// prints 'cc:0:3:47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU:0'
const cc = require('five-bells-condition')
const myFulfillment = new cc.PreimageSha256()
myFulfillment.setPreimage(new Buffer(''))
console.log(myFulfillment.serializeUri())
// prints 'cf:0:'
const cc = require('five-bells-condition')
const parsedFulfillment = cc.fromFulfillmentUri('cf:0:')
// parsedFulfillment instanceof cc.PreimageSha256 === true
// Note: Merely parsing a fulfillment DOES NOT validate it.
// Validate a fulfillment
parsedFulfillment.validate()
const cc = require('five-bells-condition')
const ed25519Fulfillment = new cc.Ed25519()
ed25519Fulfillment.setPublicKey(new Buffer('ec172b93ad5e563bf4932c70e1245034c35467ef2efd4d64ebf819683467e2bf', 'hex'))
console.log(ed25519Fulfillment.getConditionUri())
// prints 'cc:4:20:7Bcrk61eVjv0kyxw4SRQNMNUZ-8u_U1k6_gZaDRn4r8:96'
const cc = require('five-bells-condition')
const edPrivateKey = new Buffer('833fe62409237b9d62ec77587520911e9a759cec1d19755b7da901b96dca3d42', 'hex')
const ed25519Fulfillment = new cc.Ed25519()
// ed25519Fulfillment.setPublicKey(new Buffer('...'))
// ed25519Fulfillment.setSignature(new Buffer('...'))
// -- or --
ed25519Fulfillment.sign(new Buffer('Hello World! Conditions are here!'), edPrivateKey)
console.log(ed25519Fulfillment.getConditionUri())
// prints 'cc:4:20:7Bcrk61eVjv0kyxw4SRQNMNUZ-8u_U1k6_gZaDRn4r8:96'
console.log(ed25519Fulfillment.serializeUri())
// prints 'cf:4:7Bcrk61eVjv0kyxw4SRQNMNUZ-8u_U1k6_gZaDRn4r-2IpH62UMvjymLnEpIldvik_b_2hpo2t8Mze9fR6DHISpf6jzal6P0wD6p8uisHOyGpR1FISer26CdG28zHAcK'
const cc = require('five-bells-condition')
const fulfillment = 'cf:4:7Bcrk61eVjv0kyxw4SRQNMNUZ-8u_U1k6_gZaDRn4r-2IpH62UMvjymLnEpIldvik_b_2hpo2t8Mze9fR6DHISpf6jzal6P0wD6p8uisHOyGpR1FISer26CdG28zHAcK'
const condition = 'cc:4:20:7Bcrk61eVjv0kyxw4SRQNMNUZ-8u_U1k6_gZaDRn4r8:96'
const message = new Buffer('Hello World! Conditions are here!')
const result = cc.validateFulfillment(fulfillment, condition, message)
// result === true
const cc = require('five-bells-condition')
const thresholdFulfillment = new cc.ThresholdSha256()
thresholdFulfillment.addSubconditionUri('cc:4:20:7Bcrk61eVjv0kyxw4SRQNMNUZ-8u_U1k6_gZaDRn4r8:96')
thresholdFulfillment.addSubfulfillmentUri('cf:0:')
thresholdFulfillment.setThreshold(1) // defaults to subconditions.length
console.log(thresholdFulfillment.getConditionUri())
// prints 'cc:2:2b:mJUaGKCuF5n-3tfXM2U81VYtHbX-N8MP6kz8R-ASwNQ:146'
const cc = require('five-bells-condition')
const thresholdFulfillment = new cc.ThresholdSha256()
thresholdFulfillment.addSubfulfillmentUri('cf:4:7Bcrk61eVjv0kyxw4SRQNMNUZ-8u_U1k6_gZaDRn4r-2IpH62UMvjymLnEpIldvik_b_2hpo2t8Mze9fR6DHISpf6jzal6P0wD6p8uisHOyGpR1FISer26CdG28zHAcK')
thresholdFulfillment.addSubfulfillmentUri('cf:0:')
thresholdFulfillment.setThreshold(1) // defaults to subconditions.length
console.log(thresholdFulfillment.getConditionUri())
// prints 'cc:2:2b:mJUaGKCuF5n-3tfXM2U81VYtHbX-N8MP6kz8R-ASwNQ:146'
const thresholdFulfillmentUri = thresholdFulfillment.serializeUri()
// Note: If there are more than enough fulfilled subconditions, shorter
// fulfillments will be chosen over longer ones.
// thresholdFulfillmentUri.length === 77
console.log(thresholdFulfillmentUri)
// prints 'cf:2:AQEBAgEBAwAAAAABAQAnAAQBICDsFyuTrV5WO_STLHDhJFA0w1Rn7y79TWTr-BloNGfivwFg'
const cc = require('five-bells-condition')
const prefix = new cc.PrefixSha256()
prefix.setPrefix(new Buffer('2016:'))
prefix.setSubconditionUri('cc:4:20:7Bcrk61eVjv0kyxw4SRQNMNUZ-8u_U1k6_gZaDRn4r8:96')
console.log(prefix.getConditionUri())
// prints 'cc:1:25:7myveZs3EaZMMuez-3kq6u69BDNYMYRMi_VF9yIuFLc:102'
const cc = require('five-bells-condition')
const prefix = new cc.PrefixSha256()
prefix.setPrefix(new Buffer('Hello World! '))
prefix.setSubfulfillmentUri('cf:4:7Bcrk61eVjv0kyxw4SRQNMNUZ-8u_U1k6_gZaDRn4r-2IpH62UMvjymLnEpIldvik_b_2hpo2t8Mze9fR6DHISpf6jzal6P0wD6p8uisHOyGpR1FISer26CdG28zHAcK')
const fulfillmentUri = prefix.serializeUri()
console.log(fulfillmentUri)
// prints 'cf:1:DUhlbGxvIFdvcmxkISAABGDsFyuTrV5WO_STLHDhJFA0w1Rn7y79TWTr-BloNGfiv7YikfrZQy-PKYucSkiV2-KT9v_aGmja3wzN719HoMchKl_qPNqXo_TAPqny6Kwc7IalHUUhJ6vboJ0bbzMcBwo'
const conditionUri = prefix.getConditionUri()
const message = new Buffer('Conditions are here!')
cc.validateFulfillment(fulfillmentUri, conditionUri, message)
const cc = require('five-bells-condition')
const rsaFulfillment = new cc.RsaSha256()
rsaFulfillment.setPublicModulus(new Buffer('b30e7a938783babf836850ff49e14f87e3f92d5c46e33feca3e4f0b22358580b11765995f4b8eea7fb4712c2e1e316f7f775a953d232216a169d9a64ddc007120a400b37f2afc077b62fe304de74de6a119ec4076b529c4f6096b0baad4f533df0173b9b822fd85d65fa4befa92d8f524f69cbca0136bd80d095c169aec0e095', 'hex'))
console.log(rsaFulfillment.getConditionUri())
// prints 'cc:3:11:Bw-r77AGqSCL0huuMQYj3KW0Jh67Fpayeq9h_4UJctg:260'
const cc = require('five-bells-condition')
const exampleMessage = new Buffer('Hello World! Conditions are here!')
const privateKey =
'-----BEGIN RSA PRIVATE KEY-----\n' +
'MIICXAIBAAKBgQCzDnqTh4O6v4NoUP9J4U+H4/ktXEbjP+yj5PCyI1hYCxF2WZX0\n' +
'uO6n+0cSwuHjFvf3dalT0jIhahadmmTdwAcSCkALN/KvwHe2L+ME3nTeahGexAdr\n' +
'UpxPYJawuq1PUz3wFzubgi/YXWX6S++pLY9ST2nLygE2vYDQlcFprsDglQIDAQAB\n' +
'AoGAB7Rjyd1W6b475U027vLm/S3uFumVk0m44QSE5uVmc8NmKPWJ4lHi0w+Y61G/\n' +
'booaeWdytcyho5ZxCq8OEAynQSkJiBNtzBg+xCGcO6GPOf+dFBYZFQsXiG/EbwrA\n' +
'pT0cv+AqiGzLIAh2WtNI6cr5/ZEMScNhMcQ4AZ1kRyUdpIECQQDbRtFz0dSMMvS/\n' +
'1KtDZxej9HqC5xOEuCDEZuLvk4bW4mC02OP/H/VV5qqclz0LIvMWK6TDtoFRpkvD\n' +
'UYiYoc85AkEA0QtH1zQlGGlliLcWoPeqjkbtf3ocmYy2exBSCwnOf87xV//k9pNC\n' +
'7jmoIzRgKVef8kQR/mXWszo3WbWMt0aAPQJBAMtoRD/GM/7h/fw9Uamy5lEnJsZr\n' +
'iMWi8HKAZp+LIJgRY1gfolA12yWWVknwWaYNA6ZbUfpjQE73jmxfI/FCmLECQBmF\n' +
'WAr06cZ2L5gmShPyyJbAIASdItq4LBsQHgQM+XHvENXeftR/m/87eMR7g3XopbVN\n' +
'DClTw4d0Bwfjuz8w0z0CQFG7RmgPqsTEGfojpRgLZnec87R6XhuUY5ZoGgpnx7r9\n' +
'/zGekAwjBZDKpc+H0jC14JjMzRRKeWVEpDU3k2cfBH0=\n' +
'-----END RSA PRIVATE KEY-----\n'
const rsaFulfillment = new cc.RsaSha256()
// rsaFulfillment.setPublicModulus(new Buffer('...'))
// rsaFulfillment.setSignature(new Buffer('...'))
// -- or --
// TODO: In the future the modulus should be extracted from the private key.
rsaFulfillment.setPublicModulus(new Buffer('b30e7a938783babf836850ff49e14f87e3f92d5c46e33feca3e4f0b22358580b11765995f4b8eea7fb4712c2e1e316f7f775a953d232216a169d9a64ddc007120a400b37f2afc077b62fe304de74de6a119ec4076b529c4f6096b0baad4f533df0173b9b822fd85d65fa4befa92d8f524f69cbca0136bd80d095c169aec0e095', 'hex'))
rsaFulfillment.sign(exampleMessage, privateKey)
console.log(rsaFulfillment.serializeUri().length)
// prints '352'
// Verify RSA-SHA256 condition
const rsaFulfillmentUri = rsaFulfillment.serializeUri()
const rsaConditionUri = rsaFulfillment.getConditionUri()
cc.validateFulfillment(rsaFulfillmentUri, rsaConditionUri, exampleMessage)
const cc = require('five-bells-condition')
// Parse a condition
const condition = 'cc:0:3:47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU:0'
const parsedCondition = cc.fromConditionUri(condition)
console.log(parsedCondition.constructor.name)
// prints 'Condition'
// Compile to a condition
console.log(parsedCondition.serializeUri())
// prints condition
const cc = require('five-bells-condition')
const thresholdFulfillmentUri = 'cf:2:AQEBAgEBAwAAAAABAQAnAAQBICDsFyuTrV5WO_STLHDhJFA0w1Rn7y79TWTr-BloNGfivwFg'
const reparsedFulfillment = cc.fromFulfillmentUri(thresholdFulfillmentUri)
const reserializedFulfillment = reparsedFulfillment.serializeUri()
console.log(reserializedFulfillment)
// prints thresholdFulfillmentUri
const cc = require('five-bells-condition')
const myCondition = new cc.Condition()
myCondition.setTypeId(cc.PreimageSha256.TYPE_ID)
myCondition.setBitmask(cc.PreimageSha256.FEATURE_BITMASK)
myCondition.setHash(new Buffer('e3b0c44298fc1c149afbf4c8996fb92427ae41e4649b934ca495991b7852b855', 'hex'))
myCondition.setMaxFulfillmentLength(0)
console.log(myCondition.serializeUri())
// prints 'cc:0:3:47DEQpj8HBSa-_TImW-5JCeuQeRkm5NMpJWZG3hSuFU:0'
Number
Number
Buffer
Number
String
Buffer
Boolean
Condition
Condition
Number
Number
Condition
String
Buffer
String
Buffer
Boolean
Fulfillment
Fulfillment
Boolean
Buffer
String
Buffer
String
Crypto-condition.
A primary design goal of crypto-conditions was to keep the size of conditions constant. Even a complex multi-signature can be represented by the same size condition as a simple hashlock.
However, this means that a condition only carries the absolute minimum information required. It does not tell you anything about its structure.
All that is included with a condition is the fingerprint (usually a hash of the parts of the fulfillment that are known up-front, e.g. public keys), the maximum fulfillment size, the set of features used and the condition type.
This information is just enough that an implementation can tell with certainty whether it would be able to process the corresponding fulfillment.
Kind: inner class of types
Number
Number
Buffer
Number
String
Buffer
Boolean
Condition
Condition
Number
Return the type of this condition.
The type is a unique integer ID assigned to each type of condition.
Kind: instance method of Condition
Returns: Number
- Type corresponding to this condition.
Set the type.
Sets the type ID for this condition.
Kind: instance method of Condition
Param | Type | Description |
---|---|---|
type | Number | Integer representation of type. |
Number
Return the bitmask of this condition.
For simple condition types this is simply the set of bits representing the features required by the condition type.
For structural conditions, this is the bitwise OR of the bitmasks of the condition and all its subconditions, recursively.
Kind: instance method of Condition
Returns: Number
- Bitmask required to verify this condition.
Set the bitmask.
Sets the required bitmask to validate a fulfillment for this condition.
Kind: instance method of Condition
Param | Type | Description |
---|---|---|
bitmask | Number | Integer representation of bitmask. |
Buffer
Return the hash of the condition.
A primary component of all conditions is the hash. It encodes the static properties of the condition. This method enables the conditions to be constant size, no matter how complex they actually are. The data used to generate the hash consists of all the static properties of the condition and is provided later as part of the fulfillment.
Kind: instance method of Condition
Returns: Buffer
- Hash of the condition
Validate and set the hash of this condition.
Typically conditions are generated from fulfillments and the hash is calculated automatically. However, sometimes it may be necessary to construct a condition URI from a known hash. This method enables that case.
Kind: instance method of Condition
Param | Type | Description |
---|---|---|
hash | Buffer | Hash as binary. |
Number
Return the maximum fulfillment length.
The maximum fulfillment length is the maximum allowed length for any fulfillment payload to fulfill this condition.
The condition defines a maximum fulfillment length which all implementations will enforce. This allows implementations to verify that their local maximum fulfillment size is guaranteed to accomodate any possible fulfillment for this condition.
Otherwise an attacker could craft a fulfillment which exceeds the maximum size of one implementation, but meets the maximum size of another, thereby violating the fundamental property that fulfillments are either valid everywhere or nowhere.
Kind: instance method of Condition
Returns: Number
- Maximum length (in bytes) of any fulfillment payload that
fulfills this condition..
Set the maximum fulfillment length.
The maximum fulfillment length is normally calculated automatically, when
calling Fulfillment#getCondition
. However, when
Kind: instance method of Condition
Param | Type | Description |
---|---|---|
Maximum | Number | fulfillment payload length in bytes. |
String
Generate the URI form encoding of this condition.
Turns the condition into a URI containing only URL-safe characters. This format is convenient for passing around conditions in URLs, JSON and other text-based formats.
Kind: instance method of Condition
Returns: String
- Condition as a URI
Buffer
Serialize condition to a buffer.
Encodes the condition as a string of bytes. This is used internally for encoding subconditions, but can also be used to passing around conditions in a binary protocol for instance.
Kind: instance method of Condition
Returns: Buffer
- Serialized condition
Boolean
Ensure the condition is valid according the local rules.
Checks the condition against the local bitmask (supported condition types) and the local maximum fulfillment size.
Kind: instance method of Condition
Returns: Boolean
- Whether the condition is valid according to local rules.
Condition
Create a Condition object from a URI.
This method will parse a condition URI and construct a corresponding Condition object.
Kind: static method of Condition
Returns: Condition
- Resulting object
Param | Type | Description |
---|---|---|
serializedCondition | String | URI representing the condition |
Condition
Create a Condition object from a binary blob.
This method will parse a stream of binary data and construct a corresponding Condition object.
Kind: static method of Condition
Returns: Condition
- Resulting object
Param | Type | Description |
---|---|---|
reader | Reader | Binary stream implementing the Reader interface |
Base class for fulfillment types.
Kind: inner class of types
Number
Number
Condition
String
Buffer
String
Buffer
Boolean
Fulfillment
Fulfillment
Number
Return the type ID of this fulfillment.
Kind: instance method of Fulfillment
Returns: Number
- Type ID as an integer.
Number
Return the bitmask of this fulfillment.
For simple fulfillment types this is simply the bit representing this type.
For meta-fulfillments, these are the bits representing the types of the subconditions.
Kind: instance method of Fulfillment
Returns: Number
- Bitmask corresponding to this fulfillment.
Condition
Generate condition corresponding to this fulfillment.
An important property of crypto-conditions is that the condition can always be derived from the fulfillment. This makes it very easy to post fulfillments to a system without having to specify which condition the relate to. The system can keep an index of conditions and look up any matching events related to that condition.
Kind: instance method of Fulfillment
Returns: Condition
- Condition corresponding to this fulfillment.
String
Shorthand for getting condition URI.
Stands for getCondition().serializeUri().
Kind: instance method of Fulfillment
Returns: String
- Condition URI.
Buffer
Shorthand for getting condition encoded as binary.
Stands for getCondition().serializeBinary().
Kind: instance method of Fulfillment
Returns: Buffer
- Binary encoded condition.
String
Generate the URI form encoding of this fulfillment.
Turns the fulfillment into a URI containing only URL-safe characters. This format is convenient for passing around fulfillments in URLs, JSON and other text-based formats.
Kind: instance method of Fulfillment
Returns: String
- Fulfillment as a URI
Buffer
Serialize fulfillment to a buffer.
Encodes the fulfillment as a string of bytes. This is used internally for encoding subfulfillments, but can also be used to passing around fulfillments in a binary protocol for instance.
Kind: instance method of Fulfillment
Returns: Buffer
- Serialized fulfillment
Boolean
Validate this fulfillment.
This implementation is a stub and will be overridden by the subclasses.
Kind: instance method of Fulfillment
Returns: Boolean
- Validation result
Fulfillment
Create a Fulfillment object from a URI.
This method will parse a fulfillment URI and construct a corresponding Fulfillment object.
Kind: static method of Fulfillment
Returns: Fulfillment
- Resulting object
Param | Type | Description |
---|---|---|
serializedFulfillment | String | URI representing the fulfillment |
Fulfillment
Create a Fulfillment object from a binary blob.
This method will parse a stream of binary data and construct a corresponding Fulfillment object.
Kind: static method of Fulfillment
Returns: Fulfillment
- Resulting object
Param | Type | Description |
---|---|---|
reader | Reader | Binary stream implementing the Reader interface |
ED25519: Ed25519 signature condition.
This condition implements Ed25519 signatures.
ED25519 is assigned the type ID 4. It relies only on the ED25519 feature suite which corresponds to a bitmask of 0x20.
Kind: inner class of types
Set the public publicKey.
This is the Ed25519 public key. It has to be provided as a buffer.
Kind: instance method of Ed25519
Param | Type | Description |
---|---|---|
publicKey | Buffer | Public Ed25519 publicKey |
Set the signature.
Instead of using the private key to sign using the sign() method, we can also generate the signature elsewhere and pass it in.
Kind: instance method of Ed25519
Param | Type | Description |
---|---|---|
signature | Buffer | 64-byte signature. |
Sign a message.
This method will take a message and an Ed25519 private key and store a corresponding signature in this fulfillment.
Kind: instance method of Ed25519
Param | Type | Description |
---|---|---|
message | Buffer | Message to sign. |
privateKey | String | Ed25519 private key. |
Generate the condition hash.
Since the public key is the same size as the hash we'd be putting out here, we just return the public key.
Kind: instance method of Ed25519
Param | Type | Description |
---|---|---|
hasher | Hasher | Destination where the hash payload will be written. |
Boolean
Verify the signature of this Ed25519 fulfillment.
The signature of this Ed25519 fulfillment is verified against the provided message and public key.
Kind: instance method of Ed25519
Returns: Boolean
- Whether this fulfillment is valid.
Param | Type | Description |
---|---|---|
message | Buffer | Message to validate against. |
PREFIX-SHA-256: Prefix condition using SHA-256.
A prefix condition will prepend a static prefix to the message before passing the prefixed message on to a single subcondition.
You can use prefix conditions to effectively narrow the scope of a public key or set of public keys. Simply take the condition representing the public key and place it as a subcondition in a prefix condition. Now any message passed to the subcondition will be prepended with a prefix.
Prefix conditions are especially useful in conjunction with threshold conditions. You could have a group of signers, each using a different prefix to sign a common message.
PREFIX-SHA-256 is assigned the type ID 1. It relies on the SHA-256 and PREFIX feature suites which corresponds to a feature bitmask of 0x05.
Kind: inner class of types
Set the (unfulfilled) subcondition.
Each prefix condition builds on an existing condition which is provided via this method.
Kind: instance method of PrefixSha256
Param | Type | Description |
---|---|---|
subcondition | Condition | Condition that will receive the prefixed message. |
Set the (unfulfilled) subcondition.
This will automatically parse the URI and call setSubcondition.
Kind: instance method of PrefixSha256
Param | Type | Description |
---|---|---|
Subcondition | String | URI. |
Set the (fulfilled) subcondition.
When constructing a prefix fulfillment, this method allows you to pass in a fulfillment for the condition that will receive the prefixed message.
Note that you only have to add either the subcondition or a subfulfillment, but not both.
Kind: instance method of PrefixSha256
Param | Type | Description |
---|---|---|
fulfillment | Fulfillment | Fulfillment to use for the subcondition. |
Set the (fulfilled) subcondition.
This will automatically parse the URI and call setSubfulfillment.
Kind: instance method of PrefixSha256
Param | Type | Description |
---|---|---|
Subfulfillment | String | URI. |
Set the prefix.
The prefix will be prepended to the message during validation before the message is passed on to the subcondition.
Kind: instance method of PrefixSha256
Param | Type | Description |
---|---|---|
prefix | Buffer | Prefix to apply to the message. |
Number
Get full bitmask.
This is a type of condition that contains a subcondition. A complete bitmask must contain the set of types that must be supported in order to validate this fulfillment. Therefore, we need to calculate the bitwise OR of this condition's TYPE_BIT and the subcondition's bitmask.
Kind: instance method of PrefixSha256
Returns: Number
- Complete bitmask for this fulfillment.
Boolean
Check whether this fulfillment meets all validation criteria.
This will validate the subfulfillment. The message will be prepended with the prefix before being passed to the subfulfillment's validation routine.
Kind: instance method of PrefixSha256
Returns: Boolean
- Whether this fulfillment is valid.
Param | Type | Description |
---|---|---|
message | Buffer | Message to validate against. |
PREIMAGE-SHA-256: Hashlock condition using SHA-256.
This type of condition is also called a hashlock. By creating a hash of a difficult-to-guess 256-bit random or pseudo-random integer it is possible to create a condition which the creator can trivially fulfill by publishing the random value. However, for anyone else, the condition is cryptgraphically hard to fulfill, because they would have to find a preimage for the given condition hash.
PREIMAGE-SHA-256 is assigned the type ID 0. It relies on the SHA-256 and PREIMAGE feature suites which corresponds to a feature bitmask of 0x03.
Kind: inner class of types
Provide a preimage.
The preimage is the only input to a SHA256 hashlock condition.
Note that the preimage should contain enough (pseudo-random) data in order to be difficult to guess. A sufficiently large secret seed and a cryptographically secure pseudo-random number generator (CSPRNG) can be used to avoid having to store each individual preimage.
Kind: instance method of PreimageSha256
Param | Type | Description |
---|---|---|
preimage | Buffer | Secret data that will be hashed to form the condition. |
Boolean
Validate this fulfillment.
For a SHA256 hashlock fulfillment, successful parsing implies that the fulfillment is valid, so this method is a no-op.
Kind: instance method of PreimageSha256
Returns: Boolean
- Validation result
RSA-SHA-256: RSA signature condition using SHA-256.
This RSA condition uses RSA-PSS padding with SHA-256. The salt length is set equal the digest length of 32 bytes.
The public exponent is fixed at 65537 and the public modulus must be between 128 (1017 bits) and 512 bytes (4096 bits) long.
RSA-SHA-256 is assigned the type ID 3. It relies on the SHA-256 and RSA-PSS feature suites which corresponds to a feature bitmask of 0x11.
Kind: inner class of types
Set the public modulus.
This is the modulus of the RSA public key. It has to be provided as a raw buffer with no leading zeros.
Kind: instance method of RsaSha256
Param | Type | Description |
---|---|---|
modulus | Buffer | Public RSA modulus |
Set the signature manually.
The signature must be a valid RSA-PSS siganture.
Kind: instance method of RsaSha256
Param | Type | Description |
---|---|---|
signature | Buffer | RSA signature. |
Sign the message.
This method will take the provided message and create a signature using the provided RSA private key. The resulting signature is stored in the fulfillment.
The key should be provided as a PEM encoded private key string.
The message is padded using RSA-PSS with SHA256.
Kind: instance method of RsaSha256
Param | Type | Description |
---|---|---|
message | Buffer | Message to sign. |
privateKey | String | RSA private key |
Boolean
Verify the signature of this RSA fulfillment.
The signature of this RSA fulfillment is verified against the provided message and the condition's public modulus.
Kind: instance method of RsaSha256
Returns: Boolean
- Whether this fulfillment is valid.
Param | Type | Description |
---|---|---|
message | Buffer | Message to verify. |
THRESHOLD-SHA-256: Threshold gate condition using SHA-256.
Threshold conditions can be used to create m-of-n multi-signature groups.
Threshold conditions can represent the AND operator by setting the threshold to equal the number of subconditions (n-of-n) or the OR operator by setting the thresold to one (1-of-n).
Threshold conditions allows each subcondition to carry an integer weight.
Since threshold conditions operate on conditions, they can be nested as well which allows the creation of deep threshold trees of public keys.
By using Merkle trees, threshold fulfillments do not need to to provide the structure of unfulfilled subtrees. That means only the public keys that are actually used in a fulfillment, will actually appear in the fulfillment, saving space.
One way to formally interpret threshold conditions is as a boolean weighted threshold gate. A tree of threshold conditions forms a boolean weighted threhsold circuit.
THRESHOLD-SHA-256 is assigned the type ID 2. It relies on the SHA-256 and THRESHOLD feature suites which corresponds to a feature bitmask of 0x09.
Kind: inner class of types
Add a subcondition (unfulfilled).
This can be used to generate a new threshold condition from a set of subconditions or to provide a non-fulfilled subcondition when creating a threshold fulfillment.
Kind: instance method of ThresholdSha256
Param | Type | Default | Description |
---|---|---|---|
subcondition | Condition | Condition to add | |
[weight] | Number | 1 | Integer weight of the subcondition. |
Add a subcondition (unfulfilled).
This will automatically parse the URI and call addSubcondition.
Kind: instance method of ThresholdSha256
Param | Type | Description |
---|---|---|
Subcondition | String | URI. |
Add a fulfilled subcondition.
When constructing a threshold fulfillment, this method allows you to provide a fulfillment for one of the subconditions.
Note that you do not have to add the subcondition if you're adding the fulfillment. The condition can be calculated from the fulfillment and will be added automatically.
Kind: instance method of ThresholdSha256
Param | Type | Default | Description |
---|---|---|---|
Fulfillment | Fulfillment | to add | |
[weight] | Number | 1 | Integer weight of the subcondition. |
Add a fulfilled subcondition.
This will automatically parse the URI and call addSubfulfillment.
Kind: instance method of ThresholdSha256
Param | Type | Description |
---|---|---|
Subfulfillment | String | URI. |
Set the threshold.
Determines the weighted threshold that is used to consider this condition fulfilled. If the added weight of all valid subfulfillments is greater or equal to this number, the threshold condition is considered to be fulfilled.
Kind: instance method of ThresholdSha256
Param | Type | Description |
---|---|---|
threshold | Number | Integer threshold |
Number
Get full bitmask.
This is a type of condition that can contain subconditions. A complete bitmask must contain the set of types that must be supported in order to validate this fulfillment. Therefore, we need to calculate the bitwise OR of this condition's FEATURE_BITMASK and all subcondition's and subfulfillment's bitmasks.
Kind: instance method of ThresholdSha256
Returns: Number
- Complete bitmask for this fulfillment.
Boolean
Check whether this fulfillment meets all validation criteria.
This will validate the subfulfillments and verify that there are enough subfulfillments to meet the threshold.
Kind: instance method of ThresholdSha256
Returns: Boolean
- Whether this fulfillment is valid.
Param | Type | Description |
---|---|---|
message | Buffer | Message to validate against. |
Extensible error class.
The built-in Error class is not actually a constructor, but a factory. It
doesn't operate on this
, so if we call it as super()
it doesn't do
anything useful.
Nonetheless it does create objects that are instanceof Error. In order to easily subclass error we need our own base class which mimics that behavior but with a true constructor.
Note that this code is specific to V8 (due to Error.captureStackTrace
).
Kind: inner class of util
Utility class for encoding and decoding Base64Url.
Kind: inner class of util
Buffer
String
Buffer
Convert a base64url encoded string to a Buffer.
Kind: instance method of Base64Url
Returns: Buffer
- Decoded data.
Param | Type | Description |
---|---|---|
base64urlString | String | base64url-encoded string |
String
Encode a buffer as base64url.
Kind: instance method of Base64Url
Returns: String
- base64url-encoded data.
Param | Type | Description |
---|---|---|
buffer | Buffer | Data to encode. |
Utilities for RSA-related DER/PEM encoding.
Kind: inner class of util
Buffer
String
Buffer
Create a DER field header.
Kind: instance method of Pem
Returns: Buffer
- Encoded header bytes.
Param | Type | Description |
---|---|---|
tag | Number | DER field tag. |
contentLength | Number | Length of the following content. |
String
Convert an RSA modulus to a PEM-encoded RSAPublicKey.
Encodes the public using the RSAPublicKey format given in RFC 3447, appendix C.
This function assumes that the exponent is 65537.
Kind: instance method of Pem
Returns: String
- PEM-encoded RSA public key.
Param | Type | Description |
---|---|---|
modulus | Buffer | RSA public modulus. |
FAQs
Implementation of crypto-conditions in JavaScript
The npm package five-bells-condition receives a total of 89 weekly downloads. As such, five-bells-condition popularity was classified as not popular.
We found that five-bells-condition demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.