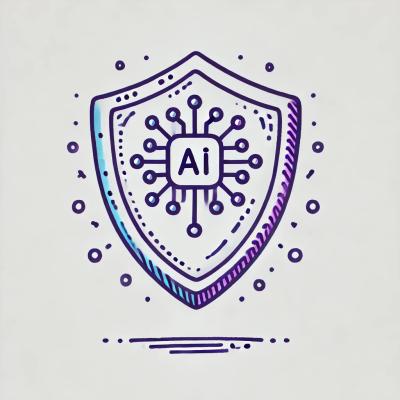
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Functional Javascript Library (inspired by Haskell's Prelude).
See desired export type below:
fjl
as a global).Note: The './dist/package/fjl*' files are for the new setup in ecma262 proposals for es6 modules ('.mjs' for es6 module and '.js' for common-js module) (these are used in package.json as the default exports).
import {...} from 'fjl';
const fjl = require('fjl');
Prelude
.fjl.is*
methods (fjl.isType
, fjl.isset
, etc.)).fjl
are curried and lib also includes versions of all methods uncurried).append
, _append
(un-curried version)map
, forEach
etc.).JSDocs [https://functional-jslib.github.io/fjl]
The docs are divided into modules though all methods in the library all live on fjl
or the top level export
of the library (the docs are written out that way to easier understand the separation and the grouping of
methods (will give users a better idea of what methods are for what (without reading to much documentation))).
Modules with a prefixed '' contain docs for un-curried members. Modules without a prefixed '' contain docs for curried members (one set of docs will be generated per module in the future).
The jsdocs link listed above has the modules included on fjl
, divided by the operation types ported over from the haskell prelude:
booleanOps
isTruthy, isFalsy, alwaysTrue, alwaysFalse
listOps
List operations imported from the Data.List
haskell module.
append, appendMany, head, last, tail, init, uncons, unconsr, concat, concatMap,
reverse, intersperse, intercalate, transpose, subsequences, subsequences1,
permutations, foldl, foldl1, foldr, foldr1, mapAccumL, mapAccumR, iterate, repeat,
replicate, cycle, unfoldr, findIndex, findIndices, elemIndex, elemIndices,
take, drop, splitAt, takeWhile, dropWhile, dropWhileEnd, span, breakOnList, at,
find, filter, map, partition, elem, notElem, lookup, isPrefixOf, isSuffixOf, isInfixOf,
isSubsequenceOf, group, groupBy, inits, tails, stripPrefix, zip, zipN, zip3,
zip4, zip5, zipWith, zipWithN, zipWith3, zipWith4, zipWith5, unzip, unzipN,
any, all, and, or, not, sum, product, maximum, minimum, scanl, scanl1, scanr,
scanr1, nub, remove, sort, sortOn, sortBy, insert, insertBy, nubBy,
removeBy, removeFirstBy, unionBy, union, intersect, intersectBy, difference,
complement
iterate
, repeat
, replicate
, cycle
In javascript we do not have lazy lists (infinite lists) like in haskell so the aforementioned methods take an integer as their first parameter (in our implementation) (since we need to know when to their internal loops); E.g.,
Javascript: take(3, iterate(a => a * 2), [1..])
Haskell: take 3 $ iterate (a -> a * 2) [1..]
Notice the [1..]
, this doesn't exist in javascript; In haskell this is an infinite lazy list
So our haskell definitions for our methods are
iterate :: (a -> a) -> [a]
repeat :: a -> [a]
replicate :: Int -> a -> [a]
cycle :: [a] -> [a]
In javascript:
repeat
and replicate
become the same:
repeat :: Int -> a -> [a]
replicate:: Int -> a -> [a]
cycle
becomes cycle :: Int -> [a] -> [a]
iterate
becomes iterate :: Int -> (a -> a) -> [a]
functionOps
The methods that comprise function operations are:
apply, call, curry, curry2, curry3, curry4, curry5, curryN,
until, flip, flip3, flip4, flip5, flipN,
negateF, negateP, negateF3, negateF4, negateF5, negateFMany,
id, compose, curry_, curry2_, curry3_, curry4_, curry5_, __ // Curry with placeholders
objectOps
These methods are not really from the haskell library but are utilities for making working with javascript objects a little bit easier.
assignDeep, assign, of, prop, typeOf, isType, instanceOf,
isFunction, isClass, isCallable,
isArray, isObject, isBoolean, isNumber, isString, isMap,
isSet, isWeakMap, isWeakSet, isUndefined, isNull, isSymbol,
isUsableImmutablePrimitive, isEmpty, isset,
isEmptyList, isEmptyObject, isEmptyCollection,
hasOwnProperty, length, keys,
objUnion, objIntersect, objDifference, objComplement,
stringOps
Import from 'Data.List' (in haskell):
lines, words, unwords, unlines
jsPlatform
Non-haskell/javascript-specific exports:
slice, includes, indexOf, lastIndexOf, split, push
Note for haskell developers:
split
in javascript is for strings.Note: Utility functions are generally not curried (minus a few exceptions:
fPureTakesOne_, fPureTakes2_, fPureTakesOneOrMore_
).
Turning regular methods into functional ones; I.e., these
take a name
and return a function that take an-argument/arguments and a type value
that has a method of name
on it.
The function returned takes arguments first and functor/member last.
fPureTakesOne, fPureTakes2, fPureTakes3, fPureTakes4, fPureTakes5,
fPureTakesOneOrMore, fPureTakesOne_, fPureTakes2_, fPureTakesOneOrMore_
Uncurried
sliceFrom, sliceTo, slice, sliceCopy
genericAscOrdering, lengths, lengthsToSmallest,
reduceUntil, reduceRightUntil, reduce, reduceRight,
lastIndex, findIndexWhere, findIndicesWhere, findWhere,
aggregateStr, aggregateArr, aggregateObj, aggregateByType,
Note:
lastIndex
gives you the last index of a list.Jsdocs here: https://functional-jslib.github.io/fjl/
See scripts
field in package.json
.
Note about 'pre-publish' script task: 'pre-publish' wasn't confused with default 'prepublish' task. 'pre-publish' task is just for getting conceptual pre-publish functionality locally (on dev-machine) without npm's default 'prepublish' side-effects/functionality (our 'pre-publish' doesn't get triggered by 'travis-ci' and the like since it isn't formally used for pre-publish on these platforms, etc..).
Unit tests are grouped by exported module: 'tests/test-listOps.js' - Tests 'listOps' module etc.
We are using 'chai' and 'mocha' though we may want to move to 'jest' in the future.
subsequences
: https://jsperf.com/subsequences/6FAQs
Functional Javascript Library.
The npm package fjl receives a total of 77 weekly downloads. As such, fjl popularity was classified as not popular.
We found that fjl demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.