Admin panel for FounderLab apps
This module will auto generate a full admin site to manage a backend based on Frameworkstein models. You provide the models you want to manage for and it will generate routes and form pages for them.
Screenshots
Homepage
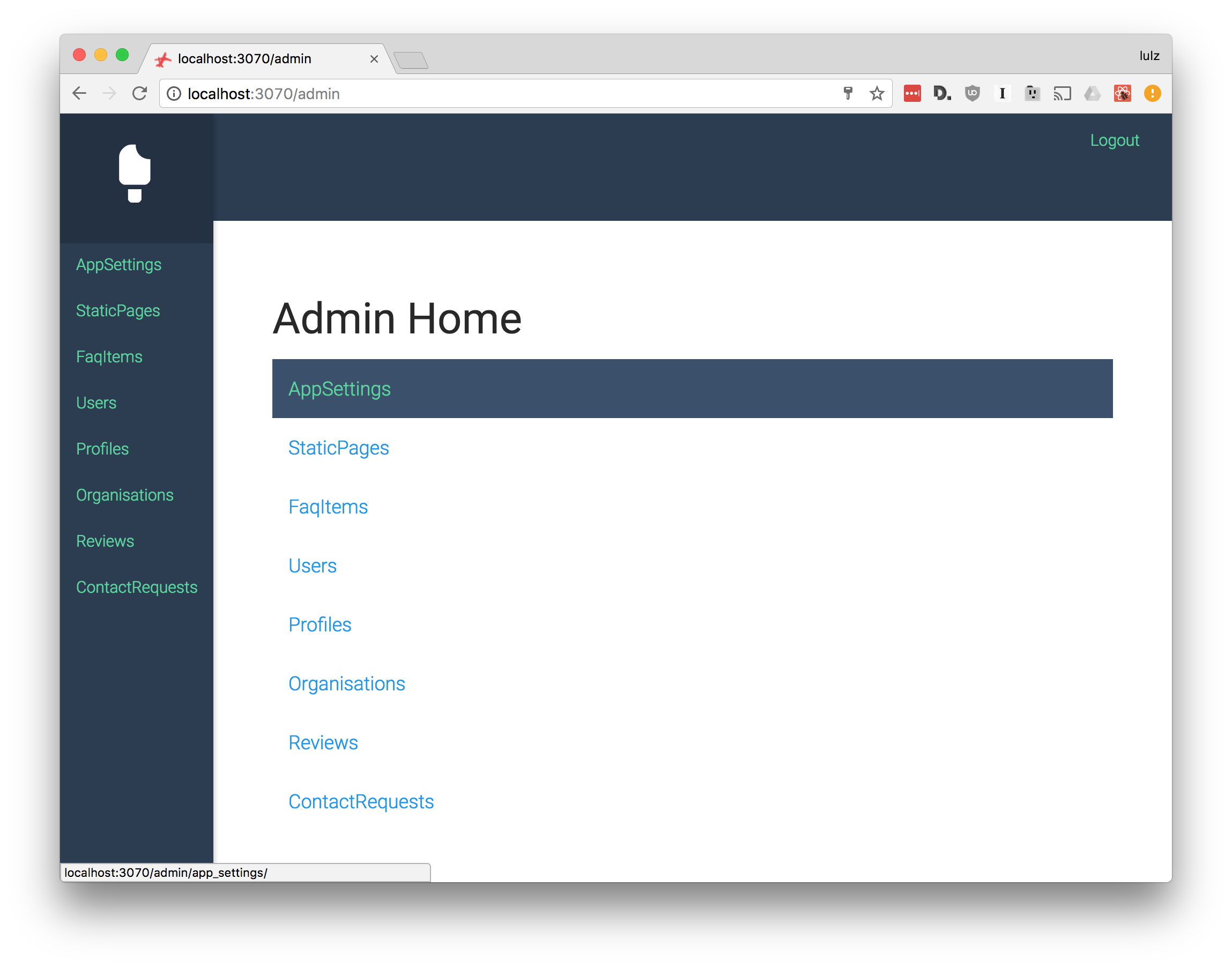
Model list
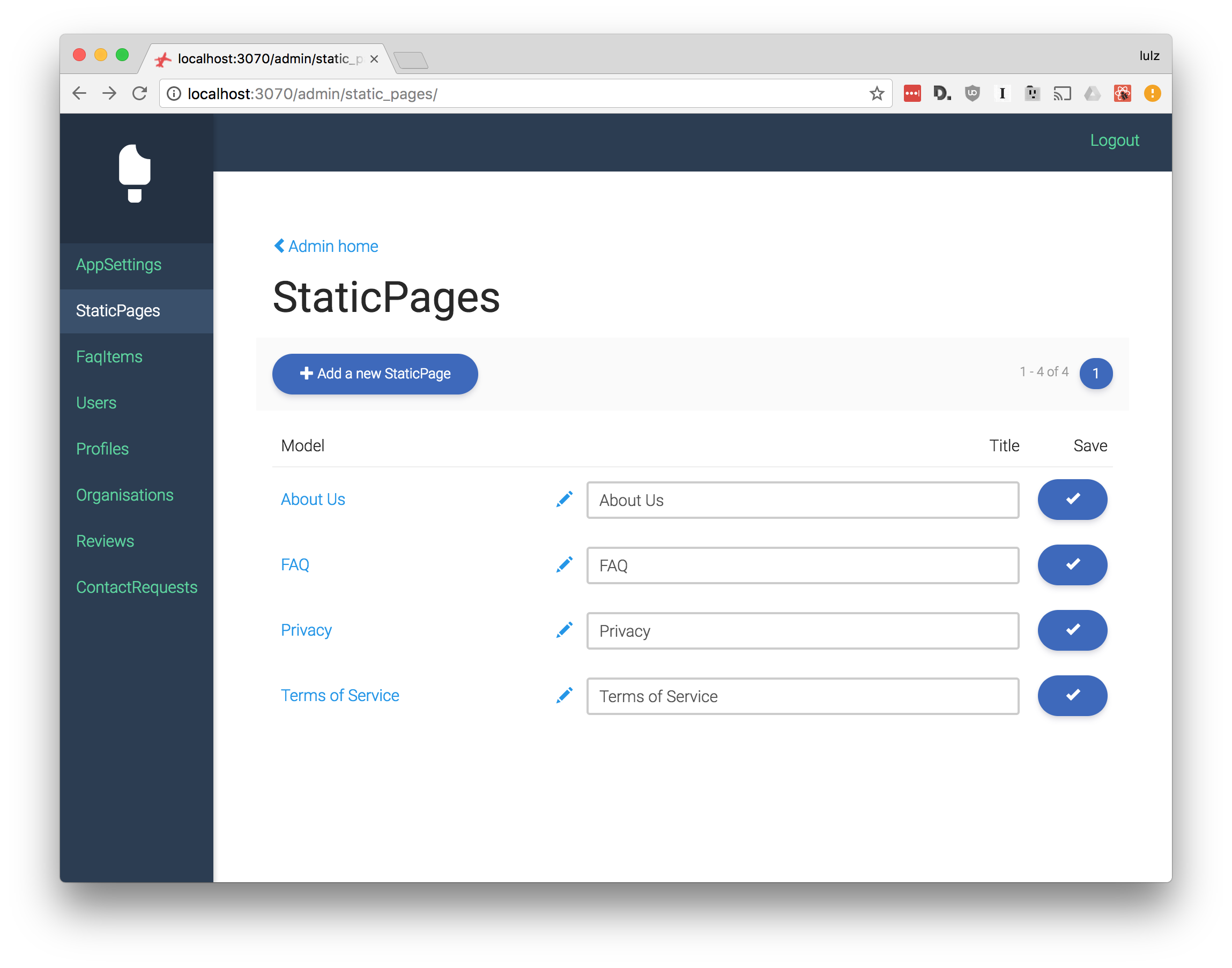
Model detail
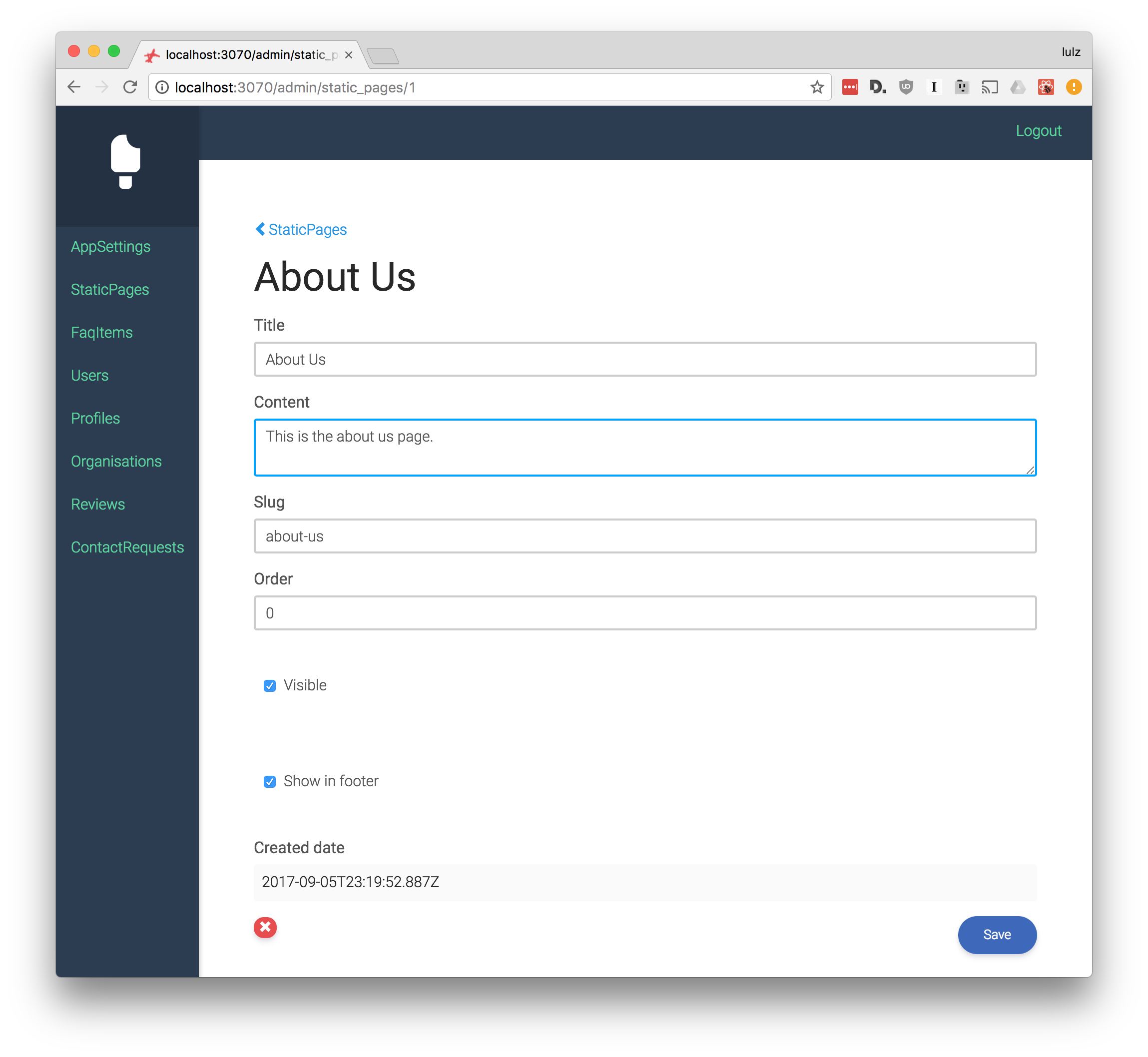
How it works
You call configureAdmin
and configure the admin with a list of models. It examines each models fields via its schema and generates form fields to edit them. You can pass in some options to control how these form fields are rendered.
Example
import configureAdmin from 'fl-admin'
import StaticPage from './models/StaticPage'
import User from './models/User'
configureAdmin({
models: [
{
Model: User,
display: model => model.email,
fields: {
email: {
listDisplay: true,
},
admin: {
listDisplay: true,
},
},
},
{
Model: Post,
fields: {
title: {
listEdit: true,
},
content: {
input: 'textarea',
},
},
},
],
})
Configuration
There are model-level and field-level configuration options. Models given to configuration functions are plain javascript objects (not instances of the model class).
Model configuration options
Values below are the defaults.
{
Model: null,
display: model.name || model.title,
name: Model.modelName || Model.model_name || Model.name,
sort: 'id',
perPage: 50,
listDelete: false,
rootPath: options.rootPath,
path: table(Model),
plural: plural(Model),
actionType: `${ACTION_PREFIX}${upper(Model)}`,
readOnlyFields: ['createdDate'],
ListComponent,
CreateComponent,
DetailComponent,
}
Field configuration options
Values below are the defaults.
{
label: label(key),
InputComponent: SmartInput,
input: 'text',
listDisplay: false,
listEdit: false,
readOnly: false,
hidden: false,
}