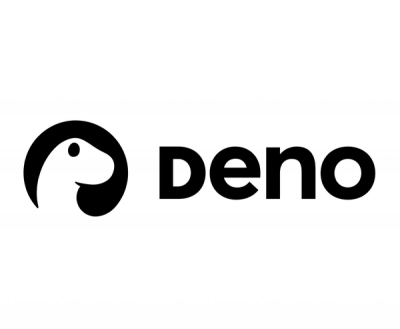
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
flacon is a dependency injection container with a clean and tiny API. It helps you to test your components individually.
npm install flacon
Imagine you have a service foo.js
.
module.exports = {
value: function () { return 'foo' }
};
Now you want another service bar.js
that uses foo.js
.
var foo = require('./foo');
module.exports = {
value: function () { return foo.value() + 'bar' }
};
This looks all good. But when testing bar.js
, mocking foo.js
is really difficult because it is a private dependency. flacon, on the other hand, forces you to explicitly declare all dependencies, making it easy to mock them.
First, we create a new container in container.js
. On a container, you can publish and load modules.
var Flacon = require('flacon');
module.exports = new Flacon();
Let's start with foo.js
. We call the publish
method with an id and a factory function.
var container = require('./container');
container.publish('foo', function () {
return {
value: function () { return 'foo' }
};
});
Moving on to bar.js
, we define foo
as a dependency. The result of foo
's factory will be passed into bar
's factory.
var container = require('./container');
container.publish('bar', ['foo'], function (foo) {
return {
value: function () { return foo.value() + 'bar' }
};
});
By simply calling the container with a module id, you will get the return value of the factory function.
var container = require('./container');
var bar = container('bar');
bar.value(); // -> 'foobar'
During testing, we can easily manipulate or mock a dependency. This will load every mocked module without caching.
var container = require('./container');
var bar = container('bar', {
foo: function (foo) {
foo.value = function () { return 'baz' };
return foo;
}
});
bar.value(); // -> 'bazbar'
flush
To force flacon to call a module's factory again, use flush
.
container.load('foo'); // factory creates module
container.flush('foo');
container.load('foo'); // factory creates module again
flacon(id, [mocks])
Loads a module by id
. Caches and returns the module.
mocks
is an object of mocking functions by id. Mocked dependencies will not be cached.
id
: The identifier, unique to the container.mocks
: A map of callbacks, mapped by module id
. The return value of each callback will be the mock.flacon.publish(id, [deps], factory)
Registers a module by id
. Returns the module's factory
.
id
: The identifier, unique to the container.deps
: An optional array of dependency id
s. Their corresponding modules will be passed into factory
.factory
: A function, taking the dependencies, that returns the module.flacon.flush()
Removes a module from the cache. Returns the container.
id
: The identifier, unique to the container.If you have a question, found a bug or want to propose a feature, have a look at the issues page.
FAQs
A hyperminimal dependency injection framework.
We found that flacon demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.