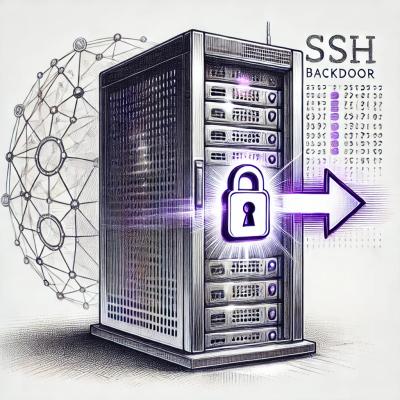
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Feature flipping (also called feature toggling) is a technique used in Continuous Deployment to allow developers to work on new features without blocking releases to production. 99 Designs explain it well.
There's also another implementation of feature flipping for node.js.
var flipper = require('flipper');
flipper.add('mutatedDolphins');
var flipper = require('flipper');
if (flipper.mutatedDolphins) {
/* dolphins are now mutated */
} else {
/* normal dolphins */
}
/* alternatively */
flipper.isEnabled('mutatedDolphins')
flipper.mutatedDolphins = true;
flipper.enable('mutatedDolphins');
flipper.mutatedDolphins = false;
flipper.disable('mutatedDolphins');
flipper.allFeatures() /* returns array of { "feature name": status } */
If you make use of the connect middleware:
var app = connect().use(flipper.http('/flipper')).listen(3000);
You can flip and inspect features via HTTP:
curl http://localhost:3000/flipper/ # returns all features with status (true/false)
curl http://localhost:3000/flipper/mutatedDolphins # returns status (true/false)
curl -X PUT http://localhost:3000/flipper/mutatedDolphins --data-binary true #enables the feature
curl -X PUT http://localhost:3000/flipper/mutatedDolphins --data-binary false
To survive application restarts, flipper can write a configuration file whenever a feature is added, enabled, or disabled. To enable this, call the persist function:
var flipper = require('flipper');
flipper.persist(__dirname + '/features.json');
FAQs
Mobile development tool
The npm package flipper receives a total of 59 weekly downloads. As such, flipper popularity was classified as not popular.
We found that flipper demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.