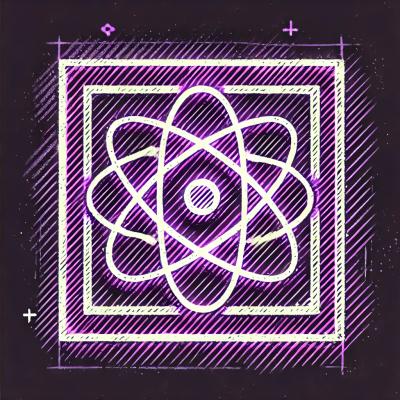
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
The modular functional reactive programming library in JavaScript.
Functional reactive programming is a powerful programming paradigm for expressing values that change over time. But existing libraries for JavaScript are huge, complex and have a high learning curve.
Flyd is different. It is simple, expressive and powerful. It is fast to learn and easy to use. It has a minimal core on top of which new abstractions can be built modularly.
Flyd gives you streams as the building block for creating reactive dataflows.
The function stream
creates a representation of a value that changes over time.
A stream is a function and at first sight it works a bit like a getter-setter:
// Create a stream with initial value 5.
var number = stream(5);
// Get the current value of the stream.
number(); // returns 5
// Update the value of the stream.
number(7); // returns 7
// The stream now returns the new value.
number(); // returns 7
Top level streams, that is streams without dependencies, should typically depend on the external world, like user input or fetched data.
Since streams are just functions you can easily plug them in whenever a function is expected.
var clicks = stream();
document.getElementById('button').addEventListener('click', clicks);
var messages = stream();
webSocket.onmessage = messages;
Clicks events will now flow down the clicks
stream and WebSockets messages
down the messages
stream.
Streams can depend on other streams. Instead of calling stream
with a value
as in the above examples we can pass it a function. The function should calculate a
value based on other streams which forms a new stream. Flyd automatically
collects the streams dependencies and updates it whenever a dependency changes.
This means that the sum
function below will be called whenever x
and y
changes.
You can think of dependent stream as streams that automatically
listens/subscribes to their dependencies.
// Create two streams of numbers
var x = stream(4);
var y = stream(6);
// Create a stream that depends on the two previous streams
// and with its value given by the two added together.
var sum = stream(function() {
return x() + y();
});
// `sum` is automatically recalculated whenever the streams it depends on changes.
x(12);
sum(); // returns 18
y(8);
sum(); // returns 20
Naturally, a stream with dependencies can depend on other streams with dependencies.
// Create two streams of numbers
var x = stream(4);
var y = stream(6);
var squareX = stream(function() {
return x() * x();
});
var squareXPlusY(function() {
return y() + doubleX();
});
squareXPlysY(); // returns 22
x(2);
squareXPlysY(); // returns 10
The body of a dependent stream is called with two streams: itself and the last changed stream on which it depends.
// Create two streams of numbers
var x = stream(1);
var y = stream(2);
var sum = stream(function(sum, changed) {
// The stream can read from itself
console.log('Last sum was ' + sum());
if (changed) { // On the initial call no stream changed
var changedName = (changed === y ? 'y' : 'x');
console.log(changedName + ' changed to ' + changed());
}
return x() + y();
});
Instead of returning a value a stream can update itself by calling itself. This is handy when working with APIs that takes callbacks.
var urls = stream('/something.json');
var responses = stream(function(resp) {
makeRequest(urls(), resp);
});
stream([responses], function() {
console.log('Recieved response!');
console.log(responses());
});
The stream above that logs the responses from the server should only be called
after an actual response has been recieved (otherwise responses()
whould return
undefined
). For this purpose you can pass stream
an array of initial
dependencies. The streams body will not be called before all of the declared
streams evaluate to something other than undefined
.
Flyd has inbuilt support for promises. Similairly to how a promise can never be resolved with a promise, a promise can never flow down a stream. Instead the fulfilled value of the promise will be sent down the stream.
var urls = stream('/something.json');
var responses = stream(function() {
return requestPromise(urls());
});
stream([responses], function() {
console.log('Recieved response!');
console.log(responses());
});
You've now seen the basic building block which Flyd provides. Let's see what we
can do with it. Lets write a function that takes a stream and a function and
returns a new stream with the functin applied to every value emitted by the
stream. In short, a map
function.
var mapStream = functin(s, f) {
return stream([s], function() {
return f(s());
});
};
We simply create a new stream dependent on the first stream. We declare the stream as a dependency so that our stream wont return values before the original stream produces its first value.
Flyd includes a map function as part of its core.
Lets try something else, reducing a stream! It could look like this:
var reduceStream = function(f, acc, s) {
return stream([s], function() {
acc = f(acc, s());
return acc;
});
};
Our reduce function takes a reducer function, in initial value and a stream. Every time the original stream emit a value we pass it to the reducer along with the accumulator.
Flyd includes a reduce function as part of its core.
You're done. Check out the API and/or the examples.
Creates a new stream.
Arguments
dependencies
] (array) – The streams on which this stream should initially depend.body
(function|*) – The function body of the stream or it initial value.staticDependencies
] – Disables automatic dependency resolution of the stream.Returns
The created stream.
###flyd.map(fn, s)
Returns a new stream consisting of every value from s
passed through fn. I.e.
mapcreates a new stream that listens to
sand applies
fn` to every new value.
Example
var numbers = stream(0);
var squaredNumbers = flyd.map(function(n) { return n*n; }, numbers);
###flyd.reduce(fn, acc, stream)
Creates a new stream with the results of calling the function on every incoming stream with and accumulator and the incoming value.
Example
var numbers = stream();
var sum = flyd.reduce(function(sum, n) { return sum+n; }, 0, numbers);
numbers(2)(3)(5);
sum(); // 10
###flyd.merge(stream1, stream2)
Creates a new stream down which all values from both stream1
and stream2
will be sent.
Example
var btn1Clicks = stream();
button1Elm.addEventListener(clicks);
var btn2Clicks = stream();
button2Elm.addEventListener(clicks);
var allClicks = flyd.merge(btn1Clicks, btn2Clicks);
Creates a new stream resulting from applying transducer
to stream
.
Example
var t = require('transducers.js');
var results = [];
var s1 = stream();
var tx = t.compose(
t.map(function(x) { return x * 2; }),
t.dedupe()
);
var s2 = flyd.transduce(tx, s1);
stream([s2], function() { results.push(s2()); });
s1(1)(1)(2)(3)(3)(3)(4);
result; // [2, 4, 6, 8]
###flyd.destroy(stream)
If the stream has no dependencies this will detach it from any streams it depends on. This makes it available for garbage collection if there are no additional references to it.
###stream()
Returns the last value of the stream.
Example
var names = stream('Turing');
names(); // 'Turing'
names('Bohr');
names(); // 'Bohr'
###stream(val)
Pushes a value down the stream.
###stream.map(f)
Returns a new stream identical to the original exept every
value will be passed through f
.
###stream1.ap(stream2)
stream1
must be a stream of functions.
Returns a new stream which is the result of applying the
functions from stream1
to the values in stream2
.
###stream.of(value)
Returns a new stream with value
as its initial value.
FAQs
The less is more, modular, functional reactive programming library
The npm package flyd receives a total of 681 weekly downloads. As such, flyd popularity was classified as not popular.
We found that flyd demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.