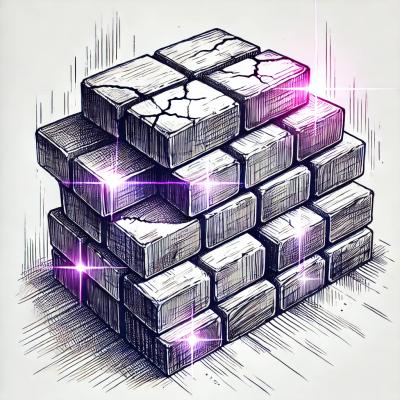
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
node and browser connection for FileMaker Server
###Status: 2 - unstable The api is under development and may change. Backwards compatibility will be maintained if feasible. See node stability ratings
###Installation fms-js can be installed using npm
npm install fms-js
###Usage Once installed you can require the fms object, and start to use it
var fms = require('fms-js);
// create a connection object
var connection = fms.connection({
url : '<url>',
userName : 'username',
password : 'password
});
//use the connection object to create requests
var listDBNamesRequest = connection.dbnames();
//and send it to FileMaker Server
//all request are asynchronous.
//Pass the callback to the 'send()' method
listDBNamesRequest.send(callback)
The API is chainable. So you can do some fun expressive things. The following will find the first 10 contacts who have the firstName 'todd"
connection
.db('Contacts')
.layout('contacts')
.find({
'firstName' : 'todd'
})
.set('-max', 10)
.send(callback)
You can keep a reference to any point in the chain. So this is the equivilent of the above
var db = connection.db('Contacts');
var layout = db.layout('contacts');
var findRequest = layout.find({
'firstName' : 'todd'
})
var findRequestFirstTen = findRequest.set('-max', 10)
findRequestFirstTen.send(callback)
FAQs
FileMaker Server Connection for Node and the browser
The npm package fms-js receives a total of 4 weekly downloads. As such, fms-js popularity was classified as not popular.
We found that fms-js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.