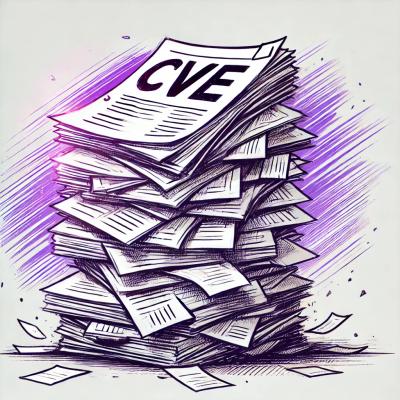
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
for-emit-of
Advanced tools
Turn Node.js Events into Async Iterables.
$ npm install for-emit-of
import forEmitOf from 'for-emit-of';
import { Emitter } from '..'; // Example
const iterator = forEmitOf(Emitter, {
event: "data", // Default
});
for await (const event of iterator){
// Do Something
}
Equivalent to
Emitter.on("data", () => {});
import forEmitOf from 'for-emit-of';
import { Emitter } from '..';
const iterator = forEmitOf(Emitter, {
transform: async (event) => { // async aware
return JSON.stringify(event);
}
});
for await (const event of iterator){
// Stringy
}
Equivalent to
Emitter.on("data", (event) => {
const stringy = JSON.stringify(event);
});
import forEmitOf from 'for-emit-of';
import { Cart } from '..';
const iterator = forEmitOf(Cart, {
event: "checkout"
});
for await (const order of iterator){
// Do Something
}
Equivalent to
Cart.on("checkout", (order) => { ... });
import forEmitOf from 'for-emit-of';
import { Cart } from '..';
const iterator = forEmitOf(Cart, {
end: ["end", "close"] // default
});
firstEventTimeout
import forEmitOf from 'for-emit-of';
import { EventEmitter } from "events";
const emitter = new EventEmitter();
const iterator = forEmitOf(emitter, {
firstEventTimeout: 1000,
});
setTimeout(() => {
emitter.emit("data", {});
}, 2000); // greater than firstEventTimeout ERROR!
for await (const msg of iterator) {
console.log(msg); // never get here
}
inBetweenTimeout
import forEmitOf from 'for-emit-of';
import { EventEmitter } from "events";
const emitter = new EventEmitter();
const iterator = forEmitOf(emitter, {
inBetweenTimeout: 1000,
});
setInterval(() => {
emitter.emit("data", {})
}, 2000) // greater than inBetweenTimeout ERROR!
for await (const msg of iterator) {
console.log(msg); // gets here once
}
import forEmitOf from 'for-emit-of';
import { EventEmitter } from "events";
const emitter = new EventEmitter();
const iterator = forEmitOf(emitter, {
limit: 10
});
const interval = setInterval(() => {
emitter.emit("data", {});
}, 100);
let msgCount = 0;
for await (const msg of iterator) {
msgCount += 1
}
clearInterval(interval);
console.log(msgCount); // 10
import forEmitOf from 'for-emit-of';
import { EventEmitter } from "events";
const emitter = new EventEmitter();
const iterator = forEmitOf(emitter, {
limit: 10,
debug: true // logs
});
import forEmitOf from 'for-emit-of';
import { EventEmitter } from "events";
const neverEmit = new EventEmitter();
const iterator = forEmitOf(neverEmit, {
keepAlive: 1000
});
for await (const data of iterator){
// waiting ⌛
}
FAQs
Turn Node.js Events into Async Iterables
The npm package for-emit-of receives a total of 215 weekly downloads. As such, for-emit-of popularity was classified as not popular.
We found that for-emit-of demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.