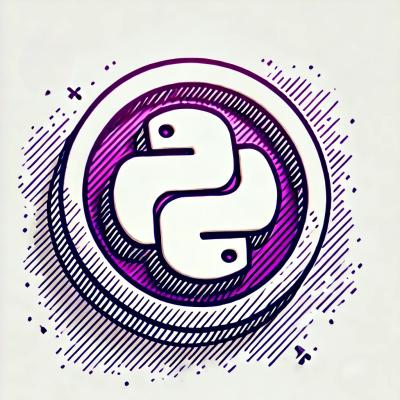
Security News
Python Overtakes JavaScript as Top Programming Language on GitHub
Python becomes GitHub's top language in 2024, driven by AI and data science projects, while AI-powered security tools are gaining adoption.
form-data-encoder
Advanced tools
The form-data-encoder package is designed to encode FormData objects into a variety of formats suitable for HTTP transmission. It is particularly useful for preparing multipart/form-data payloads without relying on the browser's native FormData implementation, which can be beneficial in non-browser environments like Node.js.
Encoding FormData for multipart/form-data
This feature allows you to encode a FormData object into a multipart/form-data format, which is suitable for HTTP requests. The code sample demonstrates how to create a FormData object, append fields and files, and then use the FormDataEncoder to encode the data and retrieve the necessary headers for an HTTP request.
const { FormDataEncoder } = require('form-data-encoder');
const { FormData, File } = require('formdata-node');
const formData = new FormData();
formData.append('field', 'value');
formData.append('file', new File(['content'], 'file.txt'));
const encoder = new FormDataEncoder(formData);
// You can now use encoder to get headers and read the encoded form data
const headers = encoder.headers;
const body = Readable.from(encoder);
Streaming encoded data
This feature is useful for streaming the encoded form data, which can be helpful when dealing with large files or when you want to send the data in chunks over a network. The code sample shows how to iterate over the encoder to handle each chunk of data.
const { FormDataEncoder } = require('form-data-encoder');
const { FormData } = require('formdata-node');
const formData = new FormData();
formData.append('field', 'value');
const encoder = new FormDataEncoder(formData);
// Stream the encoded form data
for await (const chunk of encoder) {
// Handle each chunk of data
}
The form-data package is a Node.js module that allows you to create readable 'multipart/form-data' streams. It can be used to submit forms and file uploads to other web applications. It is similar to form-data-encoder but also provides the ability to append streams, buffers, and simple values to form data.
Busboy is a Node.js module for parsing incoming HTML form data. It handles multipart/form-data, which is primarily used for uploading files. While form-data-encoder is focused on encoding and preparing form data for transmission, busboy is designed to parse and handle form data received by a server.
Multiparty is a Node.js module for parsing multipart/form-data requests, which are typically used for file uploads. Unlike form-data-encoder, which is for encoding form data, multiparty is used on the server side to process the uploaded data.
Encode FormData
content into the multipart/form-data
format
import {Readable} from "stream"
import {FormData, File} from "formdata-node"
import {Encoder} from "form-data-encoder"
import fetch from "node-fetch"
const fd = new FormData()
fd.set("greeting", "Hello, World!")
fd.set("file", new File(["On Soviet Moon landscape see binoculars through YOU"], "file.txt"))
const encoder = new Encoder(fd)
const options = {
method: "post",
// Set request headers provided by the Encoder.
// The `headers` property has `Content-Type` and `Content-Length` headers.
headers: encoder.headers,
// Create a Readable stream from the Encoder.
// You can omit usage of `Readable.from` for HTTP clients whose support async iterables.
// The Encoder will yield FormData content portions encoded into the multipart/form-data format as node-fetch consumes the stream.
body: Readable.from(encoder.encode()) // or Readable.from(encoder)
}
const response = await fetch("https://httpbin.org/post", options)
console.log(await response.json())
formdata-polyfill
:import {Readable} from "stream"
import {Encoder} from "form-data-encoder"
import {FormData, File} from "formdata-polyfill/esm-min.js"
const fd = new FormData()
fd.set("field", "Some value")
fd.set("file", new File(["File content goes here"], "file.txt"))
const encoder = new Encoder(fd)
const options = {
method: "post",
headers: encoder.headers,
body: Readable.from(encoder)
}
await fetch("https://httpbin.org/post", options)
Blob
, for that you can write a function like this:import {FormData} from "formdata-polyfill/esm-min.js"
import {blobFrom} from "fetch-blob/from.js"
import {Encoder} from "form-data-encoder"
import Blob from "fetch-blob"
import fetch from "node-fetch"
async function toBlob(form) {
const encoder = new Encoder(form)
const chunks = []
for await (const chunk of encoder) {
chunks.push(chunk)
}
return new Blob(chunks, {type: encoder.contentType})
}
const fd = new FormData()
fd.set("name", "John Doe")
fd.set("avatar", await blobFrom("path/to/an/avatar.png"), "avatar.png")
const options = {
method: "post",
body: await toBlob(fd)
}
await fetch("https://httpbin.org/post", options)
form-data-encoder
is making a Blob-ish class:import {Readable} from "stream"
import {FormData} from "formdata-polyfill/esm-min.js"
import {blobFrom} from "fetch-blob/from.js"
import {Encoder} from "form-data-encoder"
import Blob from "fetch-blob"
import fetch from "node-fetch"
class BlobDataItem {
constructor(encoder) {
this.#encoder = encoder
this.#size = encoder.headers["Content-Length"]
this.#type = encoder.headers["Content-Type"]
}
get type() {
return this.#type
}
get size() {
return this.#size
}
stream() {
return Readable.from(this.#encoder)
}
get [Symbol.toStringTag]() {
return "Blob"
}
}
const fd = new FormData()
fd.set("name", "John Doe")
fd.set("avatar", await blobFrom("path/to/an/avatar.png"), "avatar.png")
const encoder = new Encoder(fd)
// Note that node-fetch@2 performs more strictness tests for Blob objects, so you may need to do extra steps before you set up request body (like, maybe you'll need to instaniate a Blob with BlobDataItem as one of its blobPart)
const blob = new BlobDataItem(enocoder) // or new Blob([new BlobDataItem(enocoder)], {type: encoder.contentType})
const options = {
method: "post",
body: blob
}
await fetch("https://httpbin.org/post", options)
// This module is only necessary when you targeting Node.js or need web streams that implement Symbol.asyncIterator
import {ReadableStream} from "web-streams-polyfill/ponyfill/es2018"
import {Encoder} from "form-data-encoder"
import {FormData} from "formdata-node"
import fetch from "node-fetch"
const toReadableStream = iterator => new ReadableStream({
async pull(controller) {
const {value, done} = await iterator.next()
if (done) {
return controller.close()
}
controller.enqueue(value)
}
})
const fd = new FormData()
fd.set("field", "My hovercraft is full of eels")
const encoder = new Encoder(fd)
const options = {
method: "post",
headers: encoder.headers,
body: toReadableStream(encoder.encode())
}
// Note that this example requires `fetch` to support Symbol.asyncIterator, which node-fetch lacks of (but will support eventually)
await fetch("https://httpbin.org/post", options)
import {Encoder} from "form-data-encoder"
import {FormData} from "formdata-node"
import fetch from "node-fetch"
const fd = new FormData()
fd.set("field", "My hovercraft is full of eels")
const encoder = new Encoder(fd)
const options = {
method: "post",
headers: encoder.headers,
body: encoder
}
await fetch("https://httpbin.org/post", options)
import {FormData} from "formdata-node" // Or any other spec-compatible implementation
import fetch from "node-fetch"
const fd = new FormData()
fd.set("field", "My hovercraft is full of eels")
const options = {
method: "post",
body: fd
}
// Note that node-fetch does NOT support form-data-encoder
await fetch("https://httpbin.org/post", options)
You can install this package using npm:
npm install form-data-encoder
Or yarn:
yarn add form-data-encoder
Or pnpm:
pnpm add form-data-encoder
class Encoder
constructor(form[, boundary]) -> {Encoder}
Creates a multipart/form-data encoder.
boundary -> {string}
Returns boundary string
contentType -> {string}
Returns Content-Type header for multipart/form-data
headers -> {object}
Returns headers object with Content-Type and Content-Length header
encode() -> {AsyncGenerator<Uint8Array, void, undefined>}
Creates an async iterator allowing to perform the encoding by portions.
[Symbol.asyncIterator]() -> {AsyncGenerator<Uint8Array, void, undefined>}
An alias for Encoder#encode()
method.
isFileLike(value) -> {boolean}
Check if given value is a File-ish object.
isFormDataLike(value) -> {boolean}
Check if is a FormData-ish object.
FAQs
Encode FormData content into the multipart/form-data format
The npm package form-data-encoder receives a total of 4,935,525 weekly downloads. As such, form-data-encoder popularity was classified as popular.
We found that form-data-encoder demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Python becomes GitHub's top language in 2024, driven by AI and data science projects, while AI-powered security tools are gaining adoption.
Security News
Dutch National Police and FBI dismantle Redline and Meta infostealer malware-as-a-service operations in Operation Magnus, seizing servers and source code.
Research
Security News
Socket is tracking a new trend where malicious actors are now exploiting the popularity of LLM research to spread malware through seemingly useful open source packages.