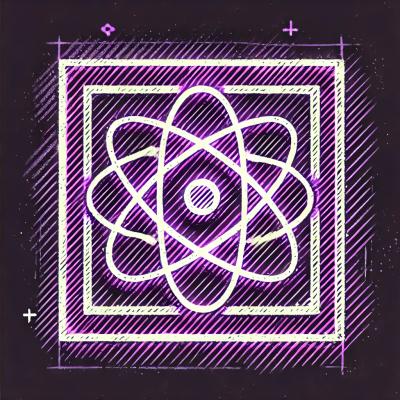
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
format-gpt
Advanced tools
FormatGPT is a robust library designed to sanitize output provided by ChatGPT. It provides a consistent and well defined methods for retrieving the data from ChatGPT by acting as a middle man between your code and ChatGPT API. It supports various formats such as:
Additionally each output format can be easily customized to suit your needs.
To install the library using npm
run the command:
npm install format-gpt
To install the library using yarn
run the command:
yarn add format-gpt
Here is quick example on how to format prompts for chat-gpt
:
import {formatGptPrompt} from "format-gpt";
formatGpt.format(prompt, {
format: "json-list",
language: "en",
options: {
attributes: [
{name: "name", type: "string"},
{name: "description", type: "string", maxLength: 200},
{name: "released", type: "date"},
{name: "price", type: "decimal"},
],
},
});
And another example on how communicate with chat-gpt
through provided wrapper:
import FormatGPT from "format-gpt";
import {Configuration, OpenAIApi} from "openai";
// Create OpenAIApi instance
const configuration = new Configuration({
organization: "YOUR_ORGANIZATION_ID",
apiKey: "YOUR_API_KEY",
});
const openai = new OpenAIApi(configuration);
// Initialize GptFormatter providing OpenAIApi instance
const formatter = new GptFormatter(openai);
// Use provided FormatGPT wrapper methods instead of those from OpenAIApi.
// Below is an example using `createChatCompletion` method.
const result = await formatter.createChatCompletion(request, options, output);
Parameters request
and options
are the same ones as passed to createChatCompletion
method from openai
library.
Parameter output
is where the magic happens.
You define how you would like the output to be structured and under the hood FormatGPT transforms your requests and prompts to achieve desired result.
The default export is formatChatGptPrompt
.
formatGptPrompt(prompt: string, output: IOutputConfig): string;
prompt (string)
- content of the prompt for chat-gptoutput (IOutputConfig)
format (Format | string)
- format of data retrieved from chat-gpt
(described below)language (string)
- language code (determines language of the output, i.e. "en"
, "de"
)size (number)
- expected tokens count (used for reporting progress when streaming)options: (IFormatOptions)
attributes: (IAttribute[])
- array with attribute definitionscolumnSeparator (string)
- column separator (for CSV format, default: ","
)rowSeparator (string)
- row separator (for CSV format. default: \n
)formatGptMessages(messages: ChatCompletionRequestMessage[], output: IOutputConfig): ChatCompletionRequestMessage[];
messages (ChatCompletionRequestMessage[])
- messages sent to chat-gpt apioutput (IOutputConfig)
- output configurationformatGptRequest(request: CreateChatCompletionRequest, output: IOutputConfig): CreateChatCompletionRequest;
request (CreateChatCompletionRequest)
- request config for chat-gpt apioutput (IOutputConfig)
- output configurationtype Format = "string"; // You can use one of the predefined formats
Available predefined formats:
text
- plain text stringarray
- javascript array (["item1", "item2", "item3"]
)csv
- text in CSV format (configure column and line separator with IOutputConfig
)html
- string containing htmlhtml-table
- string containing table in html formatmarkdown
- string containing markdownmarkdown-table
- string containing table in markdown formatjson
- JSON objectjson-list
- list of JSON objects (e.g. [{ a: "A", b: "B"}, { a: "A", b: "B"}, ...]
)xml
- string containing xmlyaml
- string containing yamlinterface IAttribute {
name: string;
type: "string" | "number" | "boolean" | "date" | "integer" | "decimal" | IAttribute[];
minLength?: number;
maxLength?: number;
custom?: string;
}
name
- display name of the attribute in output datatype
- simple type or an array of attributes (IAttribute[]
), also try experimenting with other typesminLength
- minimum length of the attribute (applicable to text)maxLength
- maximum length of the attribute (applicable to text)custom
- additional attrribute description (e.g. "uppercase"
, "truncate to 2 decimal places"
)For more information on ChatCompletionRequestMessage
and CreateChatCompletionRequest
please refer OpenAI documentation.
To build format-gpt follow these steps:
npm install
or yarn install
commandyarn
with Visual Studio Code also run yarn dlx @yarnpkg/sdks vscode
npm build
or yarn build
commandTo run the test suite, first install the dependencies, then execute tests.
Using npm
:
npm install
npm test
Using yarn
:
yarn install
yarn test
This project is licensed under the MIT License.
FAQs
ChatGPT output formatter.
We found that format-gpt demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.