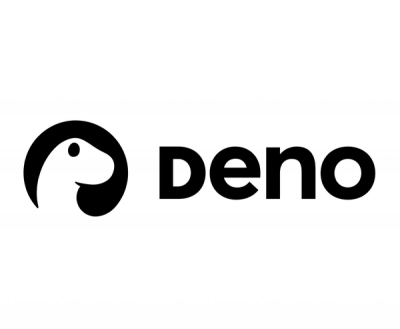
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
froala-syntax-highlighter
Advanced tools
Syntax highlighter for Froala with auto detection programming language
Syntax highlighter tool based on Shiki with programming language detection in the Froala editor. This package is compatible with Froala editor versions 3 and 4.
Install the npm module:
npm install froala-syntax-highlighter
Load the module into your project:
<script src="node_modules/froala-syntax-highlighter/lib/index.js"></script>
Update Froala configuration:
Add Syntax Highlighter button to the toolbar:
${}.froalaEditor({
// Enable the plugin.
pluginsEnabled: ['syntaxHighlighter'],
// Add the buttons to the toolbar
toolbarButtons: ['highlightCode'],
toolbarButtonsMD: ['highlightCode'],
toolbarButtonsSM: ['highlightCode'],
toolbarButtonsXS: ['highlightCode'],
})
Notice the example assumes this directory structure:
└───index.html
└───node_modules
└───froala-syntax-highlighter
Caution
Note: you can set the froala-editor and angular-froala-wysiwyg versions, as showed in the comment below, which lies between 3 and 4. In case the version is not specified, the latest stable version will be installed.
ng new $APP_NAME
# Notice that **$APP_NAME** needs to be replaced by the name that you choose.
cd $APP_NAME
npm install --save angular-froala-wysiwyg
npm install --save froala-syntax-highlighter
src/app/app.module.ts
file and add:// From Froala instructions.
// Import all Froala Editor plugins.
import "froala-editor/js/plugins.pkgd.min.js";
// Expose FroalaEditor instance to window.
declare const require: any;
(window as any).FroalaEditor = require("froala-editor");
require("froala-syntax-highlighter/lib"); // Import plugin.
// Import Angular plugin.
import {
FroalaEditorModule,
FroalaViewModule,
} from "angular-froala-wysiwyg";
...
@NgModule({
...
imports: [... FroalaEditorModule.forRoot(), FroalaViewModule.forRoot() ... ],
...
})
.angular.json
file and add:"styles": [
"src/styles.css",
"./node_modules/froala-editor/css/froala_editor.pkgd.min.css",
"./node_modules/froala-editor/css/froala_style.min.css"
]
src/app/app.component.ts
and replace all with:import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
// Set App Title.
title = 'Angular froala demo';
// Initialize the editor content.
public content: string = '<p class="text">const hello = "hello";</p>';
// Set options for the editor.
public options: Object = {
pluginsEnabled: ['syntaxHighlighter'],
toolbarButtons: ['highlightCode'],
};
}
<h1>Angular and Froala demo</h1>
<!-- Pass options and content to the component. -->
<div id="editor" [froalaEditor]="options" [(froalaModel)]="content"></div>
ng serve
create-react-app $APP_NAME
# Notice that **$APP_NAME** needs to be replaced by the name that you choose.
cd $APP_NAME
npm install react-froala-wysiwyg --save
npm install froala-syntax-highlighter --save
src/index.js
by:// Load react default libraries.
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import reportWebVitals from './reportWebVitals';
// Load Froala Editor scripts and styles.
import 'froala-editor/css/froala_style.min.css';
import 'froala-editor/css/froala_editor.pkgd.min.css';
import FroalaEditorComponent from 'react-froala-wysiwyg';
import 'froala-editor/js/plugins.pkgd.min.js';
window.FroalaEditor = require('froala-editor');
// Load Syntax Highlighter -froala plugin.
require('froala-syntax-highlighter');
const froalaConfig = {
pluginsEnabled: ['syntaxHighlighter'],
toolbarButtons: ['highlightCode'],
};
// Set the initial content.
const content = '<p class="text">const hello = "hello"/p>';
ReactDOM.render(
<FroalaEditorComponent tag="div" config={froalaConfig} model={content} />,
document.getElementById('root'),
);
// If you want your app to work offline and load faster, you can change
// unregister() to register() below. Note this comes with some pitfalls.
// Learn more about service workers: https://bit.ly/CRA-PWA
reportWebVitals();
Default theme:
'min-light';
Themes:
export type Theme =
| 'css-variables'
| 'dark-plus'
| 'dracula-soft'
| 'dracula'
| 'github-dark-dimmed'
| 'github-dark'
| 'github-light'
| 'hc_light'
| 'light-plus'
| 'material-darker'
| 'material-default'
| 'material-lighter'
| 'material-ocean'
| 'material-palenight'
| 'min-dark'
| 'min-light'
| 'monokai'
| 'nord'
| 'one-dark-pro'
| 'poimandres'
| 'rose-pine-dawn'
| 'rose-pine-moon'
| 'rose-pine'
| 'slack-dark'
| 'slack-ochin'
| 'solarized-dark'
| 'solarized-light'
| 'vitesse-dark'
| 'vitesse-light';
Programming Languages:
export type Lang =
| 'javascript'
| 'js'
| 'typescript'
| 'c'
| 'c++'
| 'python'
| 'ruby'
| 'php'
| 'go'
| 'java'
| 'html'
| 'css';
To change theme you need to call createHighlighter
method on editor object
createHighlighter(langs: Lang[], theme: StringLiteralUnion<Theme>): Promise<void>;
Example
const config = {
events: {
initialized() {
this.syntaxHighlighter.createHighlighter(['javascript', 'ruby'], 'github-light');
},
},
};
FAQs
Syntax highlighter for Froala with auto detection programming language
The npm package froala-syntax-highlighter receives a total of 7 weekly downloads. As such, froala-syntax-highlighter popularity was classified as not popular.
We found that froala-syntax-highlighter demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.