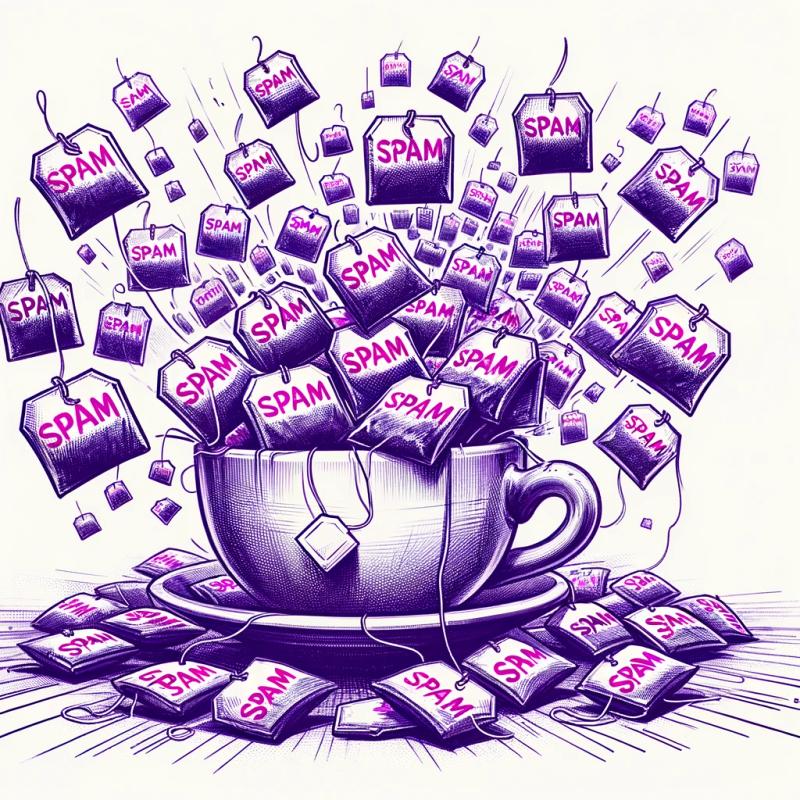
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
ftp-ts
Advanced tools
Changelog
2022-07-29, Version 1.1.0
The old LIST
command was created for manual human reading and as such no data format is defined in the spec, which means there is quite a few variations out there.
RFC3659 introduced two optional commands MLSD
/MLST
to have an extendable semantic format that is readable by software.
Usage of these commands is now preferred if supported by the FTP server.
RFC3659 introduced two optional commands EPSV
and EPRT
to support IPv6 and onwards.
By adding support detection and heuristics these are now available for the client to use.
Added FTP.localPort(bindIp, portRange)
which may be overridden for even better control over port selection and choice of network interfaces.
Readme
FTP-ts is an FTP client module for node.js that provides an asynchronous interface for communicating with a FTP server.
It is a rewritten version of the ftp package from mscdex
.
node.js -- v8.0 or newer
Note: For node version < 10, one of the --harmony_async_iteration
or --harmony
flags must be used.
npm install ftp-ts
import Client from "ftp-ts";
// connect to localhost:21 as anonymous
Client.connect({host: "127.0.0.1", port: 21}).then(async (c) => {
console.dir(await c.list());
c.end();
});
import Client from "ftp-ts";
import { createWriteStream } from "fs";
// connect to localhost:21 as anonymous
Client.connect({host: "127.0.0.1", port: 21}).then(async (c) => {
const stream = await c.get('foo.txt');
stream.pipe(createWriteStream('foo.local-copy.txt'));
c.end();
});
import Client from "ftp-ts";
// connect to localhost:21 as anonymous
Client.connect({host: "127.0.0.1", port: 21}).then((c) => {
c.put('foo.txt', 'foo.remote-copy.txt');
c.end();
})
import Client from "ftp-ts";
// connect to localhost:21 as anonymous
// Config PORT address and PORT range
Client.connect({host: "127.0.0.1", port: 2111, portAddress: "127.0.0.1", portRange: "6000-7000"}).then(async (c) => {
console.dir(await c.list());
c.end();
});
List of implemented required "standard" commands (RFC 959):
List of implemented optional "standard" commands (RFC 959):
List of implemented extended commands (RFC 3659)
Creates a new FTP client.
options
<Object> See ftp.connect for which options are available.Emitted after connection.
Emitted when connection and authentication were sucessful.
Emitted when the connection has fully closed.
Emitted when the connection has ended.
Emitted when an error occurs. In case of protocol-level errors, the error contains a code
property that references the related 3-digit FTP response code.
bindIp
<string> The IP to bind the PORT
socket to.portRange
<string> The range of ports to use when setting up PORT
sockets.This method may be overridden on the instance to allow for better managed PORT
/EPRT
commands.
For example, you may wish to bind only to a specific network interface for security reasons.
In that case you may override this method to return the bindIp
with a value of 127.0.0.1
instead of 0.0.0.0
to only allow incoming connections from localhost.
Another reason may be to decide upon a port number and await
some NAT rules to propagate before the remote server connects.
This could also be useful if your external IP family does not match the family of your interface due to proxying or NAT rules.
By default the zero bindIp
will always be in the same IP family as the external IP set as portAddress
in the IOption
object.
options
<Object>
host
<string> The hostname or IP address of the FTP server. Default: 'localhost'
.port
<number> The port of the FTP server. Default: 21
.portAddress
<string> The external IP address for the server to connect to when using PORT
.portRange
<string> The range of ports to use when setting up PORT
sockets.secure
<boolean> | <string> Set to true
for both control and data connection encryption, 'control'
for control connection encryption only, or 'implicit'
for implicitly encrypted control connection (this mode is deprecated in modern times, but usually uses port 990) Default: false
.secureOptions
<Object> Additional options to be passed to tls.connect()
.user
<string> Username for authentication. Default: 'anonymous'
.password
<string> Password for authentication. Default: 'anonymous@'
.connTimeout
<number> How long (in milliseconds) to wait for the control connection to be established. Default: 10000
.dataTimeout
<number> How long (in milliseconds) to wait for a PASV
/PORT
data connection to be established. Default: 10000
.keepalive
<number> How often (in milliseconds) to send a 'dummy' (NOOP) command to keep the connection alive. Default: 10000
.Connects to an FTP server.
Closes the connection to the server after any/all enqueued commands have been executed.
Closes the connection to the server immediately.
path
<string> Default: current directory.useCompression
<boolean> Default: false
.'d'
for directory, '-'
for file (or 'l'
for symlink on *NIX only).true
if the sticky bit is set for this entry (*NIX only).Get a directory listing from the server.
path
<string> The name of the file to retreive.useCompression
<boolean> Default: false
.Retrieves a file at path
from the server.
input
<string> | <buffer> | <stream.Readable> The file name as a string, the file content as a buffer, the file content as a stream.destPath
<string> The name of the file to write.useCompression
<boolean> Default: false
.Sends data to the server to be stored as destPath
.
input
<string> | <buffer> | <stream.Readable> The file name as a string, the file content as a buffer, the file content as a stream.destPath
<string> The name of the file to write.useCompression
<boolean> Default: false
.Sends data to the server to be stored as destPath
, except if destPath
already exists, it will be appended to instead of overwritten.
oldPath
<string> The name of the file to move.newPath
<string> The new name of the file.Renames oldPath
to newPath
on the server.
Logout the user from the server.
Deletes the file represented by path
on the server.
path
<string> The path to the new working directory.promote
<boolean> Default: false
.Changes the current working directory to path
.
Aborts the current data transfer (e.g. from get(), put(), or list()).
command
<string> SITE
command to send.Sends command
(e.g. 'CHMOD 755 foo', 'QUOTA') using SITE.
Retrieves human-readable information about the server's status.
Sets the transfer data type to ASCII.
Sets the transfer data type to binary (default at time of connection).
path
<string> The path to the new directory.recursive
<boolean> Is for enabling a 'mkdir -p' algorithm Default: false
.Creates a new directory, path
, on the server.
path
<string> The path of the directory to delete.recursive
<boolean> Will delete the contents of the directory if it is not empty Default: false
.Removes a directory, path
, on the server.
Changes the working directory to the parent of the current directory.
Retrieves the current working directory.
Retrieves the server's operating system.
path
<string> Default: current directory.useCompression
<boolean> Default: false
.'d'
for directory, '-'
for file (or 'l'
for symlink on *NIX only).true
if the sticky bit is set for this entry (*NIX only).Similar to list(), except the directory is temporarily changed to path
to retrieve the directory listing. This is useful for servers that do not handle characters like spaces and quotes in directory names well for the LIST command. This function is "optional" because it relies on pwd() being available.
path
<string> The path of the file.Retrieves the size of path
.
path
<string> The path of the file.Retrieves the last modified date and time for path
.
Sets the file byte offset for the next file transfer action (get/put) to byteOffset
.
FAQs
An FTP client module written in typescript
The npm package ftp-ts receives a total of 2,553 weekly downloads. As such, ftp-ts popularity was classified as popular.
We found that ftp-ts demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.