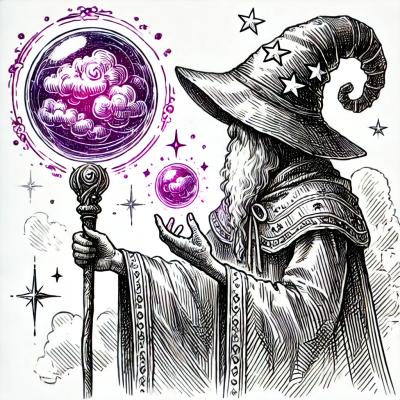
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Gamepad-ts is a heavily typed gamepad contol reciever.
It will detect and connect an USB contoller, and send corresponding events.
This code had been test with DS4Windows
On creation you hae to choose the gamepad model you want to see.
nintendo
, the controller will emit events the following events: LStick: (pos: [number, number]) => void; // Left stick moved event (x, y)
RStick: (pos: [number, number]) => void; // Right stick moved event (x, y)
dpad: (pos: [number, number]) => void; // Digital Pad change event (x, y)
LStickBt: (pressed: boolean) => void; // Left stick press
RStickBt: (pressed: boolean) => void; // Right stick press
X: (pressed: boolean) => void; // nintendo / xbox X Button
A: (pressed: boolean) => void; // nintendo / xbox A Button
B: (pressed: boolean) => void; // nintendo / xbox B Button
Y: (pressed: boolean) => void; // nintendo / xbox Y Button
'+': (pressed: boolean) => void; // nintendo plus Button
'-': (pressed: boolean) => void; // nintendo less Button
L: (pressed: boolean) => void; // nintendo L Button
R: (pressed: boolean) => void; // nintendo R Button
ZL: (pressed: boolean) => void; // nintendo Left Z
ZR: (pressed: boolean) => void; // nintendo Rigth Z
xbox
, the controller will emit events the following events: LStick: (pos: [number, number]) => void; // Left stick moved event (x, y)
RStick: (pos: [number, number]) => void; // Right stick moved event (x, y)
dpad: (pos: [number, number]) => void; // Digital Pad change event (x, y)
LStickBt: (pressed: boolean) => void; // Left stick press
RStickBt: (pressed: boolean) => void; // Right stick press
X: (pressed: boolean) => void; // nintendo / xbox X Button
A: (pressed: boolean) => void; // nintendo / xbox A Button
B: (pressed: boolean) => void; // nintendo / xbox B Button
Y: (pressed: boolean) => void; // nintendo / xbox Y Button
start: (pressed: boolean) => void; // xbox start
back: (pressed: boolean) => void; // xbox back
LB: (pressed: boolean) => void; // xbox Left Bumper
RB: (pressed: boolean) => void; // xbox Right Bumper
LT: (pressed: boolean) => void; // xbox Left Trigger
RT: (pressed: boolean) => void; // xbox Rigth trigger
playstation
, the controller will emit events the following events: LStick: (pos: [number, number]) => void; // Left stick moved event (x, y)
RStick: (pos: [number, number]) => void; // Right stick moved event (x, y)
dpad: (pos: [number, number]) => void; // Digital Pad change event (x, y)
LStickBt: (pressed: boolean) => void; // Left stick press
RStickBt: (pressed: boolean) => void; // Right stick press
square: (pressed: boolean) => void; // Playastation Button
cross: (pressed: boolean) => void; // Playastation Button
circle: (pressed: boolean) => void; // Playastation Button
triangle: (pressed: boolean) => void; // Playastation Button
share: (pressed: boolean) => void; // Playstation share
options: (pressed: boolean) => void; // Playstation options
L1: (pressed: boolean) => void; // playstaton Left Button
R1: (pressed: boolean) => void; // playstaton Right Button
L2: (pressed: boolean) => void; // playstaton Left trigger
R2: (pressed: boolean) => void; // playstaton Right trigger
generic
, the controller will emit events the following events: LStick: (pos: [number, number]) => void; // Left stick moved event (x, y)
RStick: (pos: [number, number]) => void; // Right stick moved event (x, y)
dpad: (pos: [number, number]) => void; // Digital Pad change event (x, y)
LStickBt: (pressed: boolean) => void; // Left stick press
RStickBt: (pressed: boolean) => void; // Right stick press
BL: (pressed: boolean) => void; // Button left
BD: (pressed: boolean) => void; // Button down
BR: (pressed: boolean) => void; // Button Rigth
BT: (pressed: boolean) => void; // Button Top
BMR: (pressed: boolean) => void; // Button Middle Rigth
BML: (pressed: boolean) => void; // Button Middle Left
L1: (pressed: boolean) => void; // generic / playstaton Left Bumper
R1: (pressed: boolean) => void; // generic / playstaton Right Bumper
L2: (pressed: boolean) => void; // generic / playstaton Left trigger
R2: (pressed: boolean) => void; // generic / playstaton Right trigger
Note:
X
Y
A
B
are differently mapped on Nintendo and XBox, So it's inportant to choose the correct mapping.import GamePadController from './src/index';
const ctrl = new GamePadController({brand: 'playStation'});
/*
* A,B,X,Y buttons
*/
ctrl.on('LStick', (pos) => console.log(`L: ${pos[0]}, ${pos[1]}`));
ctrl.on('RStick', (pos) => console.log(`R: ${pos[0]}, ${pos[1]}`));
ctrl.on('dpad', (pos) => console.log(`dPad: ${pos[0]}, ${pos[1]}`));
ctrl.on('square', (pressed: boolean) => console.log('square: ' + pressed));
ctrl.on('cross', (pressed: boolean) => console.log('cross: ' + pressed));
ctrl.on('circle', (pressed: boolean) => console.log('circle: ' + pressed));
ctrl.on('triangle', (pressed: boolean) => console.log('triangle: ' + pressed));
/*
* Setup
*/
ctrl.on('connected', () => console.log('Xbox controller connected'));
ctrl.on('error', (err) => console.error(err));
ctrl.start();
FAQs
gamepad contoller in typescript
The npm package gamepad-ts receives a total of 1 weekly downloads. As such, gamepad-ts popularity was classified as not popular.
We found that gamepad-ts demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.