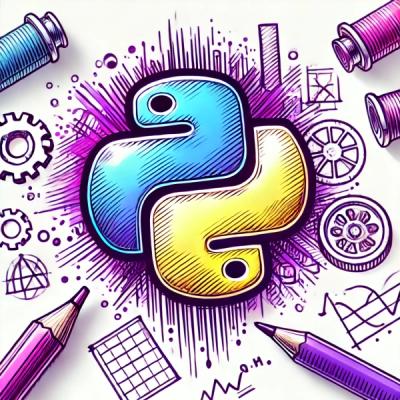
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
gd-sprest
Advanced tools
An easy way to develop against the SharePoint REST api.
Please report issues. I am constantly updating/fixing/testing to make this library better.
npm install gd-sprest --save-dev
I was finally able to put the definition file together, to ensure intellisense is available.
Bootstrap List Note - This is still in dev
A global flag is used to determine if an app web request should execute requests against the host web. The host web will default to the SPHostUrl query string value. Note - This value is false by default
$REST.DefaultRequestToHostWebFl = true;
The 'execute' method determines if the request should be executed asynchronously or synchronously. By default, all requests will be executed asynchronously, unless overridden by the developer.
The 'next' method allows you to create multiple requests against the base object. To ensure intellisense for the 'next' method, you will need to specify the base object type:
// Get the current web
(new $REST.Web())
// Get the root folders
.Folders()
// Execute the request
.next<$REST.Types.IWeb>()
// Get the 'Dev' list
.Lists("Dev")
// Get the list item collection
.Items()
// Execute the request
.execute((folders:$REST.Types.IFolders, items:$REST.Types.IListItems) => {
// Code executed after all requests have completed
});
Asynchronous Request
// Get the current web
(new Web())
// Execute the request
.execute(function(web) {
// Code to execute after the request completes
});
Synchronous Request
var web = (new $REST.Web())
// Set the flag to execute the request synchronously
.execute(true);
Multiple Asynchronous Requests
// Get the current web
(new $REST.Web())
// Get the Field Collection
.Fields()
// Query for all fields with the name of 'Title'
.query({
Filter: "Title eq 'Title'"
})
// Execute this request
.next()
// Get the 'Dev' List
.Lists("Dev")
// Get the items
.Items()
// Execute this request
.next()
// Get root folders
.Folders()
// Execute the request
.execute(function(fields, items, folders) {
// This code will execute after all requests have completed
});
Having the ability to chain the 'Properties', it allows for the developer to get the target object with one request to the server.
// This will create the web object
var list = (new $REST.Web())
// Get the list collection
.Lists()
// Create a list
.add({
BaseTemplate: 100,
Description: "This is a test list.",
Title: "Test"
})
// Execute the request
.execute(true);
// This will create the web object
(new $REST.Web())
// Get the list collection
.Lists()
// Create the list
.add({
BaseTemplate: 100,
Description: "This is a test list.",
Title: "Test"
})
// Execute the request
.execute(function(list) {
// Additional code goes here
});
// Get the list
var items = (new $REST.List("[List Name]"))
// Query using OData
.query({
// OData properties - Refer to the OData section for additional details
})
// Execute the request
.execute(true);
// Example of getting items by a CAML Query
(new $REST.List("Site Assets"))
// Get the items by a CAML Query
.getItemsByQuery("<Query><Where><Gt><FieldRef Name='ID' /><Value Type='Integer'>0</Value></Gt></Where></Query>")
// Execute the request
.execute(true);
// Example of getting items by a CAML View Query
(new $REST.List("Site Assets"))
// Get the items by a CAML View Query
.getItems("<View Scope='RecursiveAll'><Query><Where><Eq><FieldRef Name='FileLeafRef' /><Value Type='File'>sprest.js</Value></Eq></Where></Query></View>")
// Execute the request
.execute(true);
All constructors take have the following optional parameters:
// The target information is optional
new Object([Object Specific Input Parameters], targetInfo);
The target information consists of the following properties:
Since the library can be executed synchronously, the user can execute commands in the browser's console window and interact with the SharePoint site in a command-line interface.
Note - The commands will execute under the security of the current user. Note - SharePoint online may reject synchronous requests. It's better to use asynchronous requests.
Each collection will have a generic "query" method with the input of the OData query operations. The oData object consists of the following properties:
// Get the current web's list collection
var list = (new $REST.List())
// Query for the 'Dev' list
.query({
Filter: ["Title eq 'Dev'"]
})
// Execute the request
.execute(true);
// Get the 'Dev' list
(new $REST.List("Dev"))
// Get the item collection
.Items()
// Query for my items, expanding the created by information
.query({
Select: ["Title", "Author/Id", "Author/Title"],
Expand: ["Author"],
Filter: "AuthorId eq 11"
})
// Execute the request
.execute(function(items) {
// Code goes here
});
I wrote a sample test file. To run it, upload the test folder contents to a SharePoint library and access to the "test.aspx" page. This will test the basic functionality of the library.
Refer to the test script file for detailed examples of using the library.
Note - The examples below will execute one request to the server.
List
// Get the 'Document' content type in the 'Documents' library
var ct = (new $REST.List("Document"))
.ContentTypes()
.getByName("Documents")
.execute(true);
Web
// Get the 'Item' content type in the current web
var ct = (new $REST.Web())
.ContentTypes()
.getByName("Item")
.execute(true);
List
// Get the content types in the 'Documents' library
var cts = (new $REST.List("documents"))
.ContentTypes()
.execute(true);
Web
// Get the content types in the current web
(new $REST.Web())
.ContentTypes()
.execute(function(cts) {
// Additional code goes here
})
List
var field = (new $REST.List("documents"))
.Fields("Title")
.execute(true);
Web
(new $REST.Web())
.Fields("Title")
.execute(function(field) {
// Additional code goes here
});
List
var fields = (new $REST.List("documents"))
.Fields()
.execute(true);
Web
(new $REST.Web())
.Fields()
.execute(function(fields) {
// Additional code goes here
});
List
(new $REST.List("Documents"))
.RootFolder()
.Folders("forms")
.Files("EditForm.aspx")
.execute(function(file) {
// Additional code goes here
})
var file = (new $REST.List("Documents"))
.RootFolder()
.Folders("forms")
.Files("editform.aspx")
execute(true);
Web
var file = (new $REST.Web())
.getFileByServerRelativeUrl("/sites/dev/shared documents/forms/editform.aspx")
.execute(true);
List
var files = (new $REST.List("documents"))
.RootFolder()
.Files()
.execute(true);
// Get a specific folder's files
var folders = (new $REST.Web())
.getFolderByServerRelativeUrl("/sites/dev/shared documents/forms")
.Files()
.execute(true);
Web
var files = (new $REST.Web())
.Files()
.execute(true);
List
var folder = (new $REST.List("Documents"))
.RootFolder("Forms")
.execute(true)
Web
var folder = (new $REST.Web())
.getFolderByServerRelativeUrl("/sites/dev/shared documents/forms")
.execute(true);
List
// Get the root folders of the library
var folders = (new $REST.List("documents"))
.Folders()
.execute(true);
// Get a specific folder's sub-folder
var folders = (new $REST.Web())
.getFolderByServerRelativeUrl("/sites/dev/shared documents/forms")
.Folders()
.execute(true);
Web
var folders = (new $REST.Web())
.Folders()
.execute(true);
var list = (new $REST.List("documents")).execute(true);
var lists = (new $REST.Web())
.Lists()
.execute(true);
var item = (new $REST.List("documents"))
.Items(1)
.execute(true);
All Items
var items = (new $REST.List("documents"))
.Items()
.execute(true);
CAML Query
(new $REST.List("documents"))
// Get the items
.getItemsByQuery("<Query><Where><Gt><FieldRef Name='ID' /><Value Type='Integer'>0</Value></Gt></Where></Query>")
// Execute after the request completes
.execute(function(items)) {
// Code goes here
}
List
var roleAssignments = (new $REST.List("documents"))
.RoleAssignments()
.execute(true);
Web
var roleAssignments = (new $REST.Web())
.RoleAssignments()
.execute(true);
var roleDefinitions = (new $REST.List("documents"))
.RoleDefinitions()
.execute(true);
var site = (new $REST.Site()).execute();
var siteGroups = (new $REST.Web())
.SiteGroups()
.execute(true);
Site
var customActions = (new $REST.Site())
.UserCustomActions()
.execute(true);
Web
var customActions = (new $REST.Web())
.UserCustomActions()
.execute(true);
var users = (new $REST.Web())
.Users()
.execute(true);
var view = (new $REST.List("dev"))
.Views()
.query({
Filter: "Name eq 'All Items'"
})
.execute(true);
var views = (new $REST.List("documents"))
.Lists()
.execute(true);
var web = (new $REST.Web()).execute(true);
FAQs
An easy way to develop against the SharePoint REST API.
The npm package gd-sprest receives a total of 1,045 weekly downloads. As such, gd-sprest popularity was classified as popular.
We found that gd-sprest demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.