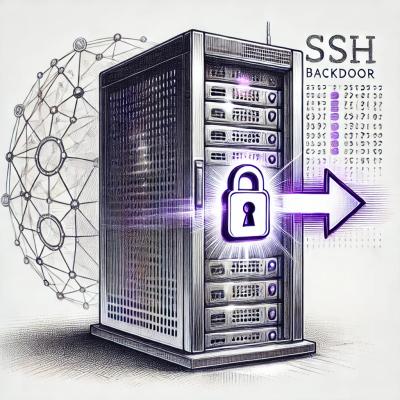
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Generator source code and files, with: text format, minification, indenting, and beautify.
This library also provides all the functions necessary to convert between different data types and store in files.
$> npm install gencode --save
var gencode = require('gencode');
//or
import gencode from 'gencode';
Storing an array in a file of any extension.
var array = ['1', '2', '555543', '23423', 'Lorem ipsum', 'Lorem ipsum 2', 'Lorem ipsum 3', 231];
gencode.toFile(array, '/home/julian/Desktop/test', 'array.txt', '\n').then((value) => {
console.log("Result: ", value);
/* Result: successfull */
}, function(err) {
console.log("Error: ", err);
});
/*
Content of file /home/julian/Escritorio/test/array.txt =>
1
2
555543
23423
Lorem ipsum
Lorem ipsum 2
Lorem ipsum 3
231
*/
Note:
- The main priority is the spaces, ie, if there are spaces and tabs then the_ spaces are taken.
var input = {
name: 'index.html',
content: [{
line: '<title>Example</title>',
space: 0,
tabs: 0
}, {
line: '<div class="header">',
}, {
line: '<div class="container">',
space: 4,
tabs: 1
}, {
line: '<div class="logo">',
space: 5,
tabs: 1
}, {
line: '<a href="/">Title</a>',
space: 5,
tabs: 1
}, {
line: '</div>',
space: 5,
tabs: 1
}, {
line: '<div class="header-right">',
space: 5,
tabs: 1
}, {
line: '<a href="/empresa"><span>Hello</span></a>',
space: 5,
tabs: 1
}, {
line: '</div>',
space: 2,
tabs: 1,
repeat: 2
}, {
tabs: 2
}]
};
var input = {
name: 'index.html',
content: [{
line: '<title>Example</title>',
space: 0,
tabs: 0
}, {
line: '<div class="header">',
}, {
line: '<div class="container">',
tabs: 1
}, {
line: '<div class="logo">',
space: 5
}, {
line: '<a href="/">Title</a>',
space: 5
}, {
line: '</div>',
space: 5
}, {
line: '<div class="header-right">',
tabs: 1
}, {
line: '<a href="/empresa"><span>Hello</span></a>',
space: 5
}, {
line: '</div>',
space: 2,
repeat: 2 //Repeats </div> two times
}]
};
gencode.generatorfull(input, "/home/julian/Desktop/test/", "index.html").then((value) => {
console.log("output: ", value);
}, function(err) {
console.log("Error: ", err);
})
var input = {
name: 'index.html',
content: [{
line: '<title>Example</title>',
space: 0,
tabs: 0
}, {
line: '<div class="header">',
}, {
line: '<div class="container">',
space: 4,
tabs: 1
}, {
line: '<div class="logo">',
space: 5,
tabs: 1
}, {
line: '<a href="/">Title</a>',
space: 5,
tabs: 1
}, {
line: '</div>',
space: 5,
tabs: 1
}, {
line: '<div class="header-right">',
space: 5,
tabs: 1
}, {
line: '<a href="/empresa"><span>Hello</span></a>',
space: 5,
tabs: 1
}, {
line: '</div>',
space: 2,
tabs: 1,
repeat: 2
}, {
tabs: 2 //this is an error in strict mode.
}]
};
/**
* [generator description]
* @param {[json]} input [json input]
* @param {[boolean]} false [strict mode, ends the generation if an error is found.]
* @return {[string]} [output]
*/
gencode.generator(input, false).then((value) => {
console.log("output: ", value);
}, function(err) {
console.log("Error: ", err);
});
Content file ():
gencode.utils.toArray('/home/julian/Desktop/test/entrada.txt', 'utf8', '\n').then((value) => { //Too: \n, \t, -, etc.
console.log("Result:", JSON.stringify(value));
}, (error) => {
console.log("ERROR=>", error);
});
/*
Result: ["a","aaronita","aarónico","aba","ababa","ababillarse","ababol","abacal"]
*/
var content = "if (err) { reject(err); } else { resolve('successfull'); }";
gencode.save(content, '/home/julian/Escritorio/test', 'save.js').then((value) => {
console.log("Result:\n", value);
}, function(err) {
console.log("Error: ", err);
});
console.log(gencode.utils.separator(3, "\n") + "|Ends here the result");
//Result:
/*
|Ends here the result
*/
console.log(gencode.utils.separator(3, "\t") + "|Ends here the result");
//Result:
// |Ends here the result
console.log(gencode.utils.separator(4, "_") + "|Ends here the result");
//Result:
//____|Ends here the result
var array = ['any', 'number', 'or', 'string', 100, 200];
gencode.utils.showArray(array);
//Result:
//any
//number
//or
//string
//100
//200
var array = ['any', 'number', 'or', 'string', 100, 200];
console.log(gencode.utils.arrayToString(array, '\t')); //\t, \n, _, etc.
//any number or string 100 200
FAQs
Generator source code and files, with: text format, minification, indenting, and beautify.
The npm package gencode receives a total of 8 weekly downloads. As such, gencode popularity was classified as not popular.
We found that gencode demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.