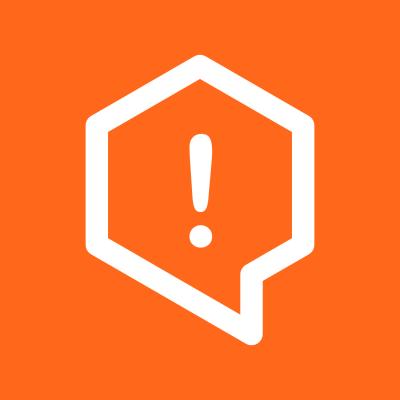
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
The geolib npm package provides a set of functions for common geospatial calculations. It allows you to perform operations such as distance calculations, finding the center of a set of coordinates, and working with bounding boxes.
Distance Calculation
This feature allows you to calculate the distance between two geographic coordinates. The `getDistance` function returns the distance in meters.
const geolib = require('geolib');
const distance = geolib.getDistance(
{ latitude: 51.5103, longitude: 7.49347 },
{ latitude: 51.515, longitude: 7.453619 }
);
console.log(distance); // Outputs distance in meters
Finding the Center of Coordinates
This feature allows you to find the geographic center of a set of coordinates. The `getCenter` function returns the center coordinate.
const geolib = require('geolib');
const center = geolib.getCenter([
{ latitude: 51.5103, longitude: 7.49347 },
{ latitude: 51.515, longitude: 7.453619 },
{ latitude: 51.525, longitude: 7.470 }
]);
console.log(center); // Outputs the center coordinate
Bounding Box Calculation
This feature allows you to calculate the bounding box for a set of coordinates. The `getBounds` function returns the bounding box.
const geolib = require('geolib');
const bounds = geolib.getBounds([
{ latitude: 51.5103, longitude: 7.49347 },
{ latitude: 51.515, longitude: 7.453619 },
{ latitude: 51.525, longitude: 7.470 }
]);
console.log(bounds); // Outputs the bounding box
Turf is a powerful geospatial analysis library that provides a wide range of geospatial functions. It is more comprehensive than geolib and includes advanced features like spatial joins, clustering, and interpolation.
Geodesy is a library for geodesic calculations. It provides functions for distance calculations, bearing, and other geospatial operations. It is similar to geolib but focuses more on geodesic calculations.
Geokit is a library for geospatial calculations and queries. It provides functions for distance calculations, bounding boxes, and other geospatial operations. It is similar to geolib but includes additional features for working with geospatial data in databases.
A small library to provide some basic geo functions like distance calculation, conversion of decimal coordinates to sexagesimal and vice versa, etc.
Calculates the distance between two geo coordinates
Takes 2 or 3. First 2 arguments must be an object with a latitude and a longitude property (e.g. {latitude: 52.518611, longitude: 13.408056}). Coordinates can be in sexagesimal or decimal format. 3rd argument is accuracy (in meters). So a calculated distance of 1248 meters with an accuracy of 100 is returned as 1200.
Return value is always an integer and represents the distance in meters.
geolib.getDistance({latitude: 51.5103, longitude: 7.49347}, {latitude: "51° 31' N", longitude: "7° 28' E"}); geolib.getDistance({latitude: 51.5103, longitude: 7.49347}, {latitude: "51° 31' N", longitude: "7° 28' E"}); // Working with W3C Geolocation API navigator.geolocation.getCurrentPosition(function(position) { alert('You are ' + geolib.getDistance(position.coords, {latitude: 51.525, longitude: 7.4575}) + ' meters away from 51.525, 7.4575'); }, function() { alert('Position could not be determined.'), {enableHighAccuracy: true});
Calculates the geographical center of all points in a collection of geo coordinates
Takes an object or array of coordinates and calculates the center of it.
Returns an object: {"latitude": centerLat, "longitude": centerLng, "distance": diagonalDistance}
var spots = { "Brandenburg Gate, Berlin": {latitude: 52.516272, longitude: 13.377722}, "Dortmund U-Tower": {latitude: 51.515, longitude: 7.453619}, "London Eye": {latitude: 51.503333, longitude: -0.119722}, "Kremlin, Moscow": {latitude: 55.751667, longitude: 37.617778}, "Eiffel Tower, Paris": {latitude: 48.8583, longitude: 2.2945}, "Riksdag building, Stockholm": {latitude: 59.3275, longitude: 18.0675}, "Royal Palace, Oslo": {latitude: 59.916911, longitude: 10.727567} } geolib.getCenter(spots); geolib.getCenter([ {latitude: 52.516272, longitude: 13.377722}, {latitude: 51.515, longitude: 7.453619}, {latitude: 51.503333, longitude: -0.119722} ]);
Checks whether a point is inside of a polygon or not. Note: the polygon coords must be in correct order!
Returns true or false
geolib.isPointInside( {latitude: 51.5125, longitude: 7.485}, [ {latitude: 51.50, longitude: 7.40}, {latitude: 51.555, longitude: 7.40}, {latitude: 51.555, longitude: 7.625}, {latitude: 51.5125, longitude: 7.625} ] ); // -> true
Similar to is point inside: checks whether a point is inside of a circle or not.
Returns true or false
// checks if 51.525, 7.4575 is within a radius of 5km from 51.5175, 7.4678 geolib.isPointInCircle({latitude: 51.525, longitude: 7.4575}, {latitude: 51.5175, longitude: 7.4678}, 5000);
Sorts an object or array of coords by distance from a reference coordinate
Returns a sorted array [{latitude: x, longitude: y, distance: z, key: property}]
// coords array geolib.orderByDistance({latitude: 51.515, longitude: 7.453619}, [ {latitude: 52.516272, longitude: 13.377722}, {latitude: 51.518, longitude: 7.45425}, {latitude: 51.503333, longitude: -0.119722} ]); // coords object geolib.orderByDistance({latitude: 51.515, longitude: 7.453619}, { a: {latitude: 52.516272, longitude: 13.377722}, b: {latitude: 51.518, longitude: 7.45425}, c: {latitude: 51.503333, longitude: -0.119722} });
Finds the nearest coordinate to a reference coordinate.
var spots = { "Brandenburg Gate, Berlin": {latitude: 52.516272, longitude: 13.377722}, "Dortmund U-Tower": {latitude: 51.515, longitude: 7.453619}, "London Eye": {latitude: 51.503333, longitude: -0.119722}, "Kremlin, Moscow": {latitude: 55.751667, longitude: 37.617778}, "Eiffel Tower, Paris": {latitude: 48.8583, longitude: 2.2945}, "Riksdag building, Stockholm": {latitude: 59.3275, longitude: 18.0675}, "Royal Palace, Oslo": {latitude: 59.916911, longitude: 10.727567} } // in this case set offset to 1 otherwise the nearest point will always be your reference point geolib.findNearest(spots['Dortmund U-Tower'], spots, 1)
Calculates the length of a collection of coordinates
Returns the length of the path in kilometers
// Calculate distance from Berlin via Dortmund to London geolib.getPathLength([ {latitude: 52.516272, longitude: 13.377722}, // Berlin {latitude: 51.515, longitude: 7.453619}, // Dortmund {latitude: 51.503333, longitude: -0.119722} // London ]); // -> 945235
Converts a given distance (in meters) to another unit.
unit
can be one of:
distance
distance to be converted (source must be in meter)
round
fractional digits
geolib.convertUnit('km', 14213, 2) // -> 14,21
Converts a sexagesimal coordinate to decimal format
geolib.sexagesimal2decimal("51° 29' 46\" N")
Converts a decimal coordinate to sexagesimal format
geolib.decimal2sexagesimal(51.49611111); // -> 51° 29' 46.00
Checks if a coordinate is already in decimal format and, if not, converts it to
geolib.useDecimal("51° 29' 46\" N"); // -> 51.59611111 geolib.useDecimal(51.59611111) // -> 51.59611111
FAQs
Library to provide basic geospatial operations like distance calculation, conversion of decimal coordinates to sexagesimal and vice versa, etc. This library is currently **2D**, meaning that altitude/elevation is not yet supported by any of its functions!
The npm package geolib receives a total of 0 weekly downloads. As such, geolib popularity was classified as not popular.
We found that geolib demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.