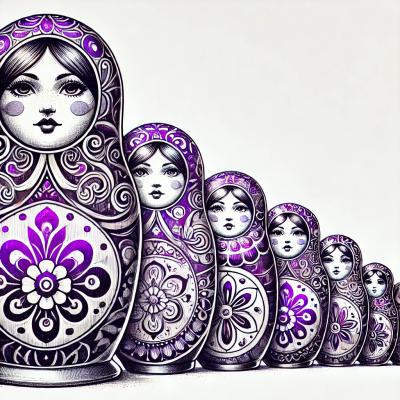
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
get-pkg-repo
Advanced tools
The get-pkg-repo npm package is designed to extract repository information from a package.json file. This can be useful for various tasks such as automating repository-related workflows, generating documentation, or integrating with other tools that require repository details.
Extract Repository URL
This feature allows you to extract the repository URL from a package.json file. The code sample demonstrates how to require the get-pkg-repo package, read the package.json file, and then extract and log the repository URL.
const getPkgRepo = require('get-pkg-repo');
const pkg = require('./package.json');
const repo = getPkgRepo(pkg);
console.log(repo.url);
Extract Repository Type
This feature allows you to extract the type of repository (e.g., git) from a package.json file. The code sample shows how to extract and log the repository type.
const getPkgRepo = require('get-pkg-repo');
const pkg = require('./package.json');
const repo = getPkgRepo(pkg);
console.log(repo.type);
Extract Repository Directory
This feature allows you to extract the directory within the repository if specified in the package.json file. The code sample demonstrates how to extract and log the repository directory.
const getPkgRepo = require('get-pkg-repo');
const pkg = require('./package.json');
const repo = getPkgRepo(pkg);
console.log(repo.directory);
The normalize-package-data package is used to normalize package metadata, including repository information. It provides a more comprehensive solution for handling package.json data but may be overkill if you only need repository details.
The parse-package-name package is designed to parse package names and extract information such as scope and name. While it doesn't specifically focus on repository information, it can be used in conjunction with other tools to achieve similar results.
The read-pkg package is used to read and parse package.json files. It provides a simple way to access package metadata, including repository information, but requires additional logic to extract specific details like repository URL or type.
Get repository url from package json data
People usually have different formats of repository url in package.json and sometimes they even have a typo.
This module uses normalize-package-data, github-url-from-git and npm/repo to parse data. Please check them out for more details.
This module can fix some common typos.
$ npm install --save get-pkg-repo
var fs = require('fs');
var getPkgRepo = require('get-pkg-repo');
fs.readFile('package.json', function(err, pkgData) {
if (err) {
...
}
var url = getPkgRepo(pkgData);
console.log(url)
//=> https://github.com/stevemao/get-pkg-repo
})
getPkgRepo(pkgData, [fixTypo, [warn]])
Returns a string.
Type: string
or json
Type: boolean
If you want to fix your typical typos automatically, pass true. See the list of predefined typos.
Type: function
or boolean
If fixTypo
is true
you can also pass a warn function. console.warn.bind(console)
is passed if it's true.
You can use cli to see what your url will look like after being parsed.
You can enter interactive mode by typing
$ get-pkg-repo
You can use the command followed by a url. You can write more than one repository urls at a time.
$ npm install --global get-pkg-repo
$ get-pkg-repo bitbucket.org/a/b.git
http://bitbucket.org/a/b
You can also validate your package.json
$ cat package.json | get-pkg-repo --fix-typo
https://github.com/stevemao/get-pkg-repo
MIT © Steve Mao
FAQs
Get repository user and project information from package.json file contents.
We found that get-pkg-repo demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.