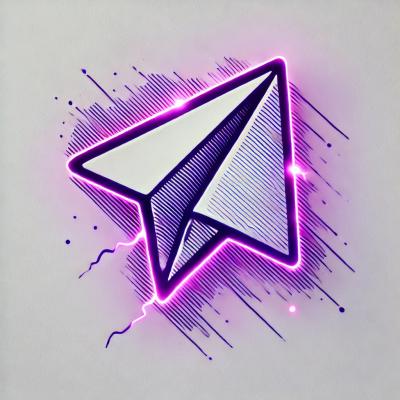
Research
npm Malware Targets Telegram Bot Developers with Persistent SSH Backdoors
Malicious npm packages posing as Telegram bot libraries install SSH backdoors and exfiltrate data from Linux developer machines.
gherkin-assembler
Advanced tools
It allows to convert Gherkin AST to feature file string.
objectToAST(document)
The objectToAST
API method converts the given Gherkin object into GherkinDocument
object.
A complete sample Gherkin object can be found here: Complete Gherkin object.
Params:
{Object} documet
- a single Gherkin objectReturns: {GherkinDocument}
format(document, options)
The format
API method formats the given Gherkin Document(s) to string (which could be written out to feature file(s)).
Params:
{GherkinDocument|Array<GherkinDocument>} document
- a single or multiple Gherkin Documents given in it's AST model.{AssemblerConfig} options
- options to set attributes of formatting.Returns: {string|Array<string>}
'use strict';
const fs = require('fs');
const assembler = require('gherkin-assembler');
const document = assembler.objectToAST(require('./login.ast.json'));
fs.writeFileSync('./login.feature', assembler.format(document), 'utf8');
AssemblerConfig
By passing an AssemblerConfig
object to format method (or other Ast type methods where it's applicable) it can be set, how feature file text is rendered.
Option | Description | Default |
---|---|---|
oneTagPerLine | Should tags rendered separately, one by line? | false , i.e. all tag of a scenario, feature, etc will be rendered in the same line |
separateStepGroups | Should step groups (when-then) be separated? | false |
compact | Should empty lines be skipped, removed from the result? | false , i.e. there will be empty lines in appropriate places |
lineBreak | The line break character(s). | \n , i.e. it uses Unix line break, to set Windows style, set \r\n |
indentation | The indentation character(s). | ' ' , i.e. it uses two space character to add indentation where it's appropriate |
The API provides types to be able to handle different parts of Gherkin feature files.
'use strict';
const {AST} = require('gherkin-assembler');
console.log(Object.keys(AST));
// Background,...,Feature,GherkinDocument,...,Tag
GherkinDocument
Model of a complete Gherkin document, i.e. feature file.
{Feature} feature
- The feature which this document contains.new GherkinDocument() : GherkinDocument
- Creates a new instance.{GherkinDocument}.toString({AssemblerConfig} [options]) : string
- Converts the document to string, i.e. formats it.{GherkinDocument}.clone() : GherkinDocument
- Clones the document.GherkinDocumnet.parse({Object} object) : GherkinDocument
- Parses the given GherkinDocument object to a GherkinDocument
.Feature
Model of a Gherkin feature.
Feature: Hello world
As a smo
I want to do smth
So that I am smth
{string} keyword
- The keyword of the feature, e.g. "Feature"
.{string} name
- The name of the feature, e.g. "Hello world"
.{string} description
- The description of the feature, e.g. "As a smo\nI want to do smth\nSo that I am smth"
.{string} language
- Tne of the supported Gherkin language, default: "en"
.{Array<Tag>} tags
- Tags of the feature.{Array<Background|Scenario|ScenarioOutline>} elements
- The elements of the feature, i.e. scenario, background or scenario outline.new Feature(keyword, name, description, language) : Feature
- Creates a new Feature
object, with the given values.{Feature}.toString({AssemblerConfig}) : string
- Converts the feature to string, i.e. formats it.{Feature}.clone() : Feature
- Clones the feature.Feature.parse({Object} object) : Feature
- Parses the given Feature object to a Feature
.Background
Model of a Gherkin Background scenario.
Background: Some background steps
Given this is a given step
And this is a given step too
When this is a when step
And this is a when step too
Then it should be a then step
And it should be a then step too
{string} keyword
- The keyword of the background, e.g. "Background"
.{string} name
- The name of the background, e.g. "Some background steps"
.{string} description
- The description of the background.{Array<Step>} steps
- The steps of the background.new Background(keyword, name, description) : Background
- Creates a new Background
object, with the given values.{Background}.useNormalStepKeywords()
- Sets the keywords of all step to normal keywords, i.e. Given
, When
, Then
.{Background}.useReadableStepKeywords()
- Sets the keywords of steps to more readable ones, if applicable, i.e. replaces multiple normal keywords with And
keyword.{Background}.toString({AssemblerConfig}) : string
- Converts the background to string, i.e. formats it.{Background}.clone() : Background
- Clones the background.Background.parse({Object} object) : Background
- Parses the given Background object to a Background
.Scenario
Model of a Gherkin scenario.
@tag2 @tag3
Scenario: Name of scenario
Description of the scenario
Given this is a given step
And this is a given step too
{string} keyword
- The keyword of the scenario, e.g. "Scenario"
.{string} name
- The name of the scenario, e.g. "Name of scenario"
.{string} description
- The description of the scenario, e.g. "Description of the scenario"
.{Array<Step>} steps
- The steps of the scenario.{Array<Tag>} tags
- Tags of the scenario.new Scenario(keyword, name, description) : Scenario
- Creates a new Scenario
object, with the given values.{Scenario}.useNormalStepKeywords()
- Sets the keywords of all step to normal keywords, i.e. Given
, When
, Then
.{Scenario}.useReadableStepKeywords()
- Sets the keywords of steps to more readable ones, if applicable, i.e. replaces multiple normal keywords with And
keyword.{Scenario}.toString({AssemblerConfig}) : string
- Converts the scenario to string, i.e. formats it.{Scenario}.clone() : Scenario
- Clones the scenario.Scenario.parse({Object} object) : Scenario
- Parses the given Scenario object to a Scenario
.ScenarioOutline
Model of a Gherkin Scenario outline.
@tag2 @tag(3)
Scenario Outline: Name of outline <key>
Given this is a given step
And this is a given step too
When this is a when step <key>
And this is a when step too
Then it should be a then step
And it should be a then step too
@tagE1
Examples: First examples
| key |
| value1 |
{string} keyword
- The keyword of the scenario outline, e.g. "Scenario Outline"
.{string} name
- The name of the scenario outline, e.g. "Name of outline <key>"
.{string} description
- The description of the scenario outline.{Array<Step>} steps
- The steps of the scenario outline.{Array<Tag>} tags
- Tags of the scenario outline.{Array<Examples>} examples
- Examples of the scenario outline.new ScenarioOutline(keyword, name, description) : ScenarioOutline
- Creates a new ScenarioOutline
object, with the given values.{ScenarioOutline}.useNormalStepKeywords()
- Sets the keywords of all step to normal keywords, i.e. Given
, When
, Then
.{ScenarioOutline}.useReadableStepKeywords()
- Sets the keywords of steps to more readable ones, if applicable, i.e. replaces multiple normal keywords with And
keyword.{ScenarioOutline}.toString({AssemblerConfig}) : string
- Converts the scenario outline to string, i.e. formats it.{ScenarioOutline}.clone() : ScenarioOutline
- Clones the scenario outline.ScenarioOutline.parse({Object} object) : ScenarioOutline
- Parses the given ScenarioOutline object to a ScenarioOutline
.Examples
Model of a Gherkin Scenario outline Examples table.
@tagE1
Examples: First examples
| key |
| value1 |
{string} keyword
- The keyword of the examples table, e.g. "Examples"
.{string} name
- The name of the examples table, e.g. "First examples"
.{Array<Tag>} tags
- Tags of the examples table.{TableRow} header
- The header row of the examples table, with column name(s).{Array<TableRow>} body
- The data rows of the examples table.new Examples(keyword, name, description) : Examples
- Creates a new Examples
object, with the given values.{Examples}.toString({AssemblerConfig}) : string
- Converts the examples table to string, i.e. formats it.{Examples}.clone() : Examples
- Clones the examples table.Examples.parse({Object} object) : Examples
- Parses the given Examples object to an Examples
.Step
Model of a Gherkin step.
Given this is a given step
And this is a given step too
{string} keyword
- The keyword of the step, e.g. "Given"
.{string} text
- The text of the step, e.g. "this is a given step"
.{DocString|DataTable} argument
- The argument of the step if there is any. It could be a DocString
or a DataTable
.new Step(keyword, text) : Step
- Creates a new Step
object, with the given values.{Step}.toString({AssemblerConfig}) : string
- Converts the step to string, i.e. formats it.{Step}.clone() : Step
- Clones the step.Step.parse({Object} object) : Step
- Parses the given Step object to a Step
.Tag
Model of a Gherkin tag (annotation).
@tag(1)
{string} value
- The tag itself, e.g. "@tag(1)"
.new Tag(value) : Tag
- Creates a new Tag
object, with the given values.{Tag}.toString() : string
- Converts the tag to string, i.e. formats it.{Tag}.clone() : Tag
- Clones the tag.Tag.parse({Object} object) : Tag
- Parses the given Tag object to a Tag
.DocString
Model of a Gherkin DocString step argument.
And this is a when step with doc string
"""
Hello world
Hello World
hello World
hello world
"""
{string} content
- The content of the docString, e.g. "Hello world\nHello World..."
.new DocString(value) : DocString
- Creates a new DocString
object, with the given values.{DocString}.toString({AssemblerConfig}) : string
- Converts the docString to string, i.e. formats it.{DocString}.clone() : DocString
- Clones the docString.DocString.parse({Object} object) : DocString
- Parses the given DocString object to a DocString
.DataTable
Model of a Gherkin DocString step argument.
And this is a when step with data table too
| col1 | col2 |
| val1 | val2 |
| val3 | val4 |
{Array<TableRow>} rows
- The rows of the data table.new DataTable(rows) : DataTable
- Creates a new DataTable
object, with the given values.{DataTable}.toString({AssemblerConfig}) : string
- Converts the data table to string, i.e. formats it.{DataTable}.clone() : DataTable
- Clones the data table.DataTable.parse({Object} object) : DataTable
- Parses the given DataTable object to a DataTable
.TableRow
Model of a row in Gherkin DataTable or Examples.
And this is a when step with data table too
| col1 | col2 |
| val1 | val2 |
| val3 | val4 |
{Array<TableCell>} cells
- The cells of the table row.new TableRow(cells) : TableRow
- Creates a new TableRow
object, with the given values.{TableRow}.toString({AssemblerConfig}) : string
- Converts the table row to string, i.e. formats it.{TableRow}.clone() : TableRow
- Clones the table row.TableRow.parse({Object} object) : TableRow
- Parses the given TableRow object to a TableRow
.TableCell
Model of a cell in a Gherkin TableRow.
And this is a when step with data table too
| col1 | col2 |
| val1 | val2 |
| val3 | val4 |
{string} value
- The value of the cell, e.g. "col1"
.new TableCell(value) : TableCell
- Creates a new TableCell
object, with the given values.{TableCell}.toString() : string
- Converts the table cell to string, i.e. formats it.{TableCell}.clone() : TableCell
- Clones the table cell.TableCell.parse({Object} object) : TableCell
- Parses the given TableCell object to a TableCell
.Although this package does not contain method to parse feature file to Gherkin object, you can use the gherkin subpackage of CucumberJS.
'use strict';
const fs = require('fs');
const {Parser} = require('gherkin');
const parser = new Parser();
const document = parser.parse(fs.readFileSync('./login.feature'));
// use objectToAST and format to write it to file again
FAQs
Assembling Gherkin ASTs to feature file string
The npm package gherkin-assembler receives a total of 13,684 weekly downloads. As such, gherkin-assembler popularity was classified as popular.
We found that gherkin-assembler demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Malicious npm packages posing as Telegram bot libraries install SSH backdoors and exfiltrate data from Linux developer machines.
Security News
pip, PDM, pip-audit, and the packaging library are already adding support for Python’s new lock file format.
Product
Socket's Go support is now generally available, bringing automatic scanning and deep code analysis to all users with Go projects.