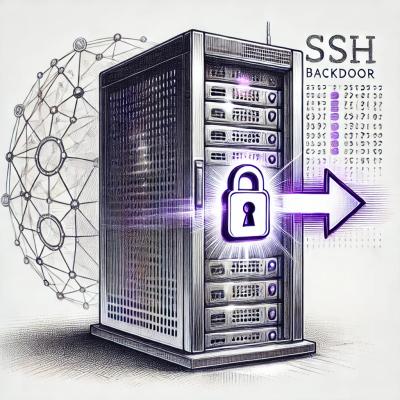
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
GimmeHttp is a library for generating HTTP request code snippets in various languages based on a simple configuration. Whether you need to quickly prototype an API request or generate example code for documentation, GimmeHttp has you covered. Just provide the request details, and let GimmeHttp generate code in your desired language.
Language | Targets |
---|---|
c | libcurl |
csharp | http, restsharp |
curl | native |
go | http |
javascript | fetch, axios, jQuery |
node | http, nod-fetch |
php | curl, guzzle |
python | requests, http |
ruby | nethttp, faraday |
rust | reqwest |
swift | nsurlsession |
To install GimmeHttp, simply use npm:
npm install gimmehttp
Here is a quick example of generating a simple GET request in Go:
import { Generate, Register, AllCodes } from 'gimmehttp';
// Register all codes
Register(AllCodes);
// Create request
const request = {
language: 'go',
target: 'native',
http: {
method: 'GET',
url: 'https://gofakeit.com'
}
};
// Generate output with request
const output = Generate(request);
console.log(output);
Output:
package main
import (
"fmt"
"net/http"
"io"
)
func main() {
url := "https://gofakeit.com"
req, _ := http.NewRequest("GET", url, nil)
resp, _ := http.DefaultClient.Do(req)
defer resp.Body.Close()
body, _ := io.ReadAll(resp.Body)
fmt.Println(string(body))
}
The core functionality of GimmeHttp is its Generate
function. This function takes in a request object and returns the generated code snippet as a string. The request object should have the following structure:
Generate(request: Request): string
interface Request {
language: string // go, javascript, python, etc.
target: string // native, axios, requests, etc.
// HTTP request details
http: {
method: string // 'GET' | 'POST' | 'PUT' | 'PATCH' | 'DELETE'
url: string // ex: 'https://gofakeit.com'
// Optional request details
headers?: { [key: string]: string }
cookies?: { [key: string]: string }
body?: any
}
// Optional - configuration for the code generation
config?: {
// The character(s) to use for indentation
indent?: string // default: ' '
// The character(s) to use for joining lines
join?: string // default: '\n'
// Whether or not to handle errors in the generated code
// default: false to help keep the generated code simple by default
handleErrors?: boolean // default: false
}
}
If you want to register a custom language and/or target, you can do so using the Register
function:
interface Target {
default?: boolean
language: string
target: string
generate: (config: any, http: any) => string
}
import { Generate, Register, Config, Http } from 'gimmehttp';
const myCustomTarget = {
language: 'html',
target: 'href',
generate(config: Config, http: Http): string {
// Custom code generation logic
return `<a href="${http.url}">${http.method}</a>`;
}
};
Register(myCustomTarget);
const request = {
language: 'html',
target: 'href',
http: {
method: 'GET',
url: 'https://gofakeit.com'
}
};
const output = Generate(request);
console.log(output);
Output:
<a href="https://gofakeit.com">GET</a>
const request = {
language: 'javascript',
target: 'fetch',
http: {
method: 'POST',
url: 'https://gofakeit.com',
headers: {
'Content-Type': 'application/json'
},
body: {
key1: 'value1'
}
}
};
const output = Generate(request);
console.log(output);
Output:
fetch("https://gofakeit.com", {
method: "POST",
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({"key1":"value1"}),
})
.then(response => {
if (!response.ok) {
throw new Error("Network response was not ok");
}
return response.text();
})
.then(data => console.log(data));
GimmeHttp is an open-source project that welcomes contributions from the community. If you would like to contribute, please follow these steps:
Feel free to contribute to the project, suggest improvements, or report issues on our GitHub page!
FAQs
HTTP request code generator
The npm package gimmehttp receives a total of 79 weekly downloads. As such, gimmehttp popularity was classified as not popular.
We found that gimmehttp demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.