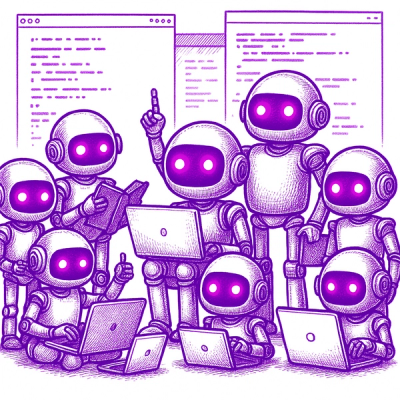
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
A bare-bones applet that handles basic Git commands on common Git-hosting platforms.
A bare-bones applet that handles basic Git commands on common Git-hosting platforms.
Install by running:
npm install -g git-applet
or:
npm install -g https://github.com/NotTimTam/git-applet.git
const GA = require("git-applet");
const gitHandler = new GA(
GA.gitPlatforms.github, // The repository api path for your Git platform. Common ones are stored under GA.gitPlatforms.
"my_example_token", // Your Git platform access token.
"NotTimTam", // The owner of the repository.
"my-repo" // The name of the repository.
);
Create or edit a file with a specific path.
// ...
gitHandler.commitFileAndPush(
"README.md", // The path to commit the file to. Including the file's name and file extension.
"Hello, world!", // The content of the file.
true, // Whether to overwrite an existing file or not.
"Added README.md." // An optional commit message.
);
Check if a file exists in a directory.
// ...
// Returns the SHA of the existing file, or false.
gitHandler.doesFileExist(
"README.md" // The path to the file to check.
);
Get the contents of a file in the repository.
// ...
gitHandler.getFileContents(
"README.md" // The path to the file.
);
Delete an existing file.
// ...
gitHandler.deleteFile(
"README.md", // The path to the file.
"Deleted README.md." // An optional commit message.
);
Rename and/or move a file to a new directory.
// ...
gitHandler.renameFile(
"README.md", // The existing file location.
"old/docs/README.md", // The new file location/name.
"Moved the old README." // An optional commit message.
);
Get a JSON object listing all the files and directories in the repository.
// ...
gitHandler.getFileTree();
Send a custom API request to the Git api.
// ...
gitHandler.apiRequest(
`${gitHandler.repositoryURL}/contents/README.md`, // The sub-path of the repo API url.
"POST", // The API request method.
myData // The data to send with the request.
);
FAQs
A bare-bones applet that handles basic Git commands on common Git-hosting platforms.
The npm package git-applet receives a total of 0 weekly downloads. As such, git-applet popularity was classified as not popular.
We found that git-applet demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.