Comparing version 0.1.14 to 0.2.0
@@ -71,3 +71,3 @@ "use strict"; | ||
function newSubCommand(args) { | ||
const { status, message } = gitCommand('switch', ['-c', ...args]); | ||
const { status, message } = gitSwitch(['--create', ...args]); | ||
state.scene = Scene.Message; | ||
@@ -117,7 +117,7 @@ state.message = message; | ||
} | ||
var ListLineType; | ||
(function (ListLineType) { | ||
ListLineType[ListLineType["Head"] = 0] = "Head"; | ||
ListLineType[ListLineType["Branch"] = 1] = "Branch"; | ||
})(ListLineType || (ListLineType = {})); | ||
var ListItemType; | ||
(function (ListItemType) { | ||
ListItemType[ListItemType["Head"] = 0] = "Head"; | ||
ListItemType[ListItemType["Branch"] = 1] = "Branch"; | ||
})(ListItemType || (ListItemType = {})); | ||
var Scene; | ||
@@ -216,3 +216,3 @@ (function (Scene) { | ||
return list.filter((line) => { | ||
return line.type !== ListLineType.Head; | ||
return line.type !== ListItemType.Head; | ||
}).slice(0, 10); | ||
@@ -250,6 +250,6 @@ } | ||
switch (line.type) { | ||
case ListLineType.Head: { | ||
case ListItemType.Head: { | ||
return viewCurrentHEAD(line.content, layout); | ||
} | ||
case ListLineType.Branch: { | ||
case ListItemType.Branch: { | ||
quickSelectIndex++; | ||
@@ -331,2 +331,3 @@ return viewBranch(line.content, quickSelectIndex, layout); | ||
}, []).map(line => lineSpacer + line), | ||
'', | ||
'' | ||
@@ -347,4 +348,4 @@ ]; | ||
function render(lines) { | ||
process.stdout.write(lines.join('\n') + '\n'); | ||
renderState.cursorY = lines.length + 1; | ||
process.stdout.write(lines.join('\n')); | ||
renderState.cursorY = lines.length; | ||
} | ||
@@ -407,3 +408,3 @@ function cursorTo(x, y) { | ||
return list.slice().sort((a, b) => { | ||
if (b.type === ListLineType.Head) { | ||
if (b.type === ListItemType.Head) { | ||
return 1; | ||
@@ -421,3 +422,3 @@ } | ||
list.push({ | ||
type: ListLineType.Head, | ||
type: ListItemType.Head, | ||
content: state.currentHEAD, | ||
@@ -433,3 +434,3 @@ searchMatchScore: state.searchString === '' ? 1 : fuzzy_1.fuzzyMatch(state.searchString, state.currentHEAD.detached ? state.currentHEAD.sha : state.currentHEAD.branchName) | ||
return { | ||
type: ListLineType.Branch, | ||
type: ListItemType.Branch, | ||
content: branch, | ||
@@ -567,7 +568,7 @@ searchMatchScore: state.searchString === '' ? 1 : fuzzy_1.fuzzyMatch(state.searchString, branch.name) | ||
switch (line.type) { | ||
case ListLineType.Head: { | ||
case ListItemType.Head: { | ||
const content = line.content; | ||
return content.detached ? content.sha : content.branchName; | ||
} | ||
case ListLineType.Branch: { | ||
case ListItemType.Branch: { | ||
return line.content.name; | ||
@@ -579,7 +580,7 @@ } | ||
const commandString = ['git', command, ...args].join(' '); | ||
const { stdout, stderr, error, status } = child_process_1.spawnSync('git', [command, ...args]); | ||
const { stdout, stderr, error, status } = child_process_1.spawnSync('git', [command, ...args], { encoding: 'utf-8' }); | ||
if (error) { | ||
throw new Error(`Could not run ${bold(commandString)}.`); | ||
} | ||
const cleanLines = (stream) => stream.toString().trim().split('\n').filter(line => line !== ''); | ||
const cleanLines = (text) => text.trim().split('\n').filter(line => line !== ''); | ||
const statusIndicatorColor = status > 0 ? red : green; | ||
@@ -591,7 +592,28 @@ const message = [ | ||
]; | ||
return { status, message }; | ||
return { status, message, stdout, stderr }; | ||
} | ||
function switchBranch(branchName, state) { | ||
const switchResult = gitCommand('switch', [branchName]); | ||
if (switchResult.status === 0) { | ||
function chainGitCommands(...commands) { | ||
return commands.reduce((results, command) => { | ||
const result = command(); | ||
results.push(result); | ||
return results; | ||
}, []); | ||
} | ||
function compoundGitCommandsResult(results) { | ||
return results.reduce((compoundResult, result, i) => { | ||
compoundResult.status = result.status; | ||
compoundResult.message = compoundResult.message.concat(result.message); | ||
if (i !== results.length - 1) { | ||
compoundResult.message.push(''); | ||
} | ||
compoundResult.stderr += result.stderr; | ||
compoundResult.stdout += result.stdout; | ||
return compoundResult; | ||
}, { status: 0, message: [], stderr: '', stdout: '' }); | ||
} | ||
function gitSwitch(args) { | ||
const isParameter = (argument) => argument.startsWith('-') || argument.startsWith('--'); | ||
const switchResult = gitCommand('switch', args); | ||
const branchName = args.length === 1 && !isParameter(args[0]) ? args[0] : null; | ||
if (switchResult.status === 0 && branchName !== null) { | ||
updateBranchLastSwitch(branchName, Date.now(), state); | ||
@@ -601,5 +623,19 @@ } | ||
} | ||
function selectListLine(line) { | ||
const branchName = getBranchNameForLine(line); | ||
if (line.type === ListLineType.Head) { | ||
function switchBranch(branchName, state) { | ||
const statusResult = gitCommand('status', ['--porcelain']); | ||
const statusDirty = statusResult.stdout.length > 0; | ||
if (!statusDirty) { | ||
return gitSwitch([branchName]); | ||
} | ||
const results = chainGitCommands(() => gitCommand('stash', ['--include-untracked']), () => gitSwitch([branchName]), () => gitCommand('stash', ['apply'])); | ||
const compoundResult = compoundGitCommandsResult(results); | ||
compoundResult.message = [ | ||
`There are uncommitted changes. Will stash them and apply to ${bold(branchName)} after switch.`, | ||
'' | ||
].concat(compoundResult.message); | ||
return compoundResult; | ||
} | ||
function switchToListItem(item) { | ||
const branchName = getBranchNameForLine(item); | ||
if (item.type === ListItemType.Head) { | ||
state.scene = Scene.Message; | ||
@@ -622,3 +658,3 @@ state.message = [`Staying on ${bold(branchName)}`]; | ||
if (key.equals(ENTER)) { | ||
selectListLine(state.list[state.highlightedLineIndex]); | ||
switchToListItem(state.list[state.highlightedLineIndex]); | ||
return; | ||
@@ -667,3 +703,3 @@ } | ||
if (quickSelectIndex < quickSelectLines.length) { | ||
selectListLine(quickSelectLines[quickSelectIndex]); | ||
switchToListItem(quickSelectLines[quickSelectIndex]); | ||
} | ||
@@ -698,3 +734,3 @@ return; | ||
function jumpTo(args) { | ||
const switchResult = gitCommand('switch', args); | ||
const switchResult = gitSwitch(args); | ||
if (switchResult.status === 0) { | ||
@@ -710,7 +746,7 @@ state.scene = Scene.Message; | ||
state.scene = Scene.Message; | ||
state.message = [`${bold(yellow(state.searchString))} does not mach any branch`]; | ||
state.message = [`${bold(yellow(state.searchString))} does not match any branch`]; | ||
view(state); | ||
process.exit(1); | ||
} | ||
selectListLine(state.list[0]); | ||
switchToListItem(state.list[0]); | ||
} | ||
@@ -717,0 +753,0 @@ function multilineTextLayout(text, columns) { |
@@ -27,10 +27,10 @@ | ||
Creates a new branch and switches into it. | ||
{wrap:6}A proxy to the native "git switch -c <branch name>", so all the standard options are supported.{/wrap} | ||
{wrap:6}A proxy to the native "git switch --create" , so all the standard options are supported.{/wrap} | ||
{wrap:6}See https://git-scm.com/docs/git-switch for the list of options.{/wrap} | ||
{dim}${/dim} git jump delete <branch name> [<branch name>, ...] | ||
{wrap:6}Deletes one or multiple branches. Proxy to the native "git branch --delete <branch name>".{/wrap} | ||
{wrap:6}Deletes one or multiple branches. Proxy to the native "git branch --delete".{/wrap} | ||
{dim}${/dim} git jump rename <branch name> <new branch name> | ||
{wrap:6}Renames local branch. Proxy to the native "git branch --move <branch name> <new branch name>".{/wrap} | ||
{wrap:6}Renames local branch. Proxy to the native "git branch --move".{/wrap} | ||
@@ -37,0 +37,0 @@ |
{ | ||
"name": "git-jump", | ||
"version": "0.1.14", | ||
"version": "0.2.0", | ||
"description": "Git Branches Helper", | ||
@@ -5,0 +5,0 @@ "scripts": { |
@@ -58,3 +58,3 @@ 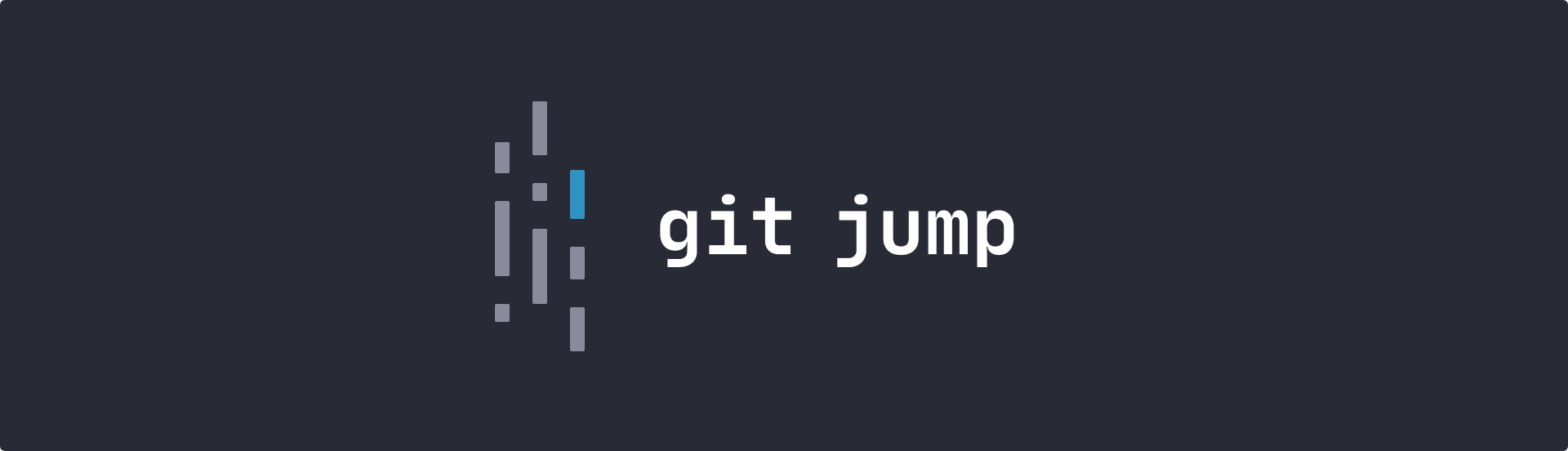 | ||
For example `git jump my-branch --track origin/main --quiet` works just fine. | ||
For example `git jump my-branch --discard-changes` works just fine. | ||
@@ -66,3 +66,3 @@ <br /> | ||
``` | ||
Creates a new branch and switches into it. | ||
Creates a new branch and switches into it. Supports all native parameters of `git switch`, for example `git jump new <branch name> --track origin/main`. | ||
@@ -69,0 +69,0 @@ <br /> |
Sorry, the diff of this file is not supported yet
43134
973