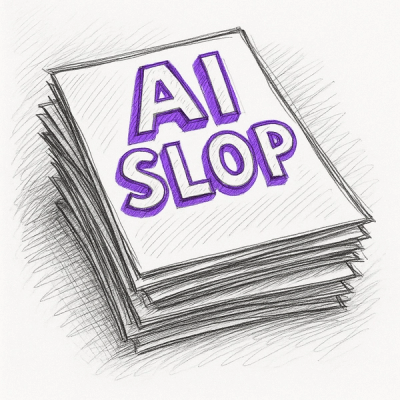
Security News
Django Joins curl in Pushing Back on AI Slop Security Reports
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
git-revision-webpack-plugin
Advanced tools
Simple webpack plugin that generates VERSION and COMMITHASH files during build
Simple webpack plugin that generates VERSION
and COMMITHASH
files during build based on a local git repository.
Given a webpack project, install it as a local development dependency:
npm install --save-dev git-revision-webpack-plugin
Then, simply configure it as a plugin in the webpack config:
var GitRevisionPlugin = require('git-revision-webpack-plugin')
module.exports = {
plugins: [
new GitRevisionPlugin()
]
}
It outputs a VERSION
based on git-describe such as:
v0.0.0-34-g7c16d8b
And a COMMITHASH
such as:
7c16d8b1abeced419c14eb9908baeb4229ac0542
The plugin requires no configuration by default, but it is possible to configure it to support custom git workflows.
lightweightTags: false
If you need lightweight tags support, you may turn on lighweithTags
option in this way:
var GitRevisionPlugin = require('git-revision-webpack-plugin')
module.exports = {
plugins: [
new GitRevisionPlugin({
lightweightTags: true
})
]
}
commithashCommand: 'rev-parse HEAD'
To change the default git
command used to read the value of COMMITHASH
:
var GitRevisionPlugin = require('git-revision-webpack-plugin')
module.exports = {
plugins: [
new GitRevisionPlugin({
commithashCommand: 'rev-list --max-count=1 --no-merges HEAD'
})
]
}
versionCommand: 'describe --always'
To change the default git
command used to read the value of VERSION
:
var GitRevisionPlugin = require('git-revision-webpack-plugin')
module.exports = {
plugins: [
new GitRevisionPlugin({
versionCommand: 'describe --always --tags --dirty'
})
]
}
It is also possible to use two path substituitions on build to get either the revision or version as part of output paths.
[git-revision-version]
[git-revision-hash]
Example:
module.exports = {
output: {
publicPath: 'http://my-fancy-cdn.com/[git-revision-version]/',
filename: '[name]-[git-revision-hash].js'
}
}
The VERSION
and COMMITHASH
are also exposed through a public API.
Example using the DefinePlugin:
var gitRevisionPlugin = new GitRevisionPlugin()
module.exports = {
plugins: [
new DefinePlugin({
'VERSION': JSON.stringify(gitRevisionPlugin.version()),
'COMMITHASH': JSON.stringify(gitRevisionPlugin.commithash()),
})
]
}
FAQs
[](https://badge.fury.io/js/git-revision-webpack-plugin) [](https://www.npmjs.com/package/
The npm package git-revision-webpack-plugin receives a total of 151,807 weekly downloads. As such, git-revision-webpack-plugin popularity was classified as popular.
We found that git-revision-webpack-plugin demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.