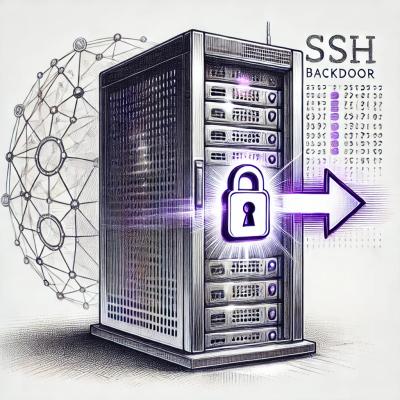
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
glitched-writer
Advanced tools
Glitched, text writing module. Highly customizable settings. Decoding, decrypting, scrambling, and simply spelling text.
A lightweight, glitched, text writing module. Highly customizable settings. Decoding, decrypting, scrambling, and simply spelling text. For web and node.
Writes your text, by glitching or spelling it out.
Highly customizable behavior. Set of options will help you achieve the effect you desire.
Can be attached to a HTML Element for automatic text-displaying.
Callback functions firing on finish and every step.
Custom Event gw-finished are dispatched on the HTML Element.
For styling purposes writer attatches gw-writing class to the HTML Element and data-gw-string attribute with current string.
Possible to write text with html tags in it or letterize string into many span elements.
Written in Typescript.
Download package through npm.
npm i glitched-writer
Then import GlitchedWriter class in the JavaScript file.
import GlitchedWriter from 'glitched-writer'
Or use the CDN and attach this script link to your html document.
<script src="https://cdn.jsdelivr.net/npm/glitched-writer@2.0.10/lib/index.min.js"></script>
Creating writer class instance:
// Calling GlitchedWriter constructor:
const Writer = new GlitchedWriter(
htmlElement, // Element or Selector string
options, // {...} or Preset name
onStepCallback, // (string, data) => {}
onFinishCallback, // (string, data) => {}
)
// Custom options:
const Writer = new GlitchedWriter(htmlElement, {
interval: [10, 70],
oneAtATime: true,
letterize: true,
})
// On-step-callback added:
const Writer = new GlitchedWriter(htmlElement, {}, (string, writerData) => {
console.log(`Current string: ${string}`)
console.log('All the class data:', writerData)
})
Writing stuff and waiting with async / await.
import { wait } from 'glitched-writer'
// Wrap this in some async function:
// Or use .then() instead.
const res = await Writer.write('Welcome')
console.log(`Finished writing: ${res.string}`)
console.log('All the writer data:', res)
await wait(1200) // additional simple promise to wait some time
await Writer.write('...to Glitch City!')
Writer.write('Some very cool header.').then(({ status, message }) => {
// this will run when the writing stops.
console.log(`${status}: ${message}`)
})
setTimeout(() => {
Writer.pause() // will stop writing
}, 1000)
setTimeout(async () => {
await Writer.play() // continue writing
console.log(Writer.string) // will log after finished writing
}, 2000)
For quick one-time writing.
import { glitchWrite } from 'glitched-writer'
glitchWrite('Write this and DISAPER!', htmlElement, options, ...)
Don't be afraid to call write method on top of each oder. New will stop the ongoing.
inputEl.addEventListener('input', () => {
Writer.write(inputEl.value)
})
// html element that you passed in writer constructor.
textHtmlElement.addEventListener('gw-finished', e =>
console.log('finished writing:', e.detail.string),
)
.add(string) & .remove(number) are methods usefull for quick, slight changes to the displayed text.
// Let's say current text content is: "Hello World"
Writer.add('!!!')
// -> Hello World!!!
Writer.remove(9)
// -> Hello
(! experimental & potentially dangerous !) Let's you write text with html tags in it. Can be enabled along with "letterize" option, but the "double tags", like ... won't show up.
// You need to enable html option.
const Writer = new GlitchedWriter(htmlElement, { html: true })
Writer.write('<b>Be sure to click <a href="...">this!</a></b>')
(Experimental!) Splits written text into series of elements. Then writing letters seperately into these child-elements.
// You need to enable html option.
const Writer = new GlitchedWriter(htmlElement, { letterize: true })
Writer.write('Hello there!')
/**
* The shape of one character:
* span.gw-char (state classes: .gw-typing or .gw-finished)
* span.gw-ghosts
* span.gw-letter (also .gw-glitched when it is a "glitched" char.)
* span.gw-ghosts
*/
List of all things that can be imported from glitched-writer module.
import GlitchedWriter, { // <-- GlitchedWriter class
ConstructorOptions, // <-- Options type
Callback, // <-- Callback type
WriterDataResponse, // <-- Type of response in callbacks
glitchWrite, // <-- One time write funcion
presets, // <-- Object with all prepared presets of options
glyphs, // <-- Same but for glyph charsets
wait, // <-- Ulitity async function, that can be used to wait some time
} from 'glitched-writer'
To use one of the available presets, You can simply write it's name when creating writer, in the place of options. Available presets as for now:
default - Loaded automatically, featured on the GIF up top.
nier - Imitating the way text was appearing in the NieR: Automata's UI.
typewriter - One letter at a time, only slightly glitched.
terminal - Imitating being typed by a machine or a computer.
zalgo - Inspired by the "zalgo" or "cursed text", Ghost characters mostly includes the unicode combining characters, which makes the text glitch vertically.
neo - Recreated: Justin Windle's "Text Scramble Effect"
new GlitchedWriter(htmlElement, 'nier')
You can import the option object of mentioned presets and tweak them, as well as some glyph sets.
import { presets, glyphs } from 'glitched-writer'
new GlitchedWriter(htmlElement, presets.typewriter)
{
// name [min , max ] | const // default
steps?: [number, number] | number, // [1, 8]
interval?: [number, number] | number, // [60, 170]
initialDelay?: [number, number] | number, // [0, 2000]
changeChance?: number, // 0.6
ghostChance?: number, // 0.2
maxGhosts?: number, // '0.2'
glyphs?: string | string[] | Set<string>, // glyphs.full + glyphs.zalgo
glyphsFromString?: boolean, // false
startFrom?: 'matching' | 'previous' | 'erase', // 'matching'
oneAtATime?: boolean, // false
html?: boolean, // false
letterize?: boolean, // false
fillSpace?: boolean // true
}
Range values will result in random values for each step for every letter.
Ghost are "glitched letters" that gets rendered randomly in the time of writing, but aren't part of final string.
steps - Number of minimum steps it takes one letter to reach it's goal one. Set to 0 if you want them to change to right letter in one step. (int)
interval - Interval between each step, for every letter. (int: ms)
initialDelay - first delay each letter must wait before it starts working (int: ms)
changeChance - Percentage chance for letter to change to glitched one (from glyphs) (p: 0-1)
ghostChance - Percentage chance for ghost letter to appear (p: 0-1)
maxGhosts - Maximal number of ghosts to occur
glyphs - A set of characters that can appear as ghosts or letters can change into them
glyphsFromString - If you want to add letters from written string to the glyph charset
startFrom - Decides on witch algorithm to use.
oneAtATime - If writing should take place from left-to-right, letter-by-letter or normally: all-at-once.
html - Potentially dangerous option. If true, written string will be injected as html, not text content. It provides advanced text formating with html tags and more. But be sure to not enable it on user-provided content.
letterize - Experimental option. Instead of injecting written text to "textContent" or "innerHTML", it appends every letter of that text as a child element. Then changing textContent of that span to current letter. It gives a lot of styling possibilities, as you can style ghosts, letters, and whole chars seperately, depending on current writer and char state.
fillSpace - Normally if letter gets erased it actually gets replaced with space instead - to make words appear from and disappear into space, rather then sticking to the rest of the words. Set to false if you want to disable this.
FAQs
Glitched, text writing module. Highly customizable settings. Decoding, decrypting, scrambling, or simply spelling out text.
The npm package glitched-writer receives a total of 46 weekly downloads. As such, glitched-writer popularity was classified as not popular.
We found that glitched-writer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.