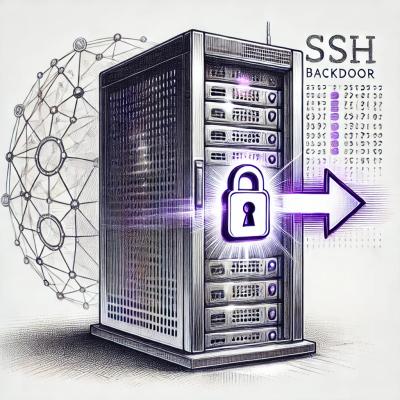
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
glitched-writer
Advanced tools
Glitched text-writer module, with highly customizable settings to get the effect You're looking for. Generally for web as a client dependency, but can be also used elswere.
Glitched, text-writing npm module, with highly customizable settings to get the effect You're looking for. Works for both web and node.js applications.
Writes your text, by glitching or spelling it out.
Highly customizable behavior. Set of options will help you achieve the effect you desire.
Can be attached to a HTML Element for automatic text-displaying.
Callback functions for every step and finish.
Events gw_finished and gw_step are dispatched on the HTML Element.
For styling purposes, while writing: attatches glitched-writer--writing class to the HTML Element and data-string attribute with current string state.
Written in Typescript.
Download package through npm.
npm i glitched-writer
Then import GlitchedWriter class in the JavaScript file.
import GlitchedWriter from 'glitched-writer'
Or use the CDN and attach this script link to your html document.
<script src="https://cdn.jsdelivr.net/npm/glitched-writer@2.0.0/lib/index.min.js"></script>
Creating writer class instance:
// Calling GlitchedWriter constructor:
const Writer = new GlitchedWriter(htmlElement, options, onStepCallback, onFinishCallback)
// Custom options:
const Writer = new GlitchedWriter(htmlElement, {
interval: [10, 70],
oneAtATime: true
})
// On-step-callback added:
const Writer = new GlitchedWriter(htmlElement, undefined, (string, writerData) => {
console.log(`Current string: ${string}`)
console.log('All the class data:', writerData)
})
// Using alternative class-creating function:
import { createGlitchedWriter } from 'glitched-writer'
const Writer = createGlitchedWriter(htmlElement, ...)
Writing stuff and waiting with async / await.
import { wait } from 'glitched-writer'
// Wrap this in some async function:
// Or use .then() instead.
const res = await Writer.write('Welcome')
console.log(`Finished writing: ${res.string}`)
console.log('All the writer data:', res)
await wait(1200) // additional simple promise to wait some time
await Writer.write('...to Glitch City!')
Don't be afraid to call write method on top of each oder. Newer will stop the ongoing one.
inputEl.addEventListener('input', () => {
Writer.write(inputEl.value)
})
Writer.write('Some very cool header.').then(({ status, message }) => {
// this will run when the writing stops.
console.log(`${status}: ${message}`)
})
setTimeout(() => {
Writer.pause() // will stop writing
}, 1000)
setTimeout(async () => {
await Writer.play() // continue writing
console.log(Writer.string) // will log after finished writing
}, 2000)
For quick one-time writing.
import { glitchWrite } from 'glitched-writer'
glitchWrite('Write this and DISAPER!', htmlElement, options, ...)
textHtmlElement.addEventListener('gw_finished', e =>
console.log('finished writing:', e.detail.string),
)
textHtmlElement.addEventListener('gw_step', e =>
console.log('current step:', e.detail.string),
)
New (experimental & potentially dangerous) config option let's you write text with html tags in it.
// You need to enable html option.
const Writer = new GlitchedWriter(htmlElement, { html: true })
Writer.write('<b>Be sure to click <a href="...">this!</a></b>')
List of all things that can be imported from glitched-writer module.
import GlitchedWriter, { // <-- GlitchedWriter class
ConstructorOptions, // <-- Options type
Callback, // <-- Callback type
WriterDataResponse, // <-- Type of response in callbacks
createGlitchedWriter, // <-- Alternative to creating writer class instance
glitchWrite, // <-- One time write funcion
presets, // <-- Object with all prepared presets of options
glyphs, // <-- Same but for glyph charsets
wait, // <-- Ulitity async function, that can be used to wait some time
} from 'glitched-writer'
To use one of the available presets, You can simply write it's name when creating writer, in the place of options. Available presets as for now:
new GlitchedWriter(htmlElement, 'nier')
You can import the option object of mentioned presets and tweak them, as well as some glyph sets.
import { presets, glyphs } from 'glitched-writer'
new GlitchedWriter(htmlElement, presets.typewriter)
{
steps?: RangeOrNumber, // [1, 6]
interval?: RangeOrNumber, // [50, 150]
initialDelay?: RangeOrNumber, // [0, 1500]
changeChance?: RangeOrNumber, // 0.6
ghostChance?: RangeOrNumber, // 0.15
maxGhosts?: number | 'relative', // 'relative'
glyphs?: string | string[] | Set<string>, // glyphs.full
glyphsFromString?: 'previous' | 'goal' | 'both' | 'none', // 'none'
oneAtATime?: boolean, // false
html?: boolean, // false
startFrom?: 'matching' | 'previous' | 'erase', // 'matching'
leadingText?: AppendedText, // undefined
trailingText?: AppendedText // undefined
}
interface AppendedText {
value: string
display: 'always' | 'when-typing' | 'when-not-typing'
}
type RangeOrNumber = [number, number] | number
Range values will result in random values for each step for every letter.
Ghost are letters that gets rendered in the time of writing, but are removed to reach goal string.
FAQs
Glitched, text writing module. Highly customizable settings. Decoding, decrypting, scrambling, or simply spelling out text.
The npm package glitched-writer receives a total of 46 weekly downloads. As such, glitched-writer popularity was classified as not popular.
We found that glitched-writer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.