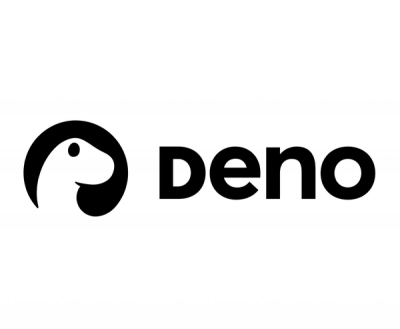
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
glitched-writer
Advanced tools
Glitched text-writer module, with highly customizable settings to get the effect You're looking for. Generally for web as a client dependency, but can be also used elswere.
Glitched, text-writing npm module, with highly customizable settings to get the effect You're looking for. Works for both web and node.js applications.
Writes your text, by glitching or spelling it out.
Highly customizable behavior. Set of options will help you achieve the effect you desire.
Can be attached to a HTML Element for automatic text-displaying.
Callback functions for every step and finish.
Events gw-finished and gw-step are dispatched on the HTML Element.
For styling purposes, while writing: attatches gw-writing class to the HTML Element and data-gw-string attribute with current string state.
Written in Typescript.
Download package through npm.
npm i glitched-writer
Then import GlitchedWriter class in the JavaScript file.
import GlitchedWriter from 'glitched-writer'
Or use the CDN and attach this script link to your html document.
<script src="https://cdn.jsdelivr.net/npm/glitched-writer@2.0.6/lib/index.min.js"></script>
Creating writer class instance:
// Calling GlitchedWriter constructor:
const Writer = new GlitchedWriter(htmlElement, options, onStepCallback, onFinishCallback)
// Custom options:
const Writer = new GlitchedWriter(htmlElement, {
interval: [10, 70],
oneAtATime: true
})
// On-step-callback added:
const Writer = new GlitchedWriter(htmlElement, undefined, (string, writerData) => {
console.log(`Current string: ${string}`)
console.log('All the class data:', writerData)
})
// Using alternative class-creating function:
import { createGlitchedWriter } from 'glitched-writer'
const Writer = createGlitchedWriter(htmlElement, ...)
Writing stuff and waiting with async / await.
import { wait } from 'glitched-writer'
// Wrap this in some async function:
// Or use .then() instead.
const res = await Writer.write('Welcome')
console.log(`Finished writing: ${res.string}`)
console.log('All the writer data:', res)
await wait(1200) // additional simple promise to wait some time
await Writer.write('...to Glitch City!')
Don't be afraid to call write method on top of each oder. Newer will stop the ongoing one.
inputEl.addEventListener('input', () => {
Writer.write(inputEl.value)
})
Writer.write('Some very cool header.').then(({ status, message }) => {
// this will run when the writing stops.
console.log(`${status}: ${message}`)
})
setTimeout(() => {
Writer.pause() // will stop writing
}, 1000)
setTimeout(async () => {
await Writer.play() // continue writing
console.log(Writer.string) // will log after finished writing
}, 2000)
For quick one-time writing.
import { glitchWrite } from 'glitched-writer'
glitchWrite('Write this and DISAPER!', htmlElement, options, ...)
textHtmlElement.addEventListener('gw_finished', e =>
console.log('finished writing:', e.detail.string),
)
textHtmlElement.addEventListener('gw_step', e =>
console.log('current step:', e.detail.string),
)
New (experimental & potentially dangerous) config option let's you write text with html tags in it.
// You need to enable html option.
const Writer = new GlitchedWriter(htmlElement, { html: true })
Writer.write('<b>Be sure to click <a href="...">this!</a></b>')
List of all things that can be imported from glitched-writer module.
import GlitchedWriter, { // <-- GlitchedWriter class
ConstructorOptions, // <-- Options type
Callback, // <-- Callback type
WriterDataResponse, // <-- Type of response in callbacks
createGlitchedWriter, // <-- Alternative to creating writer class instance
glitchWrite, // <-- One time write funcion
presets, // <-- Object with all prepared presets of options
glyphs, // <-- Same but for glyph charsets
wait, // <-- Ulitity async function, that can be used to wait some time
} from 'glitched-writer'
To use one of the available presets, You can simply write it's name when creating writer, in the place of options. Available presets as for now:
new GlitchedWriter(htmlElement, 'nier')
You can import the option object of mentioned presets and tweak them, as well as some glyph sets.
import { presets, glyphs } from 'glitched-writer'
new GlitchedWriter(htmlElement, presets.typewriter)
{
steps?: RangeOrNumber, // [1, 8]
interval?: RangeOrNumber, // [60, 170]
initialDelay?: RangeOrNumber, // [0, 2000]
changeChance?: RangeOrNumber, // 0.6
ghostChance?: RangeOrNumber, // 0.2
maxGhosts?: number, // '0.2'
glyphs?: string | string[] | Set<string>, // glyphs.full + glyphs.zalgo
glyphsFromString?: boolean // false
startFrom?: 'matching' | 'previous' | 'erase', // 'matching'
oneAtATime?: boolean, // false
html?: boolean, // false
fillSpace?: boolean // true,
}
type RangeOrNumber = [number, number] | number
Range values will result in random values for each step for every letter.
Ghost are letters that gets rendered in the time of writing, but are removed to reach goal string.
FAQs
Glitched, text writing module. Highly customizable settings. Decoding, decrypting, scrambling, or simply spelling out text.
The npm package glitched-writer receives a total of 63 weekly downloads. As such, glitched-writer popularity was classified as not popular.
We found that glitched-writer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.