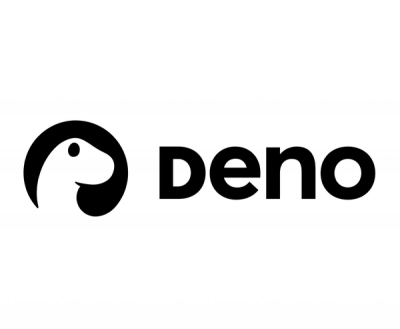
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Gotu Kola helps define your business entities (*)
(*) Entities: they are the first natural place we should aim to place business logic in domain-driven applications.
$ npm install gotu
const User =
entity('User', {
name: field(String),
lastAccess: field(Date),
accessCount: field(Number),
hasAccess: field(Boolean),
plan: field(Plan),
})
const user = new User()
user.name = "Beth"
user.plan.monthlyCost = 10
user.validate()
A value of an field can be validated against the field type or its custom validation.
const Plan =
entity('Plan', {
...
monthlyCost: field(Number),
})
const User =
entity('User', {
name: field(String),
plan: field(Plan)
})
const user = new User()
user.name = 42
user.plan.monthlyCost = true
user.validate()
user.errors // { name: ["name must be of type string"], plan: { monthlyCost: ["monthlyCost must be of type number"] } }
user.isValid // false
For custom validation Gotu uses validate.js library under the hood.
Use { validation: ... }
to specify a valid validate.js validation (sorry) on the field definition.
const User =
entity('User', {
...
password: field(String, {
presence: true,
length: { minimum: 6 },
message: "must be at least 6 characters"
})
})
const user = new User()
user.password = '1234'
user.validate()
user.errors // { password: ["Password must be at least 6 characters"] }
user.isValid // false
fromJSON(value)
Returns a new instance of a entity
const User =
entity('User', {
name: field(String)
})
// from hash
const user = User.fromJSON({ name: 'Beth'})
// or string
const user = User.fromJSON(`{ "name": "Beth"}`)
JSON.stringify(entity)
To serialize an entity to JSON string use JSON.stringify
function.
const json = JSON.stringify(user) // { "name": "Beth"}
A entity field type has a name, type, default value, validation and more.
A field in an entity can be of basic types, the same as those used by JavaScript:
Number
: double-precision 64-bit binary format IEEE 754 value
String
: a UTF‐16 character sequence
Boolean
: true or false
Date
: represents a single moment in time in a platform-independent format.
const User =
entity('User', {
name: field(String),
lastAccess: field(Date),
accessCount: field(Number),
hasAccess: field(Boolean)
})
For complex types, with deep relationship between entities, a field can be of entity type:
const Plan =
entity('Plan', {
...
monthlyCost: field(Number),
})
const User =
entity('User', {
...
plan: field(Plan)
})
It is possible to define a default value when an entity instance is initiate.
const User =
entity('User', {
...
hasAccess: field(Boolean, { default: false })
})
const user = new User()
user.hasAccess // false
If the default value is a function
it will call the function and return the value as default value:
const User =
entity('User', {
...
hasAccess: field(Boolean, { default: () => false })
})
const user = new User()
user.hasAccess // false
For scalar types a default value is assumed if a default value is not given:
Type | Default Value |
---|---|
Number | 0 |
String | "" |
Boolean | false |
Date | null |
For entity types the default value is a new instance of that type. It is possible to use null
as default:
const User =
entity('User', {
...
plan: field(Plan, { default: null })
})
const user = new User()
user.plan // null
A method can be defined to create custom behaviour in an entity:
const User =
entity('User', {
...
role: field(String),
hasAccess() { return this.role === "admin" },
})
const user = new User()
const access = user.hasAccess()
FAQs
Domain entities javascript library.
The npm package gotu receives a total of 3 weekly downloads. As such, gotu popularity was classified as not popular.
We found that gotu demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.