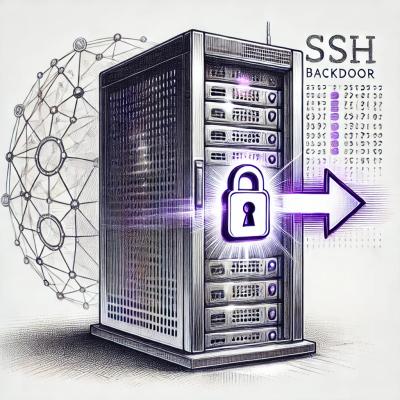
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
gql-payload
Advanced tools
This is an optimized fork of gql-query-builder with extra features for generating GraphQL payloads using plain JavaScript Objects (JSON).
npm install gql-payload --save
or yarn add gql-payload
import { gqlQuery, gqlMutation, gqlSubscription } from 'gql-payload'
const query = gqlQuery(options: object)
const mutation = gqlMutation(options: object)
const subscription = gqlSubscription(options: object)
options
is { operation, fields, variables }
or an array of options
Name | Description | Type | Required | Example |
---|---|---|---|---|
operation | Name of operation to be executed on server | String | Object | Yes |
getThoughts, createThought
{ name: 'getUser', alias: 'getAdminUser' }
|
fields | Selection of fields | Array | No |
['id', 'name', 'thought']
['id', 'name', 'thought', { user: ['id', 'email'] }]
|
variables | Variables sent to the operation | Object | No |
{ key: value } eg: { id: 1 }
{ key: { value: value, required: true, type: GQL type, list: true, name: argument name } eg: {
email: { value: "user@example.com", required: true },
password: { value: "123456", required: true },
secondaryEmails: { value: [], required: false, type: 'String', list: true, name: secondaryEmail }
}
|
An optional third argument adapter
is a typescript/javascript class that implements IQueryAdapter
, IMutationAdapter
or ISubscriptionAdapter
.
If adapter is undefined then src/adapters/DefaultQueryAdapter
or src/adapters/DefaultMutationAdapter
is used.
import { gqlQuery } as gql from 'gql-payload'
const query = gqlQuery(options: object, adapter?: MyCustomQueryAdapter,config?: object)
Name | Description | Type | Required | Example |
---|---|---|---|---|
operationName | Name of operation to be sent to the server | String | No | getThoughts, createThought |
fragment | Named fragment config for reusable fields to be sent to the server | Array | No |
[{
name: "NamedFragment",
on: "User",
fields: ["grade"]
}]
|
import { gqlQuery } from "gql-payload";
const query = gqlQuery({
operation: "thoughts",
fields: ["id", "name", "thought"]
});
console.log(query);
Output:
{
query: 'query {thoughts { id name thought } }',
variables: {}
}
import { gqlQuery } from "gql-payload";
const query = gqlQuery({
operation: "thought",
variables: { id: 1 },
fields: ["id", "name", "thought"]
});
console.log(query)
Output:
{
query: 'query ($id: Int) { thought (id: $id) { id name thought } }',
variables: { id: 1 }
}
import { gqlQuery } from "gql-payload";
const query = gqlQuery({
operation: "orders",
fields: [
"id",
"amount",
{
user: [
"id",
"name",
"email",
{
address: [
"city",
"country"
]
}
]
}
]
});
console.log(query);
Output:
{
query: 'query { orders { id amount user { id name email address { city country } } } }',
variables: {}
}
import { gqlQuery } from "gql-payload";
const query = gqlQuery({
operation: "userLogin",
variables: {
email: { value: "jon.doe@example.com", required: true },
password: { value: "123456", required: true }
},
fields: ["userId", "token"]
});
console.log(query);
Output:
{
query: 'query ($email: String!, $password: String!) { userLogin (email: $email, password: $password) { userId token } }',
variables: { email: 'jon.doe@example.com', password: '123456' }
}
import { gqlQuery } from "gql-payload";
const query = gqlQuery([{
operation: "someoperation",
fields: [{
operation: "nestedoperation",
fields: ["field1"],
variables: {
id2: {
name: "id",
type: "ID",
value: 123
}
}
}],
variables: {
id1: {
name: "id",
type: "ID",
value: 456
}
}
}]);
console.log(query);
Output:
{
query: 'query ($id2: ID, $id1: ID) { someoperation (id: $id1) { nestedoperation (id: $id2) { field1 } } }',
variables: { id1: 456, id2: 123 }
}
import { gqlQuery } from "gql-payload";
const query = gqlQuery({
operation: "userLogin",
fields: ["userId", "token"]
}, {
operationName: "someoperation"
});
console.log(query);
Output:
{
query: 'query someoperation { userLogin { userId token } }',
variables: {}
}
import { gqlQuery } from "gql-payload";
const query = gqlQuery([
{
operation: "getFilteredUsersCount"
},
{
operation: "getAllUsersCount",
fields: []
},
{
operation: "getFilteredUsers",
fields: [{ count: [] }]
}
]);
console.log(query);
Output:
{
query: 'query { getFilteredUsersCount getAllUsersCount getFilteredUsers { count } }',
variables: {}
}
import { gqlQuery } from "gql-payload";
const query = gqlQuery({
operation: {
name: "thoughts",
alias: "myThoughts"
},
fields: ["id", "name", "thought"]
});
console.log(query);
Output:
{
query: 'query { myThoughts: thoughts { id name thought } }',
variables: {}
}
import { gqlQuery } from "gql-payload";
const query = gqlQuery({
operation: "thought",
fields: [
"id",
"name",
"thought",
{
operation: "FragmentType",
fields: ["emotion"],
inlineFragment: true
}
]
});
console.log(query);
Output:
{
query: 'query { thought { id name thought ... on FragmentType { emotion } } }',
variables: {}
}
import { gqlQuery } from "gql-payload";
const query = gqlQuery({
operation: "thought",
fields: [
"id",
"name",
"thought",
{
operation: "FragmentName",
namedFragment: true
}
]
}, {
fragments: [
{
name: "FragmentName",
on: "FragmentType",
fields: ["emotion"]
}
]
});
console.log(query);
Output:
{
query: 'query { thought { id name thought ...FragmentName } } fragment FragmentName on FragmentType { emotion }',
variables: {}
}
For example, to inject SomethingIDidInMyAdapter
in the operationWrapperTemplate
method.
import { gqlQuery } from "gql-payload";
import MyQueryAdapter from "where/adapters/live/MyQueryAdapter";
const query = gqlQuery({
operation: "thoughts",
fields: ["id", "name", "thought"]
}, null, MyQueryAdapter);
console.log(query);
Output:
{
query: 'query SomethingIDidInMyAdapter { thoughts { id name thought } }',
variables: {}
}
Take a peek at DefaultQueryAdapter to get an understanding of how to make a new adapter.
import { gqlMutation } from "gql-payload";
const mutation = gqlMutation({
operation: "thoughtCreate",
variables: {
name: "Tyrion Lannister",
thought: "I drink and I know things."
},
fields: ["id"]
});
console.log(mutation);
Output:
{
query: 'mutation ($name: String, $thought: String) { thoughtCreate (name: $name, thought: $thought) { id } }',
variables: { name: 'Tyrion Lannister', thought: 'I drink and I know things.' }
}
import { gqlMutation } from "gql-payload";
const mutation = gqlMutation({
operation: "userSignup",
variables: {
name: { value: "Jon Doe" },
email: { value: "jon.doe@example.com", required: true },
password: { value: "123456", required: true }
},
fields: ["userId"]
});
console.log(mutation);
Output:
{
query: 'mutation ($name: String, $email: String!, $password: String!) { userSignup (name: $name, email: $email, password: $password) { userId } }',
variables: { name: 'Jon Doe', email: 'jon.doe@example.com', password: '123456' }
}
import { gqlMutation } from 'gql-payload'
const mutation = gqlMutation({
operation: "userPhoneNumber",
variables: {
phone: {
value: { prefix: "+91", number: "9876543210" },
type: "PhoneNumber",
required: true
}
},
fields: ["id"]
})
console.log(mutation)
Output:
{
query: 'mutation ($phone: PhoneNumber!) { userPhoneNumber (phone: $phone) { id } }',
variables: { phone: { prefix: '+91', number: '9876543210' } }
}
For example, to inject SomethingIDidInMyAdapter
in the operationWrapperTemplate
method.
import { gqlMutation } from "gql-payload";
import MyMutationAdapter from "where/adapters/live/MyMutationAdapter";
const mutation = gqlMutation({
operation: "thoughts",
fields: ["id", "name", "thought"]
}, null, MyMutationAdapter);
console.log(mutation);
Output:
{
query: 'mutation SomethingIDidInMyAdapter { thoughts { id name thought } }',
variables: {}
}
Take a peek at DefaultMutationAdapter to get an understanding of how to make a new adapter.
import { gqlMutation } from "gql-payload";
const mutation = gqlMutation({
operation: "thoughts",
fields: ["id", "name", "thought"]
}, {
operationName: "someoperation"
});
console.log(mutation);
Output:
{
query: 'mutation someoperation { thoughts { id name thought } }',
variables: {}
}
import { gqlSubscription } from "gql-payload";
const subscription = gqlSubscription({
operation: "thoughtCreate",
variables: {
name: "Tyrion Lannister",
thought: "I drink and I know things."
},
fields: ["id"]
});
console.log(subscription);
Output:
{
query: 'subscription ($name: String, $thought: String) { thoughtCreate (name: $name, thought: $thought) { id } }',
variables: { name: 'Tyrion Lannister', thought: 'I drink and I know things.' }
}
For example, to inject SomethingIDidInMyAdapter
in the operationWrapperTemplate
method.
import { gqlSubscription } from "gql-payload";
import MySubscriptionAdapter from "where/adapters/live/MySubscriptionAdapter";
const subscription = gqlSubscription({
operation: "thoughts",
fields: ["id", "name", "thought"]
}, null, MySubscriptionAdapter);
console.log(subscription);
Output:
{
query: 'subscription SomethingIDidInMyAdapter { thoughts { id name thought } }',
variables: {}
}
Take a peek at DefaultSubscriptionAdapter to get an understanding of how to make a new adapter.
import { gqlQuery } from "gql-payload";
async function getThoughts () {
const query = gqlQuery({
operation: "thoughts",
fields: ["id", "name", "thought"]
});
try {
const response = await fetch("http://api.example.com/graphql", {
method: "POST",
headers: {
"Content-Type": "application/json"
},
body: JSON.stringify(query)
});
const data = await response.json();
console.log(data);
}
catch (error) {
console.log(error);
}
}
import { $fetch } from "ofetch";
import { gqlQuery } from "gql-payload";
async function getThoughts () {
const query = gqlQuery({
operation: "thoughts",
fields: ["id", "name", "thought"]
});
const data = await $fetch("http://api.example.com/graphql", {
method: "POST",
headers: {
"Content-Type": "application/json"
},
body: query
}).catch((error) => console.log(error));
console.log(data);
}
import axios from "axios";
import { gqlQuery } from "gql-payload";
async function getThoughts () {
try {
const response = await axios.post(
"http://api.example.com/graphql",
gqlQuery({
operation: "thoughts",
fields: ["id", "name", "thought"]
})
);
console.log(response);
}
catch (error) {
console.log(error);
}
}
FAQs
GraphQL Payload Builder
The npm package gql-payload receives a total of 13 weekly downloads. As such, gql-payload popularity was classified as not popular.
We found that gql-payload demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.