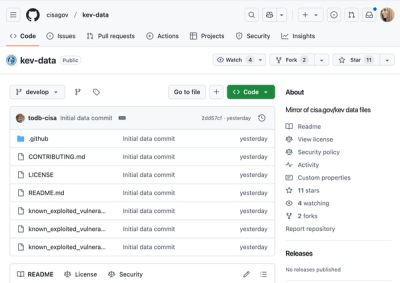
Security News
CISA Brings KEV Data to GitHub
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.
graceful-readlink
Advanced tools
The graceful-readlink npm package provides a way to read symbolic links in a manner that gracefully handles errors and edge cases. It is designed to be a more robust alternative to the standard fs.readlink method in Node.js.
Reading Symbolic Links
This feature allows you to read the target of a symbolic link synchronously. The code sample demonstrates how to use the graceful-readlink package to read a symbolic link and print its target path.
const readlink = require('graceful-readlink');
const linkPath = '/path/to/symlink';
const targetPath = readlink.sync(linkPath);
console.log(targetPath);
Graceful Error Handling
This feature ensures that errors are handled gracefully when reading symbolic links. The code sample shows how to use a try-catch block to handle potential errors that may occur during the read operation.
const readlink = require('graceful-readlink');
const linkPath = '/path/to/symlink';
try {
const targetPath = readlink.sync(linkPath);
console.log(targetPath);
} catch (err) {
console.error('Error reading symbolic link:', err);
}
The fs-extra package extends the native Node.js fs module with additional methods, including readlink. It provides more comprehensive file system operations and better error handling compared to the standard fs module.
FAQs
graceful fs.readlink
The npm package graceful-readlink receives a total of 859,521 weekly downloads. As such, graceful-readlink popularity was classified as popular.
We found that graceful-readlink demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.