Gradienticons
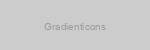
Gradienticons is a powerful and flexible React icon component library that brings your icons to life with gradient support, hover effects, and shape variations. With its intuitive API and flexible input handling, Gradienticons makes it easy to create stunning, customizable icons for any React project.

Features
- 🎨 Support for solid colors and gradients
- 🖱️ Hover effects for colors and gradients
- 🔷 Multiple shape options (circle, square)
- 📏 Customizable size and padding
- 🔳 Background color and shape customization
- 💫 Box shadow and icon shadow effects
- 🔄 Flexible input handling for all props
Table of Contents
Installation
You can install Gradienticons using npm or yarn:
npm install gradienticons
or
yarn add gradienticons
Quick Start
Here's a quick example to get you started with Gradienticons:
import React from 'react';
import { Icon } from 'gradienticons';
function App() {
return (
<div>
<h1>Welcome to My App</h1>
<Icon
name="home"
size={50}
gradientColors={['#ff00ff', '#00ffff']}
shape="circle"
backgroundColor="#f0f0f0"
/>
</div>
);
}
export default App;
Usage
Gradienticons is designed to be flexible and easy to use. Here's a more detailed example showcasing various features:
import React from 'react';
import { Icon } from 'gradienticons';
function MyComponent() {
return (
<div>
<Icon
name="home"
size={50} // or "50px" or "3em"
color="blue"
gradientColors={['#ff00ff', '#00ffff']}
hoverColor="red"
hoverGradientColors={['#ff0000', '#0000ff']}
shape="circle"
backgroundColor="#f0f0f0"
hoverBackgroundColor="#e0e0e0"
outerPadding={10} // or "10px"
opacity={0.8} // or "0.8"
boxShadow="0 4px 6px rgba(0, 0, 0, 0.1)"
iconShadow="2px 2px 2px rgba(0, 0, 0, 0.5)"
cursor="pointer"
/>
</div>
);
}
export default MyComponent;
API Reference
Prop | Type | Default | Description |
---|
name | string | - | Name of the icon (required) |
size | string | number | '1em' | Size of the icon |
color | string | 'currentColor' | Color of the icon |
gradientColors | array | null | Array of two colors for gradient |
hoverColor | string | null | Color on hover |
hoverGradientColors | array | null | Gradient colors on hover |
directionStart | string | 'topLeft' | Start direction of gradient |
directionEnd | string | 'bottomRight' | End direction of gradient |
opacity | string | number | 1 | Opacity of the icon |
backgroundColor | string | 'transparent' | Background color |
hoverBackgroundColor | string | null | Background color on hover |
shape | string | 'circle' | Shape of the icon ('circle' or 'square') |
borderRadius | string | number | 0 | Border radius for square shape |
padding | string | number | 0 | Inner padding |
outerPadding | string | number | 0 | Outer padding |
boxShadow | string | null | Box shadow CSS |
iconShadow | string | null | Icon shadow CSS |
cursor | string | 'default' | Cursor style |
Flexible Input Handling
Gradienticons supports flexible input handling for all props. You can pass values as strings or numbers, and they will be correctly parsed and applied. This flexibility makes it easier to use Gradienticons in various contexts and with different styling approaches.
Example:
<Icon
size={80}
opacity={0.7}
borderRadius="10px"
outerPadding="5"
/>
Contributing
We welcome contributions to Gradienticons! If you'd like to contribute, please follow these steps:
- Fork the repository
- Create your feature branch (
git checkout -b feature/AmazingFeature
) - Commit your changes (
git commit -m 'Add some AmazingFeature'
) - Push to the branch (
git push origin feature/AmazingFeature
) - Open a Pull Request
Please make sure to update tests as appropriate and adhere to the existing coding style.
License
Distributed under the MIT License. See LICENSE
for more information.
MIT © [DHEERAJ]