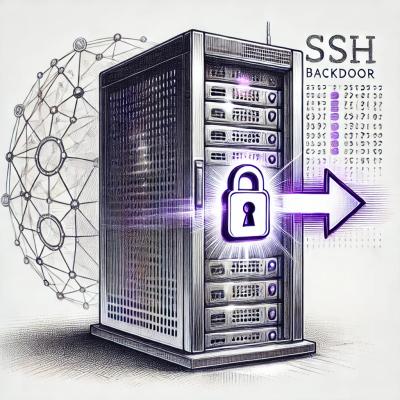
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
graphql-sse
Advanced tools
Zero-dependency, HTTP/1 safe, simple, GraphQL over Server-Sent Events Protocol server and client
The graphql-sse package provides a way to implement GraphQL over Server-Sent Events (SSE). This allows for real-time updates and subscriptions in a GraphQL API using the SSE protocol, which is a simpler alternative to WebSockets.
Setting up a GraphQL server with SSE
This code sets up a basic GraphQL server with SSE support. It defines a schema with a query and a subscription, and starts an HTTP server that listens for GraphQL SSE requests.
const { createServer } = require('http');
const { execute, subscribe } = require('graphql');
const { makeExecutableSchema } = require('@graphql-tools/schema');
const { GraphQLSse } = require('graphql-sse');
const typeDefs = `
type Query {
hello: String
}
type Subscription {
time: String
}
`;
const resolvers = {
Query: {
hello: () => 'Hello world!',
},
Subscription: {
time: {
subscribe: async function* () {
while (true) {
yield { time: new Date().toISOString() };
await new Promise(resolve => setTimeout(resolve, 1000));
}
},
},
},
};
const schema = makeExecutableSchema({ typeDefs, resolvers });
const server = createServer(GraphQLSse({ schema, execute, subscribe }));
server.listen(4000, () => {
console.log('Server is running on http://localhost:4000/graphql');
});
Client-side subscription using EventSource
This code demonstrates how to subscribe to a GraphQL subscription using the EventSource API on the client side. It listens for messages from the server and logs the data to the console.
const eventSource = new EventSource('http://localhost:4000/graphql?query=subscription{time}');
eventSource.onmessage = function(event) {
const data = JSON.parse(event.data);
console.log(data);
};
The subscriptions-transport-ws package provides WebSocket support for GraphQL subscriptions. It is more complex than graphql-sse but offers more features and flexibility for real-time communication.
The graphql-ws package is a lightweight and modern alternative to subscriptions-transport-ws for implementing GraphQL subscriptions over WebSocket. It is designed to be simple and efficient, similar to graphql-sse but using WebSocket instead of SSE.
Apollo Server is a popular GraphQL server implementation that supports subscriptions using WebSocket. It is a comprehensive solution for building GraphQL APIs and includes many features beyond just subscriptions.
Use WebSockets instead? Check out graphql-ws!
Swiftly start with the get started guide on the website.
Short and concise code snippets for starting with common use-cases. Available on the website.
Auto-generated by TypeDoc and then rendered on the website.
Read about the exact transport intricacies used by the library in the GraphQL over Server-Sent Events Protocol document.
File a bug, contribute with code, or improve documentation? Read up on our guidelines for contributing and drive development with yarn test --watch
away!
FAQs
Zero-dependency, HTTP/1 safe, simple, GraphQL over Server-Sent Events Protocol server and client
We found that graphql-sse demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.