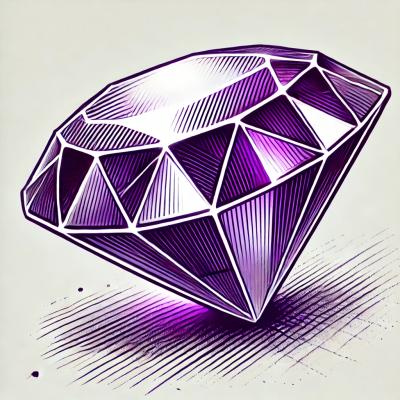
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
graphql-upload
Advanced tools
Middleware and an Upload scalar to add support for GraphQL multipart requests (file uploads via queries and mutations) to various Node.js GraphQL servers.
The graphql-upload npm package provides middleware and utilities for handling file uploads in GraphQL APIs. It allows you to upload files via GraphQL mutations, making it easier to manage file uploads in a GraphQL-based server.
File Upload Middleware
This feature provides middleware for handling file uploads in an Express-based GraphQL server. The code sample demonstrates how to set up the middleware and define a mutation for uploading files.
const { graphqlUploadExpress } = require('graphql-upload');
const express = require('express');
const { ApolloServer, gql } = require('apollo-server-express');
const typeDefs = gql`
type File {
filename: String!
mimetype: String!
encoding: String!
}
type Query {
_ : Boolean
}
type Mutation {
uploadFile(file: Upload!): File!
}
`;
const resolvers = {
Mutation: {
uploadFile: async (parent, { file }) => {
const { createReadStream, filename, mimetype, encoding } = await file;
// Save the file to the filesystem or cloud storage here
return { filename, mimetype, encoding };
}
}
};
const server = new ApolloServer({ typeDefs, resolvers });
const app = express();
app.use(graphqlUploadExpress());
server.applyMiddleware({ app });
app.listen({ port: 4000 }, () => {
console.log(`Server ready at http://localhost:4000${server.graphqlPath}`);
});
Handling File Streams
This feature demonstrates how to handle file streams in a GraphQL mutation. The code sample shows how to extract the file stream from the uploaded file and process it as needed.
const { ApolloServer, gql } = require('apollo-server');
const typeDefs = gql`
type File {
filename: String!
mimetype: String!
encoding: String!
}
type Query {
_ : Boolean
}
type Mutation {
uploadFile(file: Upload!): File!
}
`;
const resolvers = {
Mutation: {
uploadFile: async (parent, { file }) => {
const { createReadStream, filename, mimetype, encoding } = await file;
const stream = createReadStream();
// Process the file stream here (e.g., save to disk, cloud storage, etc.)
return { filename, mimetype, encoding };
}
}
};
const server = new ApolloServer({ typeDefs, resolvers });
server.listen().then(({ url }) => {
console.log(`Server ready at ${url}`);
});
The apollo-upload-server package provides similar functionality for handling file uploads in Apollo Server. It integrates seamlessly with Apollo Server and offers a straightforward way to manage file uploads in GraphQL APIs. Compared to graphql-upload, it is more tightly coupled with Apollo Server and may not be as flexible for use with other GraphQL server implementations.
Middleware and an Upload
scalar to add support for GraphQL multipart requests (file uploads via queries and mutations) to various Node.js GraphQL servers.
First, check if there are GraphQL multipart request spec server implementations (most for Node.js integrate graphql-upload
) that are more suitable for your environment than a manual setup.
To install graphql-upload
and the graphql
peer dependency with npm, run:
npm install graphql-upload graphql
Use the graphqlUploadKoa
or graphqlUploadExpress
middleware just before GraphQL middleware. Alternatively, use processRequest
to create custom middleware.
A schema built with separate SDL and resolvers (e.g. using makeExecutableSchema
from @graphql-tools/schema
) requires the Upload
scalar to be setup.
Clients implementing the GraphQL multipart request spec upload files as Upload
scalar query or mutation variables. Their resolver values are promises that resolve file upload details for processing and storage. Files are typically streamed into cloud storage but may also be stored in the filesystem.
See the example API and client.
os.tmpdir()
.Promise.all
or a more flexible solution such as Promise.allSettled
where an error in one does not reject them all.createReadStream
before the resolver returns; late calls (e.g. in an unawaited async function or callback) throw an error. Existing streams can still be used after a response is sent, although there are few valid reasons for not awaiting their completion.stream.destroy()
when an incomplete stream is no longer needed, or temporary files may not get cleaned up.The GraphQL multipart request spec allows a file to be used for multiple query or mutation variables (file deduplication), and for variables to be used in multiple places. GraphQL resolvers need to be able to manage independent file streams. As resolvers are executed asynchronously, it’s possible they will try to process files in a different order than received in the multipart request.
busboy
parses multipart request streams. Once the operations
and map
fields have been parsed, Upload
scalar values in the GraphQL operations are populated with promises, and the operations are passed down the middleware chain to GraphQL resolvers.
fs-capacitor
is used to buffer file uploads to the filesystem and coordinate simultaneous reading and writing. As soon as a file upload’s contents begins streaming, its data begins buffering to the filesystem and its associated promise resolves. GraphQL resolvers can then create new streams from the buffer by calling the function createReadStream
. The buffer is destroyed once all streams have ended or closed and the server has responded to the request. Any remaining buffer files will be cleaned when the process exits.
^14.17.0 || ^16.0.0 || >= 18.0.0
These ECMAScript modules are published to npm and exported via the package.json
exports
field:
16.0.0
Updated the fs-capacitor
dependency to v8, fixing #318.
The type FileUploadCreateReadStreamOptions
from the processRequest.mjs
module now uses types from fs-capacitor
that are slightly more specific.
The API is now ESM in .mjs
files instead of CJS in .js
files, accessible via import
but not require
. To migrate imports:
- import GraphQLUpload from "graphql-upload/GraphQLUpload.js";
+ import GraphQLUpload from "graphql-upload/GraphQLUpload.mjs";
- import graphqlUploadExpress from "graphql-upload/graphqlUploadExpress.js";
+ import graphqlUploadExpress from "graphql-upload/graphqlUploadExpress.mjs";
- import graphqlUploadKoa from "graphql-upload/graphqlUploadKoa.js";
+ import graphqlUploadKoa from "graphql-upload/graphqlUploadKoa.mjs";
- import processRequest from "graphql-upload/processRequest.js";
+ import processRequest from "graphql-upload/processRequest.mjs";
- import Upload from "graphql-upload/Upload.js";
+ import Upload from "graphql-upload/Upload.mjs";
FAQs
Middleware and a scalar Upload to add support for GraphQL multipart requests (file uploads via queries and mutations) to various Node.js GraphQL servers.
The npm package graphql-upload receives a total of 147,500 weekly downloads. As such, graphql-upload popularity was classified as popular.
We found that graphql-upload demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.