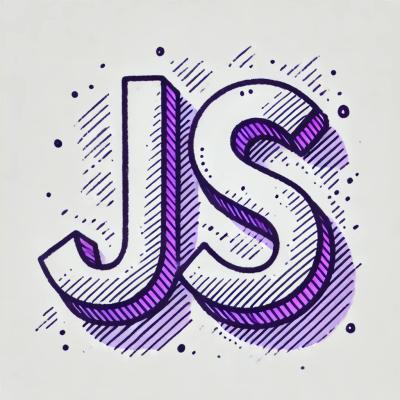
Security News
JavaScript Leaders Demand Oracle Release the JavaScript Trademark
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
The graphql npm package is the JavaScript reference implementation for GraphQL, a query language for APIs and a runtime for executing those queries by using a type system you define for your data. It allows you to create schemas, define resolvers, and execute GraphQL queries and mutations.
Creating a GraphQL Schema
This feature allows you to define the shape of your data and the way it can be queried by clients. The code sample demonstrates how to create a simple GraphQL schema with a single root query.
const { GraphQLSchema, GraphQLObjectType, GraphQLString } = require('graphql');
const schema = new GraphQLSchema({
query: new GraphQLObjectType({
name: 'RootQueryType',
fields: {
hello: {
type: GraphQLString,
resolve() {
return 'Hello world!';
}
}
}
})
});
Executing a GraphQL Query
This feature allows you to execute GraphQL queries against your schema. The code sample shows how to execute a simple query that asks for the 'hello' field, which resolves to 'Hello world!'.
const { graphql, buildSchema } = require('graphql');
const schema = buildSchema(`
type Query {
hello: String
}
`);
const root = { hello: () => 'Hello world!' };
graphql(schema, '{ hello }', root).then((response) => {
console.log(response);
});
Defining Resolvers
Resolvers are functions that resolve the value for a type or field in a schema. The code sample shows how to define a resolver for the 'hello' field that returns a string.
const { GraphQLObjectType, GraphQLString } = require('graphql');
const RootQueryType = new GraphQLObjectType({
name: 'RootQuery',
fields: {
hello: {
type: GraphQLString,
resolve() {
return 'Hello world!';
}
}
}
});
Apollo Server is a community-driven, open-source GraphQL server that works with many Node.js HTTP server frameworks. It simplifies building a GraphQL API by providing features like performance tracing and schema stitching. Compared to graphql, Apollo Server offers a more integrated set of tools for building production-ready GraphQL services.
Express GraphQL is a GraphQL HTTP server middleware for Express.js. It allows you to serve a GraphQL API as a web service. While graphql provides the core functionality for schema creation and query execution, express-graphql makes it easy to integrate GraphQL with the Express web application framework.
TypeGraphQL is a framework for building GraphQL APIs in Node.js using TypeScript, based on the official graphql-js package. It allows for defining the schema using TypeScript classes and decorators, which can be more familiar to developers with an object-oriented background. It provides a more type-safe and class-based approach to defining GraphQL schemas compared to the more traditional, function-based approach of graphql.
FAQs
A Query Language and Runtime which can target any service.
The npm package graphql receives a total of 13,045,979 weekly downloads. As such, graphql popularity was classified as popular.
We found that graphql demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.