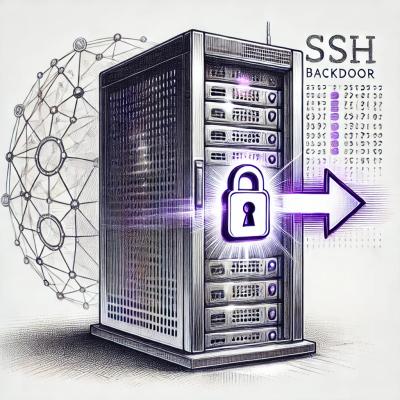
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
grunt-prompt
Advanced tools
Add interactive UI to your Gruntfile such as lists, checkboxes, text input with filtering, and password fields, all on the command line.
Ask questions during your Grunt workflow. Use user input for later tasks
This plugin requires Grunt ~0.4.1
npm install grunt-prompt --save-dev
One the plugin has been installed, it may be enabled inside your Gruntfile with this line of JavaScript:
grunt.loadNpmTasks('grunt-prompt');
In your project's Gruntfile, add a section named prompt
to the data object passed into grunt.initConfig()
.
grunt-prompt
is a multi-task. This means you can create multiple prompts.
grunt.initConfig({
prompt: {
target: {
options: {
questions: [
config: 'config.name', // arbitray name or config for any other grunt task
type: '<question type>', // list, checkbox, confirm, input, password
message: 'Question to ask the user',
default: 'value', // default value if nothing is entered
choices: 'Array|Function(answers)',
validate: Function(value), // return true if valid, error message if invalid
filter: Function(value), // modify the answer
when: Function(answers) // only ask this question when this function returns true
]
}
},
},
})
Type: String
required
This is used for three things:
config: 'jshint.allFiles.reporter'
answers
object: if (answers['jshint.allFiles.reporter'] === 'custom') {...
grunt.config
: grunt.config('jshint.allFiles.reporter')
Type: String
required
Type of question to ask:
list
: use arrow keys to pick one choice. Returns a string.checkbox
: use arrow keys and space bar to pick multiple items. Returns an array.confirm
: Yes/no. Returns a boolean.input
: Free text input. Returns a string.password
: Masked input. Returns a string.The documentation for Inquiry has more details about type as well as additional typess.
Type: String
required
Question to ask the user.
Hint: keep it short, users hate to read.
Type: String
/Array
/Boolean
depending on question type
optional
Default value used when the user just bangs Enter.
For question type 'list'
: Type: array of strings
choices: ['jshint', 'jslint', 'eslint', 'I like to live dangerously.']
For question type 'checkbox'
: Type: array of hashes
Include checked: true
to select it by default.
choices: [
{name: 'jshint', checked: true},
{name: 'jslint'},
{name: 'eslint'}
]
Type: function(value)
optional
Return true
if it is valid (true true
, not a truthy value).
Return string
message if it is not valid.
Type: function(value)
optional
Use a modified version of the input for the answer. Useful for stripping extra characters, converting strings to integers, etc.
Type: function(answers)
optional
Choose when this question is asked. Perfect for asking questions based on the results of previous questions.
This is an example of how grunt-prompt
for something like grunt-bump which makes it easy to
update your project's version in the package.json
, bower.json
, and git tag
.
prompt: {
prompt: {
bump: {
options: {
questions: [
{
config: 'bump.increment',
type: 'list',
// normally these versions wouldn't be hardcoded
message: 'Bump version from ' + '1.2.3'.cyan + ' to:',
choices: [
'1.2.4-? ❘❙❚ Build: unstable, betas, and release candidates.',
'1.2.4 ❘❙❚ Patch: backwards-compatible bug fixes.',
'1.3.0 ❘❙❚ Minor: add functionality in a backwards-compatible manner.',
'2.0.0 ❘❙❚ Major: incompatible API changes.',
'?.?.? ❘❙❚ Custom: Specify version...'
],
filter: function (value) {
var matches = value.match(/([^(\s)]*):/);
return matches && matches[1].toLowerCase();
}
},
{
config: 'bump.version',
type: 'input',
message: 'What specific version would you like',
when: function (answers) {
return answers['bump.increment'] === 'custom';
},
validate: function (value) {
var valid = require('semver').valid(value) && true;
return valid || 'Must be a valid semver, such as 1.2.3. See http://semver.org/';
}
},
{
config: 'bump.files',
type: 'checkbox',
message: 'What should get the new version:',
choices: [
{
name: 'package.json',
checked: grunt.file.isFile('package.json')
},
{
name: 'bower.json',
checked: grunt.file.isFile('bower.json')
},
{
name: 'git tag',
checked: grunt.file.isDir('.git')
}
]
}
]
}
}
}
}```
FAQs
Interactive prompt for your Grunt config using console checkboxes, text input with filtering, password fields.
We found that grunt-prompt demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.