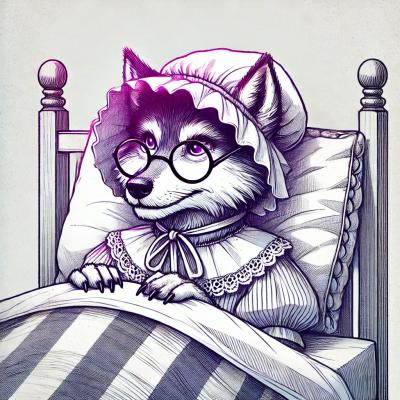
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Gulp is a toolkit that helps developers automate and enhance workflows. It is a streaming build system that allows the use of Node streams to read files from the filesystem, transform them, and output them back to the filesystem or elsewhere. Gulp is commonly used for tasks such as minification, concatenation, cache busting, unit testing, linting, and optimization.
Task Automation
Automate repetitive tasks with custom defined tasks.
const gulp = require('gulp');
gulp.task('default', function() {
// Your task code here
});
File Minification
Minify JavaScript files to reduce their size for production.
const gulp = require('gulp');
const uglify = require('gulp-uglify');
gulp.task('minify-js', function() {
return gulp.src('src/*.js')
.pipe(uglify())
.pipe(gulp.dest('dist'));
});
File Concatenation
Concatenate multiple files into a single file.
const gulp = require('gulp');
const concat = require('gulp-concat');
gulp.task('concat-js', function() {
return gulp.src('src/*.js')
.pipe(concat('all.js'))
.pipe(gulp.dest('dist'));
});
Sass Compilation
Compile Sass files into CSS.
const gulp = require('gulp');
const sass = require('gulp-sass');
gulp.task('sass', function() {
return gulp.src('src/*.scss')
.pipe(sass().on('error', sass.logError))
.pipe(gulp.dest('dist/css'));
});
Live Reloading
Automatically reload the browser when files are modified.
const gulp = require('gulp');
const browserSync = require('browser-sync').create();
gulp.task('serve', function() {
browserSync.init({
server: './dist'
});
gulp.watch('src/*.html').on('change', browserSync.reload);
});
Webpack is a powerful module bundler that can also run many of the same tasks as Gulp, but it focuses more on bundling JavaScript modules together. It has a different plugin system and uses a configuration file for defining the build steps.
Grunt is another task runner like Gulp, but it uses a configuration-over-code approach. It has a large plugin ecosystem and is configured in a declarative manner, which can be more verbose than Gulp's code-based task definitions.
Rollup is a module bundler for JavaScript which uses the new standardized format for code modules included in the ES6 revision of JavaScript. It is similar to Webpack but is known for producing smaller bundles.
Parcel is a web application bundler that offers a zero-configuration setup. It aims to provide out-of-the-box support for many development tasks without the need for additional plugins or configurations, unlike Gulp which requires setting up tasks and plugins.
Broccoli is a fast, reliable asset pipeline, supporting constant-time rebuilds and compact build definitions. Similar to Gulp, it uses a plugin architecture but focuses on providing the fastest rebuilds and simplicity in build configurations.
The streaming build system
Follow our Quick Start guide.
Find out about all our work-in-progress and outstanding issues at https://github.com/orgs/gulpjs/projects.
Check out the Getting Started guide and API docs on our website!
Excuse our dust! All other docs will be behind until we get everything updated. Please open an issue if something isn't working.
gulpfile.js
This file will give you a taste of what gulp does.
var gulp = require('gulp');
var less = require('gulp-less');
var babel = require('gulp-babel');
var concat = require('gulp-concat');
var uglify = require('gulp-uglify');
var rename = require('gulp-rename');
var cleanCSS = require('gulp-clean-css');
var del = require('del');
var paths = {
styles: {
src: 'src/styles/**/*.less',
dest: 'assets/styles/'
},
scripts: {
src: 'src/scripts/**/*.js',
dest: 'assets/scripts/'
}
};
/* Not all tasks need to use streams, a gulpfile is just another node program
* and you can use all packages available on npm, but it must return either a
* Promise, a Stream or take a callback and call it
*/
function clean() {
// You can use multiple globbing patterns as you would with `gulp.src`,
// for example if you are using del 2.0 or above, return its promise
return del([ 'assets' ]);
}
/*
* Define our tasks using plain functions
*/
function styles() {
return gulp.src(paths.styles.src)
.pipe(less())
.pipe(cleanCSS())
// pass in options to the stream
.pipe(rename({
basename: 'main',
suffix: '.min'
}))
.pipe(gulp.dest(paths.styles.dest));
}
function scripts() {
return gulp.src(paths.scripts.src, { sourcemaps: true })
.pipe(babel())
.pipe(uglify())
.pipe(concat('main.min.js'))
.pipe(gulp.dest(paths.scripts.dest));
}
function watch() {
gulp.watch(paths.scripts.src, scripts);
gulp.watch(paths.styles.src, styles);
}
/*
* Specify if tasks run in series or parallel using `gulp.series` and `gulp.parallel`
*/
var build = gulp.series(clean, gulp.parallel(styles, scripts));
/*
* You can use CommonJS `exports` module notation to declare tasks
*/
exports.clean = clean;
exports.styles = styles;
exports.scripts = scripts;
exports.watch = watch;
exports.build = build;
/*
* Define default task that can be called by just running `gulp` from cli
*/
exports.default = build;
Gulp provides a wrapper that will be loaded in your ESM code, so you can name your gulpfile as gulpfile.mjs
or with "type": "module"
specified in your package.json
file.
And here's the same sample from above written in ESNext.
import { src, dest, watch } from 'gulp';
import less from 'gulp-less';
import babel from 'gulp-babel';
import concat from 'gulp-concat';
import uglify from 'gulp-uglify';
import rename from 'gulp-rename';
import cleanCSS from 'gulp-clean-css';
import del from 'del';
const paths = {
styles: {
src: 'src/styles/**/*.less',
dest: 'assets/styles/'
},
scripts: {
src: 'src/scripts/**/*.js',
dest: 'assets/scripts/'
}
};
/*
* For small tasks you can export arrow functions
*/
export const clean = () => del([ 'assets' ]);
/*
* You can also declare named functions and export them as tasks
*/
export function styles() {
return src(paths.styles.src)
.pipe(less())
.pipe(cleanCSS())
// pass in options to the stream
.pipe(rename({
basename: 'main',
suffix: '.min'
}))
.pipe(dest(paths.styles.dest));
}
export function scripts() {
return src(paths.scripts.src, { sourcemaps: true })
.pipe(babel())
.pipe(uglify())
.pipe(concat('main.min.js'))
.pipe(dest(paths.scripts.dest));
}
/*
* You could even use `export as` to rename exported tasks
*/
function watchFiles() {
watch(paths.scripts.src, scripts);
watch(paths.styles.src, styles);
}
export { watchFiles as watch };
const build = gulp.series(clean, gulp.parallel(styles, scripts));
/*
* Export a default task
*/
export default build;
You can filter out unchanged files between runs of a task using
the gulp.src
function's since
option and gulp.lastRun
:
const paths = {
...
images: {
src: 'src/images/**/*.{jpg,jpeg,png}',
dest: 'build/img/'
}
}
function images() {
return gulp.src(paths.images.src, {since: gulp.lastRun(images)})
.pipe(imagemin())
.pipe(gulp.dest(paths.images.dest));
}
function watch() {
gulp.watch(paths.images.src, images);
}
Task run times are saved in memory and are lost when gulp exits. It will only
save time during the watch
task when running the images
task
for a second time.
Anyone can help make this project better - check out our Contributing guide!
5.0.0 (2024-03-29)
We've tried to provide a high-level changelog for gulp v5 below, but it doesn't contain all changes from the 60+ dependencies that we maintain.
Please see individual changelogs to drill down into all changes that were made.
anymatch
library for globbing paths. All globs should work the same between src
and watch
now!ordered-read-stream
.gulp.*
config filesgulp-cli
--verify
flag--require
flag to --preload
to avoid conflicting with Node.js flagsVinyl
objects with stream contents using teex
, but no longer wait for all streams to flow before cloned streams will receive dataprocess.umask()
to make directories, instead falling back to Node's default modegulplog
namespace to the storegulplog
store to the globalv8flags
cache into a filenameglob-stream
to use a custom directory walk that relies on newer Node.js features and is more performant than old implementationgulpile.cjs
and gulpfile.mjs
swc
, esbuild
, sucrase
, and mdx
loadersVinyl
contentsextends
syntax for .gulp.*
config filegulpfile
and preloads
via .gulp.*
config fileto-absolute-glob
to:
../
at the beginning of globsstream
, readable-stream
, and streamx
We created and maintain various projects that gulp depends upon. You can find their changelogs linked below:
FAQs
The streaming build system.
The npm package gulp receives a total of 0 weekly downloads. As such, gulp popularity was classified as not popular.
We found that gulp demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.