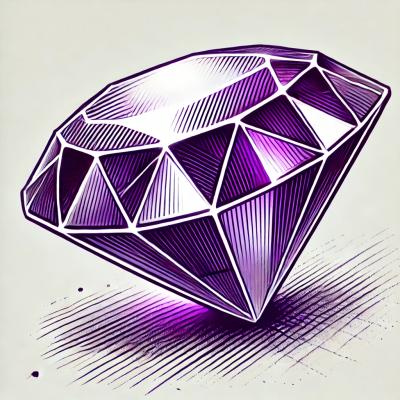
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Fork of HAMT that adds a transaction interface and support for custom key types
The hamt_plus npm package provides a high-performance, immutable hash array mapped trie (HAMT) implementation. It is designed for efficient storage and retrieval of key-value pairs, supporting operations like insertion, deletion, and lookup in a way that ensures immutability.
Creating a new HAMT
This feature allows you to create a new, empty HAMT. The `make` function initializes an empty hash array mapped trie.
const hamt = require('hamt_plus');
const map = hamt.make();
Inserting a key-value pair
This feature allows you to insert a key-value pair into the HAMT. The `set` method returns a new HAMT with the key-value pair added, ensuring immutability.
const hamt = require('hamt_plus');
let map = hamt.make();
map = map.set('key', 'value');
Retrieving a value by key
This feature allows you to retrieve a value by its key from the HAMT. The `get` method returns the value associated with the specified key.
const hamt = require('hamt_plus');
let map = hamt.make();
map = map.set('key', 'value');
const value = map.get('key');
Deleting a key-value pair
This feature allows you to delete a key-value pair from the HAMT. The `delete` method returns a new HAMT with the specified key-value pair removed.
const hamt = require('hamt_plus');
let map = hamt.make();
map = map.set('key', 'value');
map = map.delete('key');
Checking for a key
This feature allows you to check if a key exists in the HAMT. The `has` method returns a boolean indicating whether the key is present.
const hamt = require('hamt_plus');
let map = hamt.make();
map = map.set('key', 'value');
const hasKey = map.has('key');
The `immutable` package provides a variety of immutable data structures, including maps, sets, and lists. It offers a more comprehensive suite of immutable collections compared to `hamt_plus`, which focuses specifically on HAMTs.
The `mori` package is a library for using ClojureScript's persistent data structures and supporting API in JavaScript. It provides a broader range of persistent data structures, including vectors, maps, and sets, similar to `hamt_plus` but with a different API and additional data structures.
The `immutable-js` package by Facebook provides immutable data structures for JavaScript, including maps, sets, lists, and more. It offers a more extensive collection of immutable data structures compared to `hamt_plus`, which is specialized in HAMTs.
Fork of HAMT with transactions and custom key types.
This library is a fork of the HAMT hash array mapped trie library that adds a few important features in exchange for slightly degraded performance:
A transaction interface for mutating a map in a specific context. This allows efficent mass operations, while retaining the safety of a persistent data structure.
Custom key compare function.
Custom hash function.
The APIs of HAMT and HAMT+ are nearly identical, the major difference being
how empty maps are created and the lack of getHash
type operations in HAMT+,
which instead can use a user defined hash operation.
Node source is in dist_node/hamt.js
$ npm install hamt_plus
var hamt = require('hamt_plus');
var h = hamt.make();
h = hamt.set('key', 'value', h);
...
Amd source is in dist/hamt.js
requirejs.config({
paths: {
'hamt': 'dist/hamt'
}
});
require([
'hamt'],
function(hamt) {
...
});
var hamt = require('hamt_plus');
// Empty map
var h = hamt.make();
// Set 'key' to 'value'
h = hamt.set('key', 'value', h);
// Get 'key'
hamt.get('key', h); // 'value'
// The data structure is persistent so the original is not modified.
var h1 = hamt.set('a', 'x', hamt.make());
var h2 = hamt.set('b', 'y', h1);
hamt.get('a', h1); // 'x'
hamt.get('b', h1); // null
hamt.get('a', h2); // 'x'
hamt.get('b', h2); // 'y'
// Modify an entry
h2 = hamt.modify('b', function(x) { return x + 'z'; }, h2);
hamt.get('b', h2); // 'yz'
// Remove an entry
h2 = hamt.remove('b', h2);
hamt.get('a', h2); // 'x'
hamt.get('b', h2); // null
/* Aggregation ---------------------------------------------------------------*/
var h = hamt.set('b', 'y', hamt.set('a', 'x', hamt.make()));
hamt.count(h); // 2
hamt.keys(h); // ['b', 'a'];
hamt.values(h); // ['y', 'x'];
hamt.pairs(h); // [['b', 'y'], ['a', 'x']];
// Fold
var h = hamt.set('a', 10, hamt.set('b', 4, hamt.set('c', -2, hamt.make())));
hamt.fold(\p {value} -> p + value, h); // 12
/* Customization -------------------------------------------------------------*/
// Use a custom hash function or key compare function
var h = hamt.make({
hash: function(key) {
return hamt.hash(key.x + " " + key.y);
},
keyEq: function(key1, key2) {
return key1.x === key2.x && key1.y === key2.y;
}});
h = hamt.set(new Vec2(1, 2), 'abc', h);
h = hamt.set(new Vec2(3, 4), 'efg', h);
hamt.get(new Vec2(3, 4), h); // 'efg'
hamt.get(new Vec2(1, 2), h); // 'abc'
/* Mutation ------------------------------------------------------------------*/
var keys = [...];
var h = hamt.mutate(function(h) {
// Operations inside this block may mutate `h` to improve performance
// but mutation may not leak out of this block.
keys.forEach(function(key, i) {
hamt.set(key, i, h);
});
}, hamt.make());
0.0.4 - Aug 21, 2014
CollisionNode
not having an edit
member.FAQs
Fork of HAMT that adds transient mutation and support for custom key types
The npm package hamt_plus receives a total of 194,652 weekly downloads. As such, hamt_plus popularity was classified as popular.
We found that hamt_plus demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.