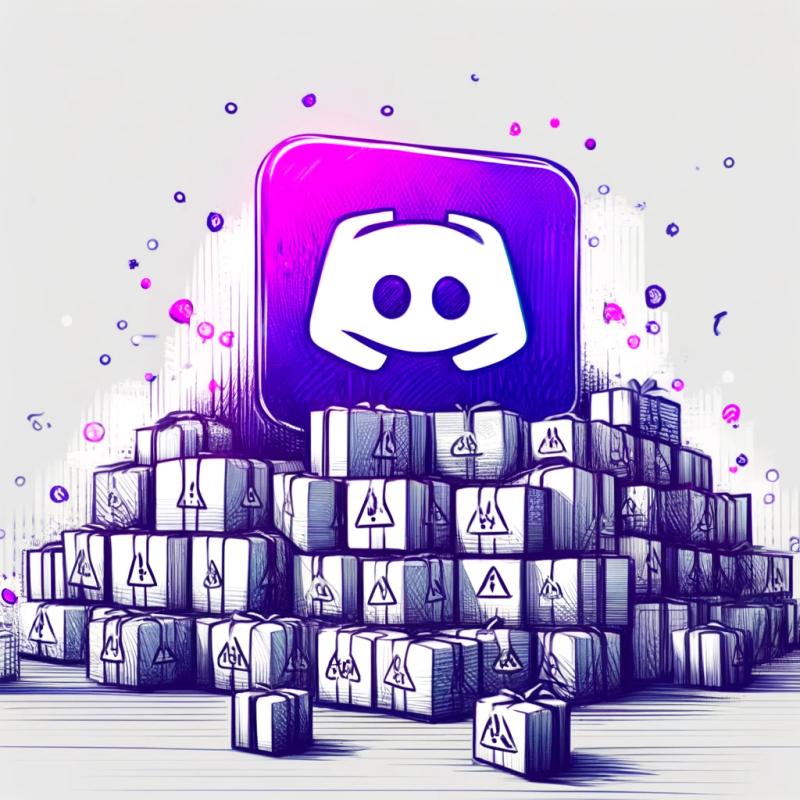
Research
Recent Trends in Malicious Packages Targeting Discord
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
hashset
Advanced tools
Readme
A simple hash set in JavaScript
var HashSet = require('hashset');
//Create an empty hash set
var hashset = new HashSet();
//Create a hash set an initialize it with a value 'a'
var hashset = new HashSet('a');
//Create a hash set an initialize it with a set of values
var hashset = new HashSet('a', 'b', 'c');
//Create a hash set an initialize it with 3 different values
var hashset = new HashSet(1, '1', [1]);
Get the length of the hash set
hashset.length;
Test whether current hash set equals to another
var hashset1 = new HashSet(1, 2, 3, 4, 5);
var hashset2 = new HashSet(1, 2, 3, 4, 5);
var result = hashset1.equals(hashset2); //true
Check whether a value is in the hash set
hashset.contains(val);
Add a new value into the hash set
hashset.add(val);
Remove a value from the hash set
hashset.remove(val);
Removing an non-existing value will not trigger any error
Convert and output all values into an array
var hashset = new HashSet(1, 2, 3);
hashset.toArray(); // [1, 2, 3]
There is no gurantee the values of the output array the same sequence as value added to the hash set.
Test whether a hash set is a sub set of another. Empty hash set is a sub set of any other hash set including another empty hash set.
var hashset1 = new HashSet();
var hashset2 = new HashSet();
var result = hashset1.isSubSetOf(hashset2);
Test whether a hash set is a super set of another. Any hash set is a super set of empty hahs set including an empty hash set.
var hashset1 = new HashSet(1, 2, 3);
var hashset2 = new HashSet(1, 2, 3);
var hashset3 = new HashSet();
var result1 = hashset1.isSuperSetOf(hashset2); //true
var result2 = hashset2.isSuperSetOf(hashset1); //true
var result3 = hashset1.isSuperSetOf(hashset3); //true
var result3 = hashset3.isSuperSetOf(hashset1); //false
Union current hash set with another
var hashset1 = new HashSet(1, 2);
var hashset2 = new HashSet(2, 3);
hashset1.unionWith(hashset2);
hashset1.contains(1); //true
hashset1.contains(2); //true
hashset1.contains(3); //true
Intersect current hash set with another
var hashset1 = new HashSet(1, 2, 3);
var hashset2 = new HashSet(2, 3, 4);
hashset1.intersectWith(hashset2);
hashset1.length; //2
hashset1.contains(2); //true
hashset1.contains(3); //true
hashset1.contains(4); //false
Make sure mocha
is installed globally
npm install mocha -g
Run npm test
to run unit test
MIT
FAQs
A simple hash set in JavaScript
The npm package hashset receives a total of 11 weekly downloads. As such, hashset popularity was classified as not popular.
We found that hashset demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
Security News
Socket CEO Feross Aboukhadijeh joins a16z partners to discuss how modern, sophisticated supply chain attacks require AI-driven defenses and explore the challenges and solutions in leveraging AI for threat detection early in the development life cycle.
Security News
NIST's new AI Risk Management Framework aims to enhance the security and reliability of generative AI systems and address the unique challenges of malicious AI exploits.