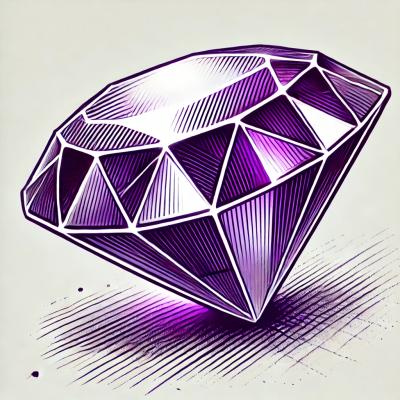
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
hast-util-sanitize
Advanced tools
The `hast-util-sanitize` package is a utility for sanitizing HTML content represented as HAST (Hypertext Abstract Syntax Tree). It helps in cleaning and securing HTML by removing potentially harmful elements and attributes, making it safe for use in web applications.
Basic Sanitization
This feature allows you to sanitize a basic HAST tree, removing any potentially harmful elements or attributes.
const sanitize = require('hast-util-sanitize');
const hast = { type: 'element', tagName: 'div', properties: { className: 'foo' }, children: [] };
const cleanHast = sanitize(hast);
console.log(cleanHast);
Custom Schema
This feature allows you to define a custom schema for sanitization, specifying which tags and attributes are allowed.
const sanitize = require('hast-util-sanitize');
const schema = { tagNames: ['div', 'span'], attributes: { '*': ['className'] } };
const hast = { type: 'element', tagName: 'div', properties: { className: 'foo', id: 'bar' }, children: [] };
const cleanHast = sanitize(hast, schema);
console.log(cleanHast);
Sanitizing Nested Elements
This feature demonstrates sanitizing nested elements, ensuring that even deeply nested potentially harmful elements are removed.
const sanitize = require('hast-util-sanitize');
const hast = { type: 'element', tagName: 'div', properties: {}, children: [ { type: 'element', tagName: 'script', properties: {}, children: [] } ] };
const cleanHast = sanitize(hast);
console.log(cleanHast);
The `sanitize-html` package is a powerful library for cleaning up user-submitted HTML, removing any potentially harmful elements and attributes. It offers a high level of customization and is widely used for sanitizing HTML content. Compared to `hast-util-sanitize`, `sanitize-html` works directly with HTML strings rather than HAST.
The `dompurify` package is a fast and tolerant XSS sanitizer for HTML, MathML, and SVG. It works by parsing the input and then serializing it back to a string, ensuring that only safe content is retained. Unlike `hast-util-sanitize`, `dompurify` operates on DOM nodes and HTML strings.
The `xss` package is a robust library for filtering and sanitizing HTML to prevent XSS attacks. It provides a rich set of options for customizing the sanitization process. While `hast-util-sanitize` focuses on HAST, `xss` works directly with HTML strings.
hast utility to sanitize a tree.
npm:
npm install hast-util-sanitize
var h = require('hastscript')
var u = require('unist-builder')
var sanitize = require('hast-util-sanitize')
var toHtml = require('hast-util-to-html')
var tree = h('div', {onmouseover: 'alert("alpha")'}, [
h(
'a',
{href: 'jAva script:alert("bravo")', onclick: 'alert("charlie")'},
'delta'
),
u('text', '\n'),
h('script', 'alert("charlie")'),
u('text', '\n'),
h('img', {src: 'x', onerror: 'alert("delta")'}),
u('text', '\n'),
h('iframe', {src: 'javascript:alert("echo")'}),
u('text', '\n'),
h('math', h('mi', {'xlink:href': 'data:x,<script>alert("foxtrot")</script>'}))
])
var unsanitized = toHtml(tree)
var sanitized = toHtml(sanitize(tree))
console.log(unsanitized)
console.log(sanitized)
Unsanitized:
<div onmouseover="alert("alpha")"><a href="jAva script:alert("bravo")" onclick="alert("charlie")">delta</a>
<script>alert("charlie")</script>
<img src="x" onerror="alert("delta")">
<iframe src="javascript:alert("echo")"></iframe>
<math><mi xlink:href="data:x,<script>alert("foxtrot")</script>"></mi></math></div>
Sanitized:
<div><a>delta</a>
<img src="x">
</div>
sanitize(tree[, schema])
Schema
Configuration. If not given, defaults to GitHub style sanitation. If any top-level key isn’t given, it defaults to GitHub’s style too.
For a thorough sample, see github.json
.
To extend the standard schema with a few changes, clone github.json
like so:
var h = require('hastscript')
var merge = require('deepmerge')
var gh = require('hast-util-sanitize/lib/github')
var sanitize = require('hast-util-sanitize')
var schema = merge(gh, {attributes: {'*': ['className']}})
var tree = sanitize(h('div', {className: ['foo']}), schema)
// `tree` still has `className`.
console.log(tree)
attributes
Map of tag names to allowed property names
(Object.<Array.<string>>
).
The special '*'
key defines property names allowed on all
elements.
One special value, namely 'data*'
, can be used to allow all data
properties.
"attributes": {
"a": [
"href"
],
"img": [
"src",
"longDesc"
],
// …
"*": [
"abbr",
"accept",
"acceptCharset",
// …
"vSpace",
"width",
"itemProp"
]
}
Instead of a single string (such as type
), which allows any property
value of that property name, it’s also possible to provide
an array (such as ['type', 'checkbox']
), where the first entry is the
property name, and all other entries allowed property values.
This is how the default GitHub schema allows only disabled checkbox inputs:
"attributes": {
// …
"input": [
["type", "checkbox"],
["disabled", true]
],
// …
}
This also plays well with properties that accept space- or comma-separated
values, such as class
.
Say you wanted to allow certain classes on span
elements for syntax
highlighting, that can be done like this:
// …
"span": [
["className", "token", "number", "operator"]
],
// …
required
Map of tag names to required property names and their default
property value (Object.<Object.<*>>
).
If the defined keys do not exist in an element’s
properties, they are added and set to the specified value.
Note that properties are first checked based on the schema at attributes
,
so properties could be removed by that step and then added again through
required
.
"required": {
"input": {
"type": "checkbox",
"disabled": true
}
}
tagNames
List of allowed tag names (Array.<string>
).
"tagNames": [
"h1",
"h2",
"h3",
// …
"strike",
"summary",
"details"
]
protocols
Map of protocols to allow in property values
(Object.<Array.<string>>
).
"protocols": {
"href": [
"http",
"https",
"mailto"
],
// …
"longDesc": [
"http",
"https"
]
}
ancestors
Map of tag names to their required ancestor elements
(Object.<Array.<string>>
).
"ancestors": {
"li": [
"ol",
"ul"
],
// …
"tr": [
"table"
]
}
clobber
List of allowed property names which can clobber (Array.<string>
).
"clobber": [
"name",
"id"
]
clobberPrefix
Prefix to use before potentially clobbering property names (string
).
"clobberPrefix": "user-content-"
strip
Names of elements to strip from the tree
(Array.<string>
).
By default, unsafe elements are replaced by their children. Some elements, should however be entirely stripped from the tree.
"strip": [
"script"
]
allowComments
Whether to allow comments (boolean
, default: false
).
"allowComments": true
allowDoctypes
Whether to allow doctypes (boolean
, default: false
).
"allowDoctypes": true
Improper use of hast-util-sanitize
can open you up to a
cross-site scripting (XSS) attack.
The defaults are safe, but deviating from them is likely unsafe.
Use hast-util-sanitize
after all other utilities, as other utilities are
likely also unsafe.
rehype-sanitize
— rehype plugin wrapperSee contributing.md
in syntax-tree/.github
for ways to get
started.
See support.md
for ways to get help.
This project has a code of conduct. By interacting with this repository, organization, or community you agree to abide by its terms.
FAQs
hast utility to sanitize nodes
The npm package hast-util-sanitize receives a total of 438,340 weekly downloads. As such, hast-util-sanitize popularity was classified as popular.
We found that hast-util-sanitize demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.