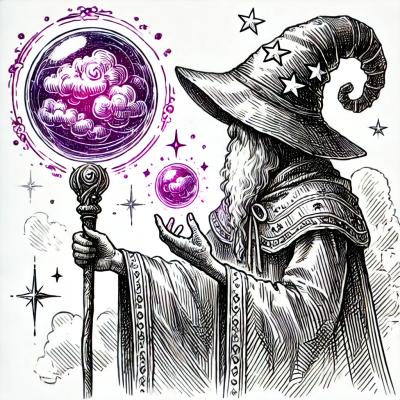
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
hastscript
Advanced tools
The hastscript npm package, often abbreviated as 'h', is a utility that allows for the easy creation of HAST (Hypertext Abstract Syntax Tree) nodes. HAST is a virtual DOM representation used for parsing, manipulating, and serializing HTML and XML documents. This package is particularly useful for developers working with virtual DOMs or those who need to manipulate HTML/XML documents programmatically.
Creating elements
This feature allows for the creation of HAST elements. The example demonstrates creating a 'div' element with a class of 'container' and the text 'Hello, world!' as its child.
const h = require('hastscript');
const element = h('div', {className: 'container'}, 'Hello, world!');
Creating elements with children
This feature enables the creation of HAST elements that contain other elements as children. The code sample shows how to create an unordered list ('ul') with two list items ('li') as its children.
const h = require('hastscript');
const list = h('ul', [
h('li', 'Item 1'),
h('li', 'Item 2')
]);
Creating elements with properties
This feature allows for the creation of HAST elements with specific properties. In the example, an anchor ('a') element is created with an 'href' attribute pointing to 'https://example.com' and containing the text 'Visit Example'.
const h = require('hastscript');
const link = h('a', {href: 'https://example.com'}, 'Visit Example');
Similar to hastscript, react-hyperscript provides a way to create React elements using a hyperscript-like syntax. While hastscript is focused on creating HAST nodes for HTML/XML documents, react-hyperscript is tailored for React's virtual DOM.
The virtual-dom package offers a virtual DOM implementation for efficient re-rendering of web interfaces. Unlike hastscript, which is specifically designed for creating HAST nodes, virtual-dom focuses on general virtual DOM functionality, including diffing and patching algorithms.
Snabbdom is a virtual DOM library that is lightweight and extensible. It shares similarities with hastscript in terms of manipulating virtual DOM trees but is more focused on being a core part of user interface rendering and updates, rather than specifically dealing with HAST nodes.
hast utility to create trees in HTML or SVG.
Similar to hyperscript
, virtual-dom/h
,
React.createElement
, and Vue’s createElement
,
but for hast.
Use unist-builder
to create any unist tree.
npm:
npm install hastscript
var h = require('hastscript')
var s = require('hastscript/svg')
// Children as an array:
console.log(
h('.foo#some-id', [
h('span', 'some text'),
h('input', {type: 'text', value: 'foo'}),
h('a.alpha', {class: 'bravo charlie', download: 'download'}, [
'delta',
'echo'
])
])
)
// Children as arguments:
console.log(
h(
'form',
{method: 'POST'},
h('input', {type: 'text', name: 'foo'}),
h('input', {type: 'text', name: 'bar'}),
h('input', {type: 'submit', value: 'send'})
)
)
// SVG:
console.log(
s('svg', {xmlns: 'http://www.w3.org/2000/svg', viewbox: '0 0 500 500'}, [
s('title', 'SVG `<circle>` element'),
s('circle', {cx: 120, cy: 120, r: 100})
])
)
Yields:
{
type: 'element',
tagName: 'div',
properties: {className: ['foo'], id: 'some-id'},
children: [
{
type: 'element',
tagName: 'span',
properties: {},
children: [{type: 'text', value: 'some text'}]
},
{
type: 'element',
tagName: 'input',
properties: {type: 'text', value: 'foo'},
children: []
},
{
type: 'element',
tagName: 'a',
properties: {className: ['alpha', 'bravo', 'charlie'], download: true},
children: [{type: 'text', value: 'delta'}, {type: 'text', value: 'echo'}]
}
]
}
{
type: 'element',
tagName: 'form',
properties: {method: 'POST'},
children: [
{
type: 'element',
tagName: 'input',
properties: {type: 'text', name: 'foo'},
children: []
},
{
type: 'element',
tagName: 'input',
properties: {type: 'text', name: 'bar'},
children: []
},
{
type: 'element',
tagName: 'input',
properties: {type: 'submit', value: 'send'},
children: []
}
]
}
{
type: 'element',
tagName: 'svg',
properties: {xmlns: 'http://www.w3.org/2000/svg', viewBox: '0 0 500 500'},
children: [
{
type: 'element',
tagName: 'title',
properties: {},
children: [{type: 'text', value: 'SVG `<circle>` element'}]
},
{
type: 'element',
tagName: 'circle',
properties: {cx: 120, cy: 120, r: 100},
children: []
}
]
}
h(selector?[, properties][, ...children])
s(selector?[, properties][, ...children])
DSL to create virtual hast trees for HTML or SVG.
selector
Simple CSS selector (string
, optional).
Can contain a tag name (foo
), IDs (#bar
), and classes (.baz
).
If there is no tag name in the selector, h
defaults to a div
element,
and s
to a g
element.
selector
is parsed by hast-util-parse-selector
.
properties
Map of properties (Object.<*>
, optional).
children
(Lists of) child nodes (string
, Node
, Array.<string|Node>
, optional).
When strings are encountered, they are mapped to text
nodes.
Use of hastscript
can open you up to a cross-site scripting (XSS)
attack as values are injected into the syntax tree.
The following example shows how a script is injected that runs when loaded in a
browser.
var tree = {type: 'root', children: []}
tree.children.push(h('script', 'alert(1)'))
Yields:
<script>alert(1)</script>
The following example shows how an image is injected that fails loading and therefore runs code in a browser.
var tree = {type: 'root', children: []}
// Somehow someone injected these properties instead of an expected `src` and
// `alt`:
var otherProps = {src: 'x', onError: 'alert(2)'}
tree.children.push(h('img', {src: 'default.png', ...otherProps}))
Yields:
<img src="x" onerror="alert(2)">
The following example shows how code can run in a browser because someone stored an object in a database instead of the expected string.
var tree = {type: 'root', children: []}
// Somehow this isn’t the expected `'wooorm'`.
var username = {
type: 'element',
tagName: 'script',
children: [{type: 'text', value: 'alert(3)'}]
}
tree.children.push(h('span.handle', username))
Yields:
<span class="handle"><script>alert(3)</script></span>
Either do not use user input in hastscript
or use
hast-util-santize
.
unist-builder
— Create any unist treehast-to-hyperscript
— Convert a Node to React, Virtual DOM, Hyperscript, and morehast-util-from-dom
— Transform a DOM tree to hasthast-util-select
— querySelector
, querySelectorAll
, and matches
hast-util-to-html
— Stringify nodes to HTMLhast-util-to-dom
— Transform to a DOM treeSee contributing.md
in syntax-tree/.github
for ways to get
started.
See support.md
for ways to get help.
This project has a Code of Conduct. By interacting with this repository, organisation, or community you agree to abide by its terms.
FAQs
hast utility to create trees
The npm package hastscript receives a total of 5,155,678 weekly downloads. As such, hastscript popularity was classified as popular.
We found that hastscript demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.