
What
Brief
This is a standalone Heap data structure from the data-structure-typed collection. If you wish to access more data
structures or advanced features, you can transition to directly installing the
complete data-structure-typed package
How
install
npm
npm i heap-typed --save
yarn
yarn add heap-typed
methods
Min Heap
Max Heap
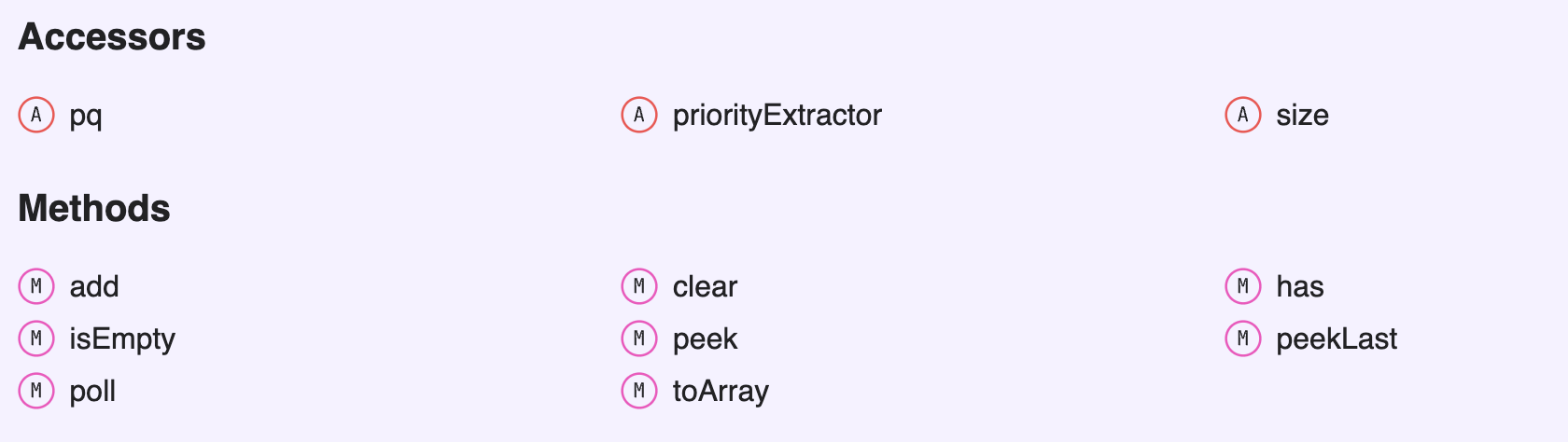
snippet
TS
import {MinHeap, MaxHeap} from 'data-structure-typed';
const minNumHeap = new MinHeap<number>();
minNumHeap.add(1).add(6).add(2).add(0).add(5).add(9);
minNumHeap.has(1)
minNumHeap.has(2)
minNumHeap.poll()
minNumHeap.poll()
minNumHeap.peek()
minNumHeap.has(1);
minNumHeap.has(2);
const arrFromHeap = minNumHeap.toArray();
arrFromHeap.length
arrFromHeap[0]
arrFromHeap[1]
arrFromHeap[2]
arrFromHeap[3]
minNumHeap.sort()
const maxHeap = new MaxHeap<{ keyA: string }>();
const myObj1 = {keyA: 'a1'}, myObj6 = {keyA: 'a6'}, myObj5 = {keyA: 'a5'}, myObj2 = {keyA: 'a2'},
myObj0 = {keyA: 'a0'}, myObj9 = {keyA: 'a9'};
maxHeap.add(1, myObj1);
maxHeap.has(myObj1)
maxHeap.has(myObj9)
maxHeap.add(6, myObj6);
maxHeap.has(myObj6)
maxHeap.add(5, myObj5);
maxHeap.has(myObj5)
maxHeap.add(2, myObj2);
maxHeap.has(myObj2)
maxHeap.has(myObj6)
maxHeap.add(0, myObj0);
maxHeap.has(myObj0)
maxHeap.has(myObj9)
maxHeap.add(9, myObj9);
maxHeap.has(myObj9)
const peek9 = maxHeap.peek(true);
peek9 && peek9.val && peek9.val.keyA
const heapToArr = maxHeap.toArray(true);
heapToArr.map(item => item?.val?.keyA)
const values = ['a9', 'a6', 'a5', 'a2', 'a1', 'a0'];
let i = 0;
while (maxHeap.size > 0) {
const polled = maxHeap.poll(true);
polled && polled.val && polled.val.keyA
i++;
}
JS
const {MinHeap, MaxHeap} = require('data-structure-typed');
const minNumHeap = new MinHeap();
minNumHeap.add(1).add(6).add(2).add(0).add(5).add(9);
minNumHeap.has(1)
minNumHeap.has(2)
minNumHeap.poll()
minNumHeap.poll()
minNumHeap.peek()
minNumHeap.has(1);
minNumHeap.has(2);
const arrFromHeap = minNumHeap.toArray();
arrFromHeap.length
arrFromHeap[0]
arrFromHeap[1]
arrFromHeap[2]
arrFromHeap[3]
minNumHeap.sort()
const maxHeap = new MaxHeap();
const myObj1 = {keyA: 'a1'}, myObj6 = {keyA: 'a6'}, myObj5 = {keyA: 'a5'}, myObj2 = {keyA: 'a2'},
myObj0 = {keyA: 'a0'}, myObj9 = {keyA: 'a9'};
maxHeap.add(1, myObj1);
maxHeap.has(myObj1)
maxHeap.has(myObj9)
maxHeap.add(6, myObj6);
maxHeap.has(myObj6)
maxHeap.add(5, myObj5);
maxHeap.has(myObj5)
maxHeap.add(2, myObj2);
maxHeap.has(myObj2)
maxHeap.has(myObj6)
maxHeap.add(0, myObj0);
maxHeap.has(myObj0)
maxHeap.has(myObj9)
maxHeap.add(9, myObj9);
maxHeap.has(myObj9)
const peek9 = maxHeap.peek(true);
peek9 && peek9.val && peek9.val.keyA
const heapToArr = maxHeap.toArray(true);
heapToArr.map(item => item?.val?.keyA)
const values = ['a9', 'a6', 'a5', 'a2', 'a1', 'a0'];
let i = 0;
while (maxHeap.size > 0) {
const polled = maxHeap.poll(true);
polled && polled.val && polled.val.keyA
i++;
}
API docs & Examples
API Docs
Live Examples
Examples Repository
Data Structures
Standard library data structure comparison
Data Structure Typed | C++ STL | java.util | Python collections |
---|
Heap<E> | priority_queue<T> | PriorityQueue<E> | heapq |
Deque<E> | deque<T> | ArrayDeque<E> | deque |
Queue<E> | queue<T> | Queue<E> | - |
HashMap<K, V> | unordered_map<K, V> | HashMap<K, V> | defaultdict |
DoublyLinkedList<E> | list<T> | LinkedList<E> | - |
SinglyLinkedList<E> | - | - | - |
BinaryTree<K, V> | - | - | - |
BST<K, V> | - | - | - |
RedBlackTree<E> | set<T> | TreeSet<E> | - |
RedBlackTree<K, V> | map<K, V> | TreeMap<K, V> | - |
TreeMultimap<K, V> | multimap<K, V> | - | - |
- | multiset<T> | - | - |
Trie | - | - | - |
DirectedGraph<V, E> | - | - | - |
UndirectedGraph<V, E> | - | - | - |
PriorityQueue<E> | priority_queue<T> | PriorityQueue<E> | - |
Array<E> | vector<T> | ArrayList<E> | list |
Stack<E> | stack<T> | Stack<E> | - |
Set<E> | - | HashSet<E> | set |
Map<K, V> | - | HashMap<K, V> | dict |
- | unordered_set<T> | HashSet<E> | - |
Map<K, V> | - | - | OrderedDict |
- | unordered_multiset | - | Counter |
- | - | LinkedHashSet<E> | - |
HashMap<K, V> | - | LinkedHashMap<K, V> | - |
- | unordered_multimap<K, V> | - | - |
- | bitset<N> | - | - |
Benchmark
avl-tree
test name | time taken (ms) | executions per sec | sample deviation |
---|
10,000 add randomly | 31.32 | 31.93 | 3.67e-4 |
10,000 add & delete randomly | 70.90 | 14.10 | 0.00 |
10,000 addMany | 40.58 | 24.64 | 4.87e-4 |
10,000 get | 27.31 | 36.62 | 2.00e-4 |
binary-tree
test name | time taken (ms) | executions per sec | sample deviation |
---|
1,000 add randomly | 12.35 | 80.99 | 7.17e-5 |
1,000 add & delete randomly | 15.98 | 62.58 | 7.98e-4 |
1,000 addMany | 10.96 | 91.27 | 0.00 |
1,000 get | 18.61 | 53.73 | 0.00 |
1,000 dfs | 164.20 | 6.09 | 0.04 |
1,000 bfs | 58.84 | 17.00 | 0.01 |
1,000 morris | 256.66 | 3.90 | 7.70e-4 |
bst
test name | time taken (ms) | executions per sec | sample deviation |
---|
10,000 add randomly | 31.59 | 31.66 | 2.74e-4 |
10,000 add & delete randomly | 74.56 | 13.41 | 8.32e-4 |
10,000 addMany | 29.16 | 34.30 | 0.00 |
10,000 get | 29.24 | 34.21 | 0.00 |
rb-tree
test name | time taken (ms) | executions per sec | sample deviation |
---|
100,000 add | 85.85 | 11.65 | 0.00 |
100,000 add & delete randomly | 211.54 | 4.73 | 0.00 |
100,000 getNode | 37.92 | 26.37 | 1.65e-4 |
comparison
test name | time taken (ms) | executions per sec | sample deviation |
---|
SRC PQ 10,000 add | 0.57 | 1748.73 | 4.96e-6 |
CJS PQ 10,000 add | 0.57 | 1746.69 | 4.91e-6 |
MJS PQ 10,000 add | 0.57 | 1749.68 | 4.43e-6 |
SRC PQ 10,000 add & pop | 3.47 | 288.14 | 6.38e-4 |
CJS PQ 10,000 add & pop | 3.39 | 295.36 | 3.90e-5 |
MJS PQ 10,000 add & pop | 3.37 | 297.17 | 3.03e-5 |
directed-graph
test name | time taken (ms) | executions per sec | sample deviation |
---|
1,000 addVertex | 0.10 | 9534.93 | 8.72e-7 |
1,000 addEdge | 6.30 | 158.67 | 0.00 |
1,000 getVertex | 0.05 | 2.16e+4 | 3.03e-7 |
1,000 getEdge | 22.31 | 44.82 | 0.00 |
tarjan | 210.90 | 4.74 | 0.01 |
tarjan all | 214.72 | 4.66 | 0.01 |
topologicalSort | 172.52 | 5.80 | 0.00 |
hash-map
test name | time taken (ms) | executions per sec | sample deviation |
---|
1,000,000 set | 275.88 | 3.62 | 0.12 |
1,000,000 Map set | 211.66 | 4.72 | 0.01 |
1,000,000 Set add | 177.72 | 5.63 | 0.02 |
1,000,000 set & get | 317.60 | 3.15 | 0.02 |
1,000,000 Map set & get | 274.99 | 3.64 | 0.03 |
1,000,000 Set add & has | 172.23 | 5.81 | 0.02 |
1,000,000 ObjKey set & get | 929.40 | 1.08 | 0.07 |
1,000,000 Map ObjKey set & get | 310.02 | 3.23 | 0.05 |
1,000,000 Set ObjKey add & has | 283.28 | 3.53 | 0.04 |
heap
test name | time taken (ms) | executions per sec | sample deviation |
---|
10,000 add & pop | 5.80 | 172.35 | 8.78e-5 |
10,000 fib add & pop | 357.92 | 2.79 | 0.00 |
doubly-linked-list
test name | time taken (ms) | executions per sec | sample deviation |
---|
1,000,000 push | 221.57 | 4.51 | 0.03 |
1,000,000 unshift | 229.02 | 4.37 | 0.07 |
1,000,000 unshift & shift | 169.21 | 5.91 | 0.02 |
1,000,000 insertBefore | 314.48 | 3.18 | 0.07 |
singly-linked-list
test name | time taken (ms) | executions per sec | sample deviation |
---|
10,000 push & pop | 212.98 | 4.70 | 0.01 |
10,000 insertBefore | 250.68 | 3.99 | 0.01 |
max-priority-queue
test name | time taken (ms) | executions per sec | sample deviation |
---|
10,000 refill & poll | 8.91 | 112.29 | 2.26e-4 |
priority-queue
test name | time taken (ms) | executions per sec | sample deviation |
---|
100,000 add & pop | 103.59 | 9.65 | 0.00 |
deque
test name | time taken (ms) | executions per sec | sample deviation |
---|
1,000,000 push | 14.55 | 68.72 | 6.91e-4 |
1,000,000 push & pop | 23.40 | 42.73 | 5.94e-4 |
1,000,000 push & shift | 24.41 | 40.97 | 1.45e-4 |
1,000,000 unshift & shift | 22.56 | 44.32 | 1.30e-4 |
queue
test name | time taken (ms) | executions per sec | sample deviation |
---|
1,000,000 push | 39.90 | 25.07 | 0.01 |
1,000,000 push & shift | 81.79 | 12.23 | 0.00 |
stack
test name | time taken (ms) | executions per sec | sample deviation |
---|
1,000,000 push | 37.60 | 26.60 | 0.00 |
1,000,000 push & pop | 47.01 | 21.27 | 0.00 |
trie
test name | time taken (ms) | executions per sec | sample deviation |
---|
100,000 push | 45.97 | 21.76 | 0.00 |
100,000 getWords | 66.20 | 15.11 | 0.00 |
Built-in classic algorithms
Algorithm | Function Description | Iteration Type |
---|
Binary Tree DFS | Traverse a binary tree in a depth-first manner, starting from the root node, first visiting the left subtree,
and then the right subtree, using recursion.
| Recursion + Iteration |
Binary Tree BFS | Traverse a binary tree in a breadth-first manner, starting from the root node, visiting nodes level by level
from left to right.
| Iteration |
Graph DFS | Traverse a graph in a depth-first manner, starting from a given node, exploring along one path as deeply as
possible, and backtracking to explore other paths. Used for finding connected components, paths, etc.
| Recursion + Iteration |
Binary Tree Morris | Morris traversal is an in-order traversal algorithm for binary trees with O(1) space complexity. It allows tree
traversal without additional stack or recursion.
| Iteration |
Graph BFS | Traverse a graph in a breadth-first manner, starting from a given node, first visiting nodes directly connected
to the starting node, and then expanding level by level. Used for finding shortest paths, etc.
| Recursion + Iteration |
Graph Tarjan's Algorithm | Find strongly connected components in a graph, typically implemented using depth-first search. | Recursion |
Graph Bellman-Ford Algorithm | Finding the shortest paths from a single source, can handle negative weight edges | Iteration |
Graph Dijkstra's Algorithm | Finding the shortest paths from a single source, cannot handle negative weight edges | Iteration |
Graph Floyd-Warshall Algorithm | Finding the shortest paths between all pairs of nodes | Iteration |
Graph getCycles | Find all cycles in a graph or detect the presence of cycles. | Recursion |
Graph getCutVertexes | Find cut vertices in a graph, which are nodes that, when removed, increase the number of connected components in
the graph.
| Recursion |
Graph getSCCs | Find strongly connected components in a graph, which are subgraphs where any two nodes can reach each other.
| Recursion |
Graph getBridges | Find bridges in a graph, which are edges that, when removed, increase the number of connected components in the
graph.
| Recursion |
Graph topologicalSort | Perform topological sorting on a directed acyclic graph (DAG) to find a linear order of nodes such that all
directed edges go from earlier nodes to later nodes.
| Recursion |
Software Engineering Design Standards
Principle | Description |
---|
Practicality | Follows ES6 and ESNext standards, offering unified and considerate optional parameters, and simplifies method names. |
Extensibility | Adheres to OOP (Object-Oriented Programming) principles, allowing inheritance for all data structures. |
Modularization | Includes data structure modularization and independent NPM packages. |
Efficiency | All methods provide time and space complexity, comparable to native JS performance. |
Maintainability | Follows open-source community development standards, complete documentation, continuous integration, and adheres to TDD (Test-Driven Development) patterns. |
Testability | Automated and customized unit testing, performance testing, and integration testing. |
Portability | Plans for porting to Java, Python, and C++, currently achieved to 80%. |
Reusability | Fully decoupled, minimized side effects, and adheres to OOP. |
Security | Carefully designed security for member variables and methods. Read-write separation. Data structure software does not need to consider other security aspects. |
Scalability | Data structure software does not involve load issues. |