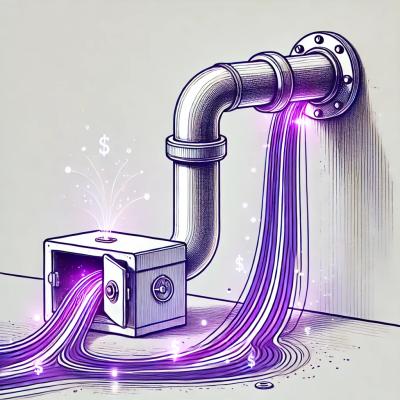
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
hedracms-sdk
Advanced tools
The **HedraCMS SDK** enables seamless integration of HedraCMS content into your frontend applications. With support for retrieving content, attributes, and paginated data, this SDK provides developers with the tools to efficiently manage CMS-driven data a
The HedraCMS SDK enables seamless integration of HedraCMS content into your frontend applications. With support for retrieving content, attributes, and paginated data, this SDK provides developers with the tools to efficiently manage CMS-driven data across multiple frameworks.
Install the SDK using npm or yarn:
npm install hedracms-sdk
# or
yarn add hedracms-sdk
Before using the SDK, initialize it in your application. The initialization ensures the SDK is ready with your account credentials and API configuration.
To initialize the SDK, use the initialize
method:
import { initialize } from 'hedracms-sdk';
initialize({
accountId: 'YOUR_ACCOUNT_ID',
accessToken: 'YOUR_ACCESS_TOKEN',
baseUrl: 'https://api.hedracms.com/api/v1',
});
accountId
: Your unique HedraCMS account identifier.accessToken
: The authentication token for API access.baseUrl
: The base URL of the HedraCMS API.import { initialize } from 'hedracms-sdk';
initialize({
accountId: '12345',
accessToken: 'abcdef12345',
baseUrl: 'https://api.hedracms.com/api/v1',
});
Use the getContent
method to retrieve single or multiple pages. The keys in the response directly match the keys you provide in the request.
import { getContent } from 'hedracms-sdk';
const content = await getContent(['homepage', 'aboutpage']);
console.log(content.homepage, content.aboutpage);
pageIds
: An array of page IDs to retrieve.batch
: Boolean. When true, sends a batch request to fetch all pages in one call.language
: String. Specify the language to retrieve localized content.const pages = await getContent(['menucontent', 'footer'], { batch: true, language: 'en' });
console.log(pages.menucontent, pages.footer);
The getAttribute
method fetches custom key-value pairs from your CMS.
import { getAttribute } from 'hedracms-sdk';
const attributes = await getAttribute(['siteSettings', 'seoConfig']);
console.log(attributes.siteSettings, attributes.seoConfig);
attributeKeys
: An array of attribute keys to retrieve.batch
: Boolean. When true, sends a batch request to fetch all attributes in one call.const siteAttributes = await getAttribute(['branding', 'siteMeta'], { batch: true });
console.log(siteAttributes.branding, siteAttributes.siteMeta);
Use the getContentList
method to retrieve paginated data for multi-page content such as blogs or products.
import { getContentList } from 'hedracms-sdk';
const paginatedData = await getContentList('blogpage', { page: 1, limit: 10 });
console.log(paginatedData.data, paginatedData.pagination);
contentType
: The type of content to retrieve (e.g., blogpage
).page
: Number. The page number to retrieve.limit
: Number. The number of items per page.language
: String. Specify the language for localized content.data
: An array of items for the current page.pagination
: Metadata including total count, total pages, and current page.interface BlogPost {
id: string;
title: string;
}
const blogPosts = await getContentList<BlogPost>('blogpage', { page: 2, limit: 5 });
console.log(blogPosts.data, blogPosts.pagination);
'use client';
import { ReactNode, useEffect, useState } from 'react';
import { initialize, isInitialized } from 'hedracms-sdk';
export function HedraCMSProvider({ children }: { children: ReactNode }) {
const [initialized, setInitialized] = useState(false);
useEffect(() => {
if (!isInitialized()) {
try {
initialize({
accountId: process.env.NEXT_PUBLIC_ACCOUNT_ID!,
accessToken: process.env.NEXT_PUBLIC_ACCESS_TOKEN!,
baseUrl: process.env.NEXT_PUBLIC_HEDRACMS_BASE_URL!,
});
setInitialized(true);
} catch (error) {
console.error('Failed to initialize HedraCMS:', error);
}
} else {
setInitialized(true);
}
}, []);
if (!initialized) {
return null;
}
return <>{children}</>;
}
import React, { useEffect, useState } from 'react';
import { initialize, isInitialized } from 'hedracms-sdk';
function App({ children }: { children: React.ReactNode }) {
const [initialized, setInitialized] = useState(false);
useEffect(() => {
if (!isInitialized()) {
try {
initialize({
accountId: process.env.REACT_APP_ACCOUNT_ID!,
accessToken: process.env.REACT_APP_ACCESS_TOKEN!,
baseUrl: process.env.REACT_APP_HEDRACMS_BASE_URL!,
});
setInitialized(true);
} catch (error) {
console.error('Failed to initialize HedraCMS:', error);
}
} else {
setInitialized(true);
}
}, []);
if (!initialized) {
return <div>Loading...</div>;
}
return <div>{children}</div>;
}
// main.ts
import { createApp } from 'vue';
import App from './App.vue';
import { initialize } from 'hedracms-sdk';
initialize({
accountId: import.meta.env.VITE_ACCOUNT_ID!,
accessToken: import.meta.env.VITE_ACCESS_TOKEN!,
baseUrl: import.meta.env.VITE_HEDRACMS_BASE_URL!,
});
createApp(App).mount('#app');
// main.ts
import { initialize } from 'hedracms-sdk';
import { platformBrowserDynamic } from '@angular/platform-browser-dynamic';
import { AppModule } from './app/app.module';
initialize({
accountId: process.env.NG_APP_ACCOUNT_ID!,
accessToken: process.env.NG_APP_ACCESS_TOKEN!,
baseUrl: process.env.NG_APP_HEDRACMS_BASE_URL!,
});
platformBrowserDynamic().bootstrapModule(AppModule).catch((err) => console.error(err));
import { initialize } from 'hedracms-sdk';
initialize({
accountId: 'YOUR_ACCOUNT_ID',
accessToken: 'YOUR_ACCESS_TOKEN',
baseUrl: 'https://api.hedracms.com/api/v1',
});
// Fetch content
import { getContent } from 'hedracms-sdk';
const loadContent = async () => {
const [homepage] = await getContent(['homepage']);
document.getElementById('content').innerHTML = `<h1>${homepage.title}</h1>`;
};
loadContent();
All methods throw errors with detailed messages. Use try-catch
for handling errors gracefully.
import { getContent } from 'hedracms-sdk';
try {
const content = await getContent(['homepage']);
} catch (error) {
console.error('Error retrieving content:', error);
}
import { getContent, getAttribute } from 'hedracms-sdk';
const pages = await getContent(['homepage', 'contactpage'], { batch: true });
console.log(pages.homepage, pages.contactpage);
const attributes = await getAttribute(['seoConfig', 'branding']);
console.log(attributes.seoConfig, attributes.branding);
© 2024 HedraCMS. All rights reserved.
FAQs
The **HedraCMS SDK** enables seamless integration of HedraCMS content into your frontend applications. With support for retrieving content, attributes, and paginated data, this SDK provides developers with the tools to efficiently manage CMS-driven data a
We found that hedracms-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.