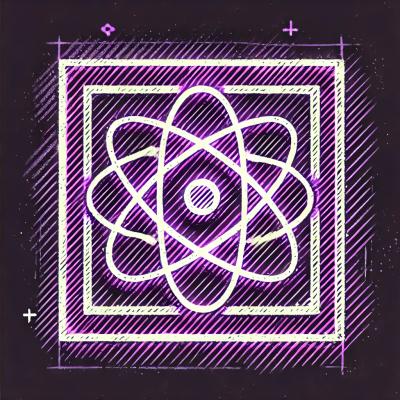
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
This is an EASY TO USE collection of useful methods for manipulating object arrays with added helpers.
Most of the examples here make use of this people object.
const people = [
{ id: 1, firstName: 'John', secondName: 'Smith', age: '61', status: 1 },
{ id: 2, firstName: 'John', secondName: 'West', age: '55', status: true },
{ id: 3, firstName: 'Brenda', secondName: 'Holt', age: '60', status: false },
{ id: 4, firstName: 'Sally', secondName: 'Brampton', age: '33', status: undefined },
{ id: 5, firstName: 'June', secondName: 'Chester', age: '47', status: NaN },
{ id: 6, firstName: 'Jack', secondName: 'Carter', age: '24', status: null },
{ id: 7, firstName: 'Jack', secondName: 'Foster', age: '58', status: 0 },
{ id: 7, firstName: 'Jack', secondName: 'Foster', age: '58', status: 0 },
{ id: 7, firstName: 'Jack', secondName: 'Foster', age: '58', status: 0 },
];
import { getEven } from 'helprjs';
function App() {
const people = [
{ id: 1, firstName: 'John', secondName: 'Smith', age: '61', status: 1 },
{ id: 2, firstName: 'John', secondName: 'West', age: '55', status: true },
{ id: 3, firstName: 'Brenda', secondName: 'Holt', age: '60', status: false },
{ id: 4, firstName: 'Sally', secondName: 'Brampton', age: '33', status: undefined },
{ id: 5, firstName: 'June', secondName: 'Chester', age: '47', status: NaN },
{ id: 6, firstName: 'Jack', secondName: 'Carter', age: '24', status: null },
{ id: 7, firstName: 'Jack', secondName: 'Foster', age: '58', status: 0 },
{ id: 7, firstName: 'Jack', secondName: 'Foster', age: '58', status: 0 },
{ id: 7, firstName: 'Jack', secondName: 'Foster', age: '58', status: 0 },
];
const result = getOdd(people,'age');
return (
<div className="App">
<h1>Users with an even age</h1>
<table>
<tr>
<th scope="col">First Name</th>
<th scope="col">Second Name</th>
<th scope="col">Age</th>
</tr>
{result && result.map((item, index) =>
<tr key={index}>
<td>{item.firstName}</td>
<td>{item.secondName}</td>
<td>{item.age}</td>
</tr>)
}
</table>
</div>
);
}
export default App;
Methods can be grouped into the following categories:
General : object array manipulation
Array: array only
Boolean : returns true or false
Helpers : deals with values
Numerical : uses numerical data
Vailidation : returns only objects that meet the valid criteria
pass in an array of values
Useage: arrayToObject(["Mike","Jones",34,true])
Returns {0: 'Mike', 1: 'Jones', 2: 34, 3: true}
pass in an array of values and custom keys
Useage: arrayToObject(["Mike","Jones",34,true], ["firstName", "lastName","age","registered"])
Returns {firstName: 'Mike', lastName: 'Jones', age: 34, registered: true}
addNewProperty(people, 'registered', true)
adds new key/value to all objects.
countKeys({a:1, b:2, c:3});
// 3
CSVtoArray(csvfile');
// [ {...} {...} ]
getEven(people,'age');
returns objects containing only even values
getObject(people,2);
returns an object from an array from array key
getOdd(people,'age');
returns objects containing only odd values
getValues(people, 'firstName');
// ["John", "John", "Brenda", "Sally", "June", "Jack", "Jack"]
groupByKey(people, 'firstName')
// {John: Array(2), Brenda: Array(1), Sally: Array(1), June: Array(1), Jack: Array(2)}
mergeArrays(arr1,arr2,key);
returns a merged array without duplicated keys
randomOrder(people);
returns randomly ordered array
const result = refine(people, "firstName", "Jack");
// return only objects that match criteria
removeDuplicates(people, 'firstName');
removes all objects containing duplicates values
removeFalsy(people, 'status');
removes all objects containing falsy values
removeValue(people,'firstName', 'John');
remove all objects that have this value
Note: 1 and '1' will give a different result, be sure to include quotation marks if targetting a string
setAllValues(people, 'age', '25');
(sets all values to 25)
sortByKey(people, 'age', 'desc');
returns object in value order
Note, 3rd paramenter is optional. desc = decending order.
toArray(people, 'age');
// ["61","55","60","33","47","24","58"]
toLower(people, 'firstName');
returns object with values as lowercase
toUpper(people, 'firstName');
returns object with values as uppercase
toString(people, 'id');
returns object with values as a string
toTruncate(people, 'firstName', 3);
returns object with values truncated to numerical value
toNumber(people, 'age');
returns object with values as numbers
toTrim(people, 'firstName');
returns object values with whitespace removed
populateArray(0,20,4)
// [4,8,12,16,20]
uniqueArray(["one",1,1,2,3,"two","three","four","one","two"])
// ["one", 1, 2, 3, "two", "three", "four"]
intersectArrays([0,1,2,3,4],[0,1,2,5,6])
// [0,1,2]
emptyArray(["one","two","three","four"])
// []
reverseArray([0,1,2,3,4,5,6])
// [6,5,4,3,2,1,0]
shuffleArray([0,1,2,3,4,5,6])
// [4,0,1,6,5,3,2]
pass in object array, key and function.
const isBelow = (currentValue) => currentValue < 99;
const result = isAll(people, 'age', isBelow)
console.log('result', result)
// true
pass in object array, key and function.
const age = 48;
const isBelow = (currentValue) => currentValue < age;
const result = isPresent(people, "age", isBelow);
console.log('result', result)
// true
isArray([1,2,3]);
// true
isBigint(9007199254740991n);
// true
isBoolean(true);
// true
isNaN(NaN);
// true
isNull(null);
// true
isNumber(1);
// true
isObject({x:1, y:2});
// true
isString('abc'});
// true
isSymbol(Symbol());
// true
isUndefined(undefined);
// true
percentage(partial, total)
// percentage(50, 200)
// 25
// calculate percentage of partial against total number
typeOf(value);
// typeOf(1); returns "number"
// typeOf([1,2,3]); returns "array"
// typeOf({x: 1}); returns "object"
uuid();
// Random uuid generator
// uuid(); returns random id
// e.g. 8ac16a9e-12fc-44fd-8311-952d212cbe02
randomId();
// Random ID generator
// zxrm95d6ug
getMaximum(people,'age');
// 61
getMinimum(people,'age');
// 24
getTotal(people, 'age');
returns sum total
getValidEmail( [{ id: 1, email: 'badEmailDotgmail.com' },{ id: 2, email: 'test@gmail.com' }] ,'email');
[{ email: 'test@gmail.com }]
returns only objects containing valid email addresses
getValidString(people, 'firstName', 5, 99)
//Note: (array, key, minimumLength, maximumLength)
returns only objects containing text within min and max length
In this example five methods have been used to generate a desired output.
It is overengineered and an unlikely requirement however it demonstrates
more possibilities when using helprjs.
FAQs
Helprjs is a JavaScript library that provides a whole mess of useful functional programming helpers without extending any built-in objects
The npm package helprjs receives a total of 36 weekly downloads. As such, helprjs popularity was classified as not popular.
We found that helprjs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.