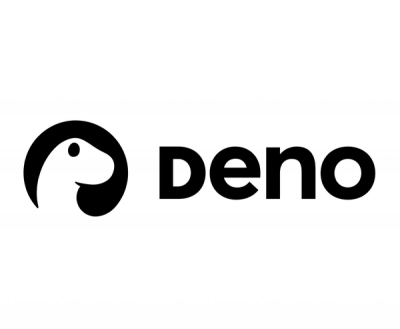
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
heroku-cli-util
Advanced tools
Set of helpful CLI utilities
npm install heroku-cli-util --save
let h = require('heroku-cli-util');
let promise = heroku.apps(appname).info();
let app = yield h.action('getting apps', promise);
console.log(`app name: ${app.name}`);
// getting apps... done
// app name: appname
Callback style
var h = require('heroku-cli-util');
h.prompt('email', {}, function (_, email) {
console.log(`your email is: ${email}`);
});
Promise style
var h = require('heroku-cli-util');
h.prompt('email', {}).then(function (email) {
console.log(`your email is: ${email}`);
});
Generator style (must be wrapped in h.command() or co block)
var h = require('heroku-cli-util');
var email = yield h.prompt('email', {});
console.log(`your email is: ${email}`);
Options
mask: true
: mask input field after submitting.
hide: true
: mask characters while entering.
Supports the same async styles as prompt()
. Errors if not confirmed.
Basic
var h = require('heroku-cli-util');
yield h.confirmApp('appname', context.flags.confirm);
// ! WARNING: Destructive Action
// ! This command will affect the app appname
// ! To proceed, type appname or re-run this command with --confirm appname
> appname
Custom message
var h = require('heroku-cli-util');
yield h.confirmApp('appname', context.flags.confirm, 'foo');
// ! foo
// ! To proceed, type appname or re-run this command with --confirm appname
> appname
var h = require('heroku-cli-util');
h.error("App not found");
// ! App not found
var h = require('heroku-cli-util');
h.warn("App not found");
// ! App not found
var h = require('heroku-cli-util');
h.columnify({
'Dynos': 1,
'Region': 'us',
'Stack': 'cedar-14'
});
// Dynos 1
// Region us
// Stack cedar-14
var h = require('heroku-cli-util');
var d = new Date();
console.log(h.formatDate(d));
// 2001-01-01T08:00:00.000Z
Used for initializing a plugin command.
give you an auth'ed instance of heroku-client
and cleanly handle API exceptions.
It uses co
so you can yield
promises.
let h = require('heroku-cli-util');
module.exports.commands = [
{
topic: 'apps',
command: 'info',
needsAuth: true,
needsApp: true,
run: h.command(function* (context, heroku) {
let app = yield heroku.apps(context.app).info();
console.dir(app);
})
}
];
With options:
let h = require('heroku-cli-util');
module.exports.commands = [
{
topic: 'apps',
command: 'info',
needsAuth: true,
needsApp: true,
run: h.command({preauth: true},
function* (context, heroku) {
let app = yield heroku.apps(context.app).info();
console.dir(app);
})
}
];
If the command has a two_factor
API error, it will ask the user for a 2fa code and retry.
If you set preauth: true
it will preauth against the current app instead of just setting the header on an app. (This is necessary if you need to do more than 1 API call that will require 2fa)
npm install
npm test
ISC
FAQs
Set of helpful CLI utilities
The npm package heroku-cli-util receives a total of 47,607 weekly downloads. As such, heroku-cli-util popularity was classified as popular.
We found that heroku-cli-util demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.