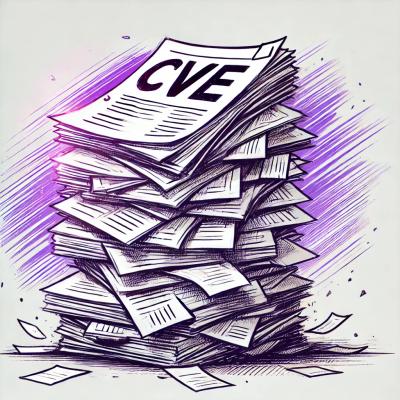
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
hkt-toolbelt
Advanced tools
A higher-kinded-type companion to ts-toolbelt.
npm install hkt-toolbelt
import { List, Conditional } from "hkt-toolbelt/list";
// Filter a tuple to only numbers
type FilterNumbers = List.Filter<Conditional.SubtypeOf<number>>;
// A :: [1, 2, 3]
type A = $<FilterNumbers, [1, null, 2, 3, "4"]>;
This library is a companion to ts-toolbelt that provides higher-kinded-type versions of its functionality. This allows for more complex types to be constructed.
The curried nature of the functions in this library is intended to be utilized to compose types using point-free style. In this respect, API types will first take in 'operations' and then the data to be operated on.
All type functions (e.g. full Kinds) take in one parameter at a time, to support currying and point-free style.
The $
operator is used to apply a higher-kinded-type function to a type. It is equivalent to the F<A>
syntax in TypeScript.
import $, { String } from "hkt-toolbelt";
type Result = $<String.Append<" world">, "hello">; // "hello world"
The $$
operator is used to apply a pipeline of kinds to a designated input type. This is a syntactic sugar for the $
and Kind.Compose
operators.
@see $
@see Kind.Compose
import { $$, Kind, String } from "hkt-toolbelt";
type Result = $$<
Kind.Compose<String.Append<" world">, String.Append<"!">>,
"hello"
>; // "hello world!"
The Cast
type is used to cast a type to another type. It is equivalent to the A as B
syntax in TypeScript. For subtle cases.
import { Cast } from "hkt-toolbelt";
type Result = Cast<"hello", string>; // "hello"
The And
type takes in a boolean and returns a function that takes in another boolean and returns the result of the two booleans being &&
'd together.
import $, { Boolean } from "hkt-toolbelt";
type Result = $<Boolean.And<true>, false>; // false
The Or
type takes in a boolean and returns a function that takes in another boolean and returns the result of the two booleans being ||
'd together.
import $, { Boolean } from "hkt-toolbelt";
type Result = $<Boolean.Or<true>, false>; // true
The Not
type takes in a boolean and returns the opposite boolean.
import $, { Boolean } from "hkt-toolbelt";
type Result = $<Boolean.Not, true>; // false
The Self
kind returns itself. This means it can be applied with $ infinitely.
import $, { Combinator } from "hkt-toolbelt";
type Result = $<$<Combinator.Self, "foo">, "foo">; // Combinator.Self
The ApplySelf
kind takes in a kind, and applies that kind to itself. This can be used to create syntho-recursive kinds.
import $, { Combinator } from "hkt-toolbelt";
type Result = $<Combinator.ApplySelf, Function.Identity>; // Function.Identity
The Equals
type is used to check if a type is equal to another type. It is equivalent to the A extends B ? (B extends A ? true : false) : false
syntax in TypeScript.
Equals
returns a higher-kinded-type function that takes a type and returns a boolean.
import $, { Conditional } from "hkt-toolbelt";
type Result = $<Conditional.Equals<"foo">, "bar">; // false
The SubtypeOf
type is used to check if a type is a subtype of another type. It is equivalent to the A extends B ? true : false
syntax in TypeScript.
The first type passed in is the supertype, and the second type passed in is the subtype.
SubtypeOf
returns a higher-kinded-type function that takes a type and returns a boolean.
import $, { Conditional } from "hkt-toolbelt";
type Result = $<Conditional.SubtypeOf<string>, "bar">; // true
The Function
type is a supertype of all functions, i.e. all functions are a subtype of Function
. It is not a kind and cannot be applied.
The Constant
type takes in a type and returns a function that takes in any type and returns the original type. It ignores its applied input and always returns the configured type.
import $, { Function } from "hkt-toolbelt";
type Result = $<Function.Constant<"foo">, number>; // "foo"
The Identity
type takes in a type and returns the same type, on the higher-kinded-type level.
import $, { Function } from "hkt-toolbelt";
type Result = $<Function.Identity, "foo">; // "foo"
The Kind
type denotes a type function that may be applied to a type using $
.
The Kind type can optionally be provided a function type to increase the specificity of its internal parameter and return types. This is used to create new kinds.
The Composable
type checks whether a tuple of kinds are composable. A tuple of kinds is composable if the output of kind $N$ is a subtype of the input of kind $N-1$.
import $, { Kind, String } from "hkt-toolbelt";
type Result = $<Kind.Composable, [String.Append<"bar">, String.Append<"foo">]>; // true
The Compose
type takes in a tuple of type functions, and composes them into one type function.
Compose
checks that the tuple of kinds is composable, and returns a higher-kinded-type function that takes in a type and returns the result of the composition.
Compose
executes functions from right to left, i.e. the last function in the tuple is executed first - as is traditional in mathematics.
import $, { Kind, String } from "hkt-toolbelt";
type Result = $<Kind.Compose<[String.Append<"bar">, String.Append<"foo">]>, "">; // "foobar"
The Pipe
type takes in a tuple of type functions, and pipes them into one type function. This operates from left to right, i.e. the first function in the tuple is executed first. This is the opposite order of Compose
.
Pipe
is often more intuitive for programmers since it reads in order of execution. This is what $$
uses internally.
import $, { Kind, String } from "hkt-toolbelt";
type Result = $<Kind.Pipe<[String.Append<"foo">, String.Append<"bar">]>, "">; // "foobar"
The _
type represents the 'unique placeholder type' used in type functions before application. Kind._
is used by $
for application.
The Map
function takes in a type function, and returns a higher kinded type that takes in a tuple type. It applies the given type function over every element in the tuple.
import $, { List, String } from "hkt-toolbelt";
type Result = $<List.Map<String.Append<"bar">>, ["foo", "baz"]>; // ["foobar", "bazbar"]
The Find
function takes in a type function, then a tuple, and returns the first tuple element for which the finder function returns true
. If no such element exists, Find
returns never
.
import $, { List, String } from "hkt-toolbelt";
type Result = $<List.Find<String.StartsWith<"foo">>, ["bar", "foobar"]>; // "foobar"
The Filter
function takes in a type function, and a tuple, and returns a tuple in-order of the input tuple, whereby only elements for which the filter function returns true
remain in the resultant tuple.
import $, { List, String } from "hkt-toolbelt";
type Result = $<List.Filter<String.StartsWith<"foo">>, ["bar", "foobar"]>; // ["foobar"]
The Append
function takes in a type, and a tuple, and applies the type such that it is appended to the end of the provided tuple.
import $, { List } from "hkt-toolbelt";
type Result = $<List.Append<"bar">, ["foo", "baz"]>; // ["foo", "baz", "bar"]
The First
function takes in a tuple, and returns the first element of the tuple.
import $, { List } from "hkt-toolbelt";
type Result = $<List.First, ["foo", "bar"]>; // "foo"
The Last
function takes in a tuple, and returns the last element of the tuple. In the case of tuples with variadic elements, the variadic element is properly handled, even if it's infix.
import $, { List } from "hkt-toolbelt";
type Result = $<List.Last, ["foo", "bar", "baz"]>; // "baz"
The Pair
function takes in a tuple, and returns a tuple of tuples, where each tuple is a pair of the original tuple's elements, in order. e.g. [1, 2, 3]
becomes [[1, 2], [2, 3]]
.
import $, { List } from "hkt-toolbelt";
type Result = $<List.Pair, [1, 2, 3]>; // [[1, 2], [2, 3]]
For variadic tuples, the variadic element is handled via introducing unions to represent the possible combinations of variadic pair elements.
The StartsWith
function takes in a string literal and returns whether or not it starts with the given prefix, returning true
or false
as appropriate.
All strings start with string
, so StartsWith<string>
will return true for all subsequent string types.
However, string
starts with no particular prefix, so $<StartsWith<"f">, string>
will result in false. All strings start with the empty string, as well.
import $, { String } from "hkt-toolbelt";
type Result = $<String.StartsWith<"foo">, "foobar">; // true
The EndsWith
function takes in a string literal and returns whether or not it ends with the given suffix, returning true
or false
as appropriate.
@see String.StartsWith
import $, { String } from "hkt-toolbelt";
type Result = $<String.EndsWith<"bar">, "foobar">; // true
The Includes
function takes in a string literal and returns whether or not it is a substring of the given string, returning true
or false
as appropriate.
@see String.StartsWith
import $, { String } from "hkt-toolbelt";
type Result = $<String.Includes<"foo">, "barfoobar">; // true
The Append
function takes in a string literal and returns a higher-kinded-type function that takes in a string and returns the result of appending the string literal to the end of the string.
import $, { String } from "hkt-toolbelt";
type Result = $<String.Append<"bar">, "foo">; // "foobar"
The Prepend
function takes in a string literal and returns a higher-kinded-type function that takes in a string and returns the result of prepending the string literal to the beginning of the string.
import $, { String } from "hkt-toolbelt";
type Result = $<String.Prepend<"foo">, "bar">; // "foobar"
[0.4.1]
FAQs
Functional and composable type utilities
The npm package hkt-toolbelt receives a total of 27 weekly downloads. As such, hkt-toolbelt popularity was classified as not popular.
We found that hkt-toolbelt demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.