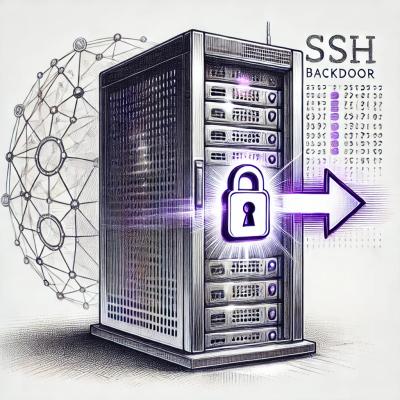
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
hoodie-plugin-store-crypto
Advanced tools
index | API | about cryptography | update | Contributing | Code of Conduct |
---|
End-to-end crypto plugin for the Hoodie client store.
This Hoodie plugin adds methods, to add, read, update and delete encrypted documents in your users store, while still being able to add, read, update and delete un-encrypted documents.
It does this by adding an object to your Hoodie-client, with similar methods to the client's store. Those methods encrypt and decrypt objects, while using the corresponding methods from Hoodie to save them.
There is no server side to this plugin!
Everything of a doc will get encrypted. Except for _id
, _rev
, _deleted
, _attachments
, _conflicts
and the hoodie
object! And all keys that start with an underscore (_) will not get encrypted!
Read more in the API Docs.
hoodie.store.add({foo: 'bar'})
.then(function (obj) { console.log(obj) })
hoodie.cryptoStore.setup('secret')
.then(async () => {
await hoodie.cryptoStore.unlock('secret')
const obj = await hoodie.cryptoStore.add({ foo: 'bar' }) // add the object encrypted
console.log(obj) // returns it un-encrypted!
const encrypted = await hoodie.store.find(obj._id) // returns the encrypted version of the object.
/*
encrypted = {
// Added by Hoodie:
_id: 'e261b431-9f8b-44d8-9835-97be550088d5',
_rev: '1-b9c5a6b9353e4dfcaf5a9183da02a647',
hoodie: {
createdAt: 'An ISO-Date'
},
// Encrypted data:
data: '09ae27028776974ef291030b85',
nonce: 'f04ad8243a5ab2f59cc4a174',
tag: '9b01f13a765ed9351d97a11bba48e7b4'
}
*/
})
This project heavily uses code and inspiration by @calvinmetcalf's crypto-pouch and Hoodie's hoodie-store-client.
Thank you to those projects and their maintainers.
There are 2 ways to use this plugin in your app:
This will add the cryptoStore to your /hoodie/client.js
if you use the hoodie
package.
First, install the plugin as dependency of your Hoodie app:
npm install --save hoodie-plugin-store-crypto
Then add it to the hoodie.plugins
array in your app’s package.json
file.
{
"name": "your-hoodie-app",
...
"hoodie": {
"plugins": [
"hoodie-plugin-store-crypto"
]
}
}
You can now start your app with npm start
. There should now be an cryptoStore
property on your client hoodie
instance. You can access it with
hoodie.cryptoStore
.
If you are using a client bundler (e.g. Browserify or Webpack), then you can import it manually.
First, install the plugin as dev-dependency of your Hoodie app:
npm install --save-dev hoodie-plugin-store-crypto
Then import it and set it up:
var Hoodie = require('@hoodie/client')
var PouchDB = require('pouchdb')
var cryptoStore = require('hoodie-plugin-store-crypto')
var hoodie = new Hoodie({ // create an instance of the hoodie-client
url: window.location.origin,
PouchDB: PouchDB
})
cryptoStore(hoodie) // sets up hoodie.cryptoStore
To use the cryptoStore you need to set a password for encryption. This can be your users password to your app, or a special password, which they will enter or you generate.
There are 5 use-cases you must put in place:
The first use of the cryptoStore. Setup can get done in your sign up function, but also if you newly added this plugin.
Use cryptoStore.setup(password, [salt])
to set the
encryption password. cryptoStore.setup(password, [salt])
will not unlock your cryptoStore instance
(just like hoodie.account.signUp)!
A salt is a second part of a password. cryptoStore.setup(password, [salt])
will save the generated salt in hoodiePluginCryptoStore/salt
, and use it. More about what the salt is.
Example:
async function signUp (username, password, cryptoPassword) {
const accountProperties = await hoodie.account.signUp({
username: username,
password: password
})
const resetKeys = await hoodie.cryptoStore.setup(cryptoPassword)
displayResetKeys(resetKeys)
return signIn(username, password, cryptoPassword) // Call your signIn function
}
Every time your user signs in you also need to unlock the cryptoStore.
Use cryptoStore.unlock(password)
for unlocking.
unlock
will try to pull hoodiePluginCryptoStore/salt
from the server. To have the latest version of it.
Example:
async function signIn (username, password, cryptoPassword) {
const accountProperties = await hoodie.account.signIn({
username: username,
password: password
})
await hoodie.cryptoStore.unlock(cryptoPassword)
// now do what you do after sign in.
}
cryptoStore
will automatically listen to account.on('signout')
events. And locks itself if it emits an event. You don't need to add any setup for it.
Use-cases for the cryptoStore.lock()
method are:
window.addEventListener('beforeunload', function (event) {
// do your cleanup
// lock the cryptoStore in an cryptographic saver way.
// It overwrites the key data 10 times.
hoodie.cryptoStore.lock()
})
This plugin doesn't save your users password! That results in you having to unlock the cryptoStore on every instance/tap of your web-app!
Example:
async function unlock (cryptoPassword) {
await hoodie.cryptoStore.unlock(cryptoPassword) // then unlock
// now do what you do after unlocking
}
You can change the password and salt used for encryption with cryptoStore.changePassword(oldPassword, newPassword)
.
This method also updates all documents, that got encrypted with the old password!
Please sync before the password change! To update all documents.
Example:
async function changePassword (oldPassword, newPassword) {
await hoodie.connectionStatus.check() // check if your app is online
if (hoodie.connectionStatus.ok) { // if your app is online: sync your users store
await hoodie.store.sync()
}
const result = await hoodie.cryptoStore.changePassword(oldPassword, newPassword)
console.log(result.notUpdated) // array of ids of all docs that weren't updated
displayResetKeys(result.resetKeys)
}
This works like changing the password. With the difference of:
The user must enter a reset-key not the old password, and calling resetPassword()
!
setup()
, changePassword()
and resetPassword()
result 10 reset-keys. You should display them to your user.
Or/and generate a text-file for your user to download.
// Generate a text file with the reset keys in it.
function generateResetKeysFile (resetKeys) {
const text = resetKeys.join('\n')
const file = new Blob([text], { type: 'text/plain' })
const url = URL.createObjectURL(file)
const a = document.getElementById('yourDownloadLink')
a.href = url
a.download = '[Your app name] reset-keys.txt' // This will be the standard file name.
// then call later URL.revokeObjectURL(url) with the url of the file.
// To remove it from memory.
}
Then, when the user did forget their encryption password, call cryptoStore.resetPassword(aResetKey, newPassword)
.
Every resetKey has a doc. Their _id
starts with hoodiePluginCryptoStore/pwReset_
, followed with the number 0 to 9. Please don't change them!
Contributions in all forms are welcome♡
Contributing might answer your questions about Contributing.
To create a welcoming project to all, this project uses a Code of Conduct. Please read it.
hoodie-plugin-store-crypto is a node.js package. You need node version 6 or higher and npm version 5 or higher. Check your installed version with node -v
and npm -v
.
git clone https://github.com/Terreii/hoodie-plugin-store-crypto.git
cd hoodie-plugin-store-crypto
npm install
Scripts for development.
Command | What it does |
---|---|
npm start | Starts a Hoodie-server with this plugin attached. |
npm test | Run all tests. |
npm run textlint | Lint the documentation. |
npm run fix:docs | Fix some lint errors in the documentation. |
npm run fix:style | Fix some code-style errors. |
npm run update-coc | Update the Code of Conduct.md. |
npm run update-contrib | Update the Contributing.md. |
FAQs
End-to-end crypto plugin for the Hoodie client store.
The npm package hoodie-plugin-store-crypto receives a total of 2 weekly downloads. As such, hoodie-plugin-store-crypto popularity was classified as not popular.
We found that hoodie-plugin-store-crypto demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.