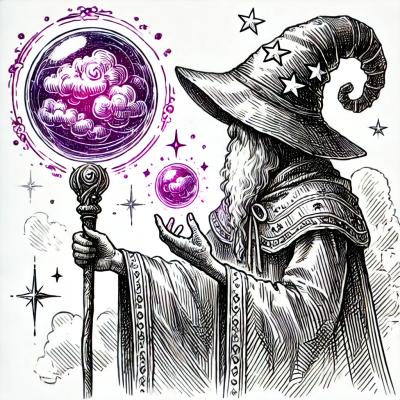
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
The hsluv npm package provides a human-friendly alternative to the HSL (Hue, Saturation, Lightness) color space. It aims to make it easier to work with colors in a way that is perceptually uniform, meaning that changes in lightness or saturation appear consistent to the human eye.
Convert HSLuv to RGB
This feature allows you to convert HSLuv color values to RGB color values. The code sample demonstrates converting an HSLuv color with hue 0, saturation 100, and lightness 50 to its RGB equivalent.
const hsluv = require('hsluv');
const rgb = hsluv.hsluvToRgb([0, 100, 50]);
console.log(rgb); // [1, 0, 0]
Convert RGB to HSLuv
This feature allows you to convert RGB color values to HSLuv color values. The code sample demonstrates converting an RGB color with values [1, 0, 0] to its HSLuv equivalent.
const hsluv = require('hsluv');
const hsluvColor = hsluv.rgbToHsluv([1, 0, 0]);
console.log(hsluvColor); // [0, 100, 50]
Convert HSLuv to Hex
This feature allows you to convert HSLuv color values to Hex color values. The code sample demonstrates converting an HSLuv color with hue 0, saturation 100, and lightness 50 to its Hex equivalent.
const hsluv = require('hsluv');
const hex = hsluv.hsluvToHex([0, 100, 50]);
console.log(hex); // '#ff0000'
Convert Hex to HSLuv
This feature allows you to convert Hex color values to HSLuv color values. The code sample demonstrates converting a Hex color '#ff0000' to its HSLuv equivalent.
const hsluv = require('hsluv');
const hsluvColor = hsluv.hexToHsluv('#ff0000');
console.log(hsluvColor); // [0, 100, 50]
Chroma.js is a JavaScript library for color conversions and color scales. It supports a wide range of color spaces including RGB, HSL, and LAB. Compared to hsluv, chroma-js offers more extensive functionality for color manipulation and generation, but it does not specifically focus on the perceptual uniformity that HSLuv provides.
The color package is a JavaScript library for color conversion and manipulation. It supports various color models such as RGB, HSL, and CMYK. While it provides a broad range of color manipulation features, it does not focus on the perceptual uniformity that HSLuv aims to achieve.
TinyColor is a small color manipulation and conversion library. It supports multiple color formats including RGB, HSL, and Hex. TinyColor is lightweight and easy to use, but it does not offer the perceptual uniformity features that HSLuv provides.
Client-side: download the latest hsluv.min.js from the releases page.
Once this module is loaded in the browser, you can access it via the
global window.hsluv
.
Server-side: npm install hsluv
.
hsluvToHex([hue, saturation, lightness])
hue is a number between 0 and 360, saturation and lightness are numbers between 0 and 100. This function returns the resulting color as a hex string.
hsluvToRgb([hue, saturation, lightness])
Like above, but returns an array of 3 numbers between 0 and 1, for the r, g, and b channel.
hexToHsluv(hex)
Takes a hex string and returns the HSLuv color as array that contains the hue (0-360), saturation(0-100) and lightness(0-100) channel. Note: The result can have rounding errors. For example saturation can be 100.00000000000007
rgbToHsluv([red, green, blue])
Like above, but red, green and blue are passed as numbers between 0 and 1.
Use hpluvToHex, hpluvToRgb, hexToHpluv and rgbToHpluv for the pastel variant (HPLuv). Note that HPLuv does not contain all the colors of RGB, so converting arbitrary RGB to it may generate invalid HPLuv colors.
FAQs
Human-friendly HSL
The npm package hsluv receives a total of 156,017 weekly downloads. As such, hsluv popularity was classified as popular.
We found that hsluv demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.